Add [title] to fillable property to allow mass assignment on [App\Models\Post]. in laravel
Answered on: Sunday 21 April, 2024 / Duration: 16 min read
Programming Language: PHP , Popularity : 4/10
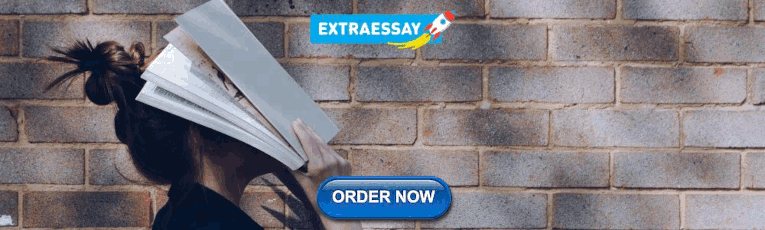
Solution 1:
To add a title field to the fillable property in the Post model in Laravel, you need to modify the $fillable array in the Post model. This will allow you to mass assign the title field when creating or updating a Post instance.
Here is an example of how you can add the title field to the fillable property in the Post model:
In this example, we added the 'title' field to the $fillable array, along with the existing 'content' and 'user_id' fields. This will allow you to mass assign the title field when creating or updating a Post instance.
To demonstrate how this works, you can create a new Post instance and mass assign the title field like this:
In this example, we are creating a new Post instance and mass assigning the 'title', 'content', and 'user_id' fields using the fill() method. The title field is included in the fillable property, so it will be accepted for mass assignment.
When you save the Post instance, the title field will be saved to the database along with the other fields. You can verify this by retrieving the Post instance and accessing the title field:
This demonstrates how you can add a title field to the fillable property in the Post model in Laravel and use mass assignment to set the title field when creating or updating Post instances.
Solution 2:
Explanation:
In Laravel, models are used to represent database tables. By default, when you mass assign data to a model, only certain attributes are allowed to be set. This is a security measure to prevent unexpected or malicious data from being inserted into your database.
To allow mass assignment on a specific model, you need to explicitly specify which attributes can be mass assigned. This can be done using the protected $fillable property.
Code Example:
In this example, we have defined the fillable property on the Post model. This means that when mass assigning data to a Post model, only the title and body attributes will be set. Any other attributes that are not included in the fillable array will be ignored.
When you mass assign data to a Post model, only the title and body attributes will be set. Any other attributes that are not included in the fillable array will be ignored.
For example, the following code will only set the title and body attributes of the $post model:
However, the following code will not set the author_id attribute of the $post model because it is not included in the fillable array:
* Security: The fillable property helps to prevent unexpected or malicious data from being inserted into your database. * Control: You can explicitly specify which attributes can be mass assigned, giving you more control over the data that is saved to your database. * Error Handling: If you try to mass assign a value to an attribute that is not included in the fillable array, Laravel will throw an exception, making it easier to identify and fix errors.
Solution 3:
In Laravel, mass assignment is the process of assigning multiple attributes to a model at once. By default, Laravel protects your models from mass assignment to prevent security vulnerabilities. To enable mass assignment for certain properties, you need to add them to the $fillable array in your model.
To add a title property to the fillable properties to allow mass assignment on the App\Models\Post model, follow these steps:
1. Open the Post model located at app/Models/Post.php .
2. Add the title property to the $fillable array if it is not already present. The $fillable array should look like this:
Here's an example of how to update the Post model:
After making these changes, you can mass-assign the title property when creating or updating a Post model. Here's an example of creating a new Post with the title property using the create method:
This creates a new Post record with the specified title property. Note that the title property should be in the $postData array when creating or updating the Post model.
Similarly, you can update an existing Post model using the update method and mass-assign the title property. Here's an example:
This updates the existing Post record with the specified title property. Again, the title property should be in the $postData array when updating the Post model.
Remember, mass-assigning properties without explicitly defining them as fillable can lead to security vulnerabilities like mass-assignment attacks. Therefore, it's recommended to define only the necessary properties in the $fillable array and avoid including sensitive properties.
More Articles :
Select in php mysql.
Answered on: Sunday 21 April, 2024 / Duration: 5-10 min read
Programming Language : PHP , Popularity : 6/10
declare variable in view for loop laravel
Programming Language : PHP , Popularity : 8/10
php csv string to associative array with column name
Without form request validation how can we use this , e: unable to locate package php7.2-mbstring.
Programming Language : PHP , Popularity : 4/10
.env example laravel
Programming Language : PHP , Popularity : 3/10
"codeigniter 4" cart
Programming Language : PHP , Popularity : 10/10
symfony command to check entities
Package phpoffice/phpexcel is abandoned, you should avoid using it. use phpoffice/phpspreadsheet instead., php time format, php remove emoji from string, "message": "call to a member function cannot() on null", "exception": "symfony\\component\\debug\\exception\\fatalthrowableerror",.
Programming Language : PHP , Popularity : 9/10
php code for if $id is not empty, do an action
Convert time to decimal php, "\u00e9" php.
Programming Language : PHP , Popularity : 7/10
->when($min_age, function ($query) use ($min_age){ $mindate = now()->subYears($min_age)->format('Y-m-d'); $query->whereHas('candidate', function ($query) use ($mindate){
Programming Language : PHP , Popularity : 5/10
laravel tinker factory
Not null laravel migration, phpmyadmin delete wordpress shortcode, php change version linux, regex for email php, php get pc cpu gpu, memory etc uses all information example, string to bool php, call php function in js, php pdo rowcount, how to get the current date in php, object of class datetime could not be converted to string, xml to object php, how does substr_compare() works php, php to save txt html.

DEV Community
Posted on Sep 7
Understanding Mass Assignment in Laravel: How fillable Protects Your Models
In Laravel, mass assignment allows you to quickly create or update records by passing an array of attributes. While convenient, it can also expose your application to security risks if not handled correctly. That’s where the fillable property comes in—it ensures that only specific fields can be mass-assigned, protecting sensitive data. This article explains how fillable works, its advantages, and why it doesn’t apply when using the save() method and how to avoid this fillable if don't need that.
What is Mass Assignment
Mass assignment refers to assigning multiple attributes to a model at once using an array. It usually happens in one of the following ways:
1. Using create():
In this example, both create() calls use mass assignment to set multiple attributes at once.
2. Using update():
Here, the update() method allows multiple fields to be updated at once through mass assignment.
Issues of Mass Assignment
Using mass assignment without proper controls can introduce serious security vulnerabilities. Consider the following example in your controller:
This is risky. Imagine request have this
Imagine they are adding an Admin and change everything. This can lead to unauthorized privilege escalation or unintended account modifications.
fillable Array and how it prevents this issues
To prevent mass assignment of sensitive fields like is_admin and status, you can specify which fields are allowed for mass assignment using the fillable array in the User model.
With the fillable array defined as shown above, only the attributes listed in the array can be mass-assigned. This means that even if a request contains fields like is_admin or status, they will be ignored when using mass assignment methods like create() or update().
Notes to consider.
insert() method not considering fillables.
insert() is a query builder method, not an Eloquent model method, so it bypasses Eloquent's features like mass assignment protection, events, and model accessors/mutators.
Ignore fillable array if needed.
If you need to bypass the fillable array for a specific operation, you can use the unguard() method provided by Laravel's Eloquent model. This method allows you to disable mass assignment protection temporarily.
How to Use unguard()
Disable Mass Assignment Protection:
- You can use Model::unguard() to disable mass assignment protection for the entire application or a specific block of code.
Enable Mass Assignment Protection Again:
- After performing the operations where mass assignment protection is not needed, you should call Model::reguard() to re-enable it.
Example Usage:
Temporarily Disable Mass Assignment Protection
Key Points:
Use with Caution : unguard() bypasses the fillable protection, so use it carefully. Ensure that you validate and sanitize the data properly before inserting it into the database.
Scope of unguard() : It affects all models in the application during its scope. If you only need to bypass fillable protection for specific models or operations, it's better to handle it at the model level or within a specific code block.
Re-enable Protection : Always call Model::reguard() after performing operations that require unguard() to ensure that mass assignment protection is re-enabled for the rest of your application.
Top comments (0)
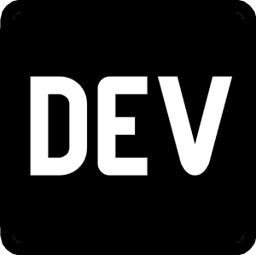
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
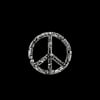
How to create an EC2 instance through Terraform
Sandeep - Sep 18

Why You Should Hide Your API Key: Best Practices for Cybersecurity
jlo128456 - Sep 18

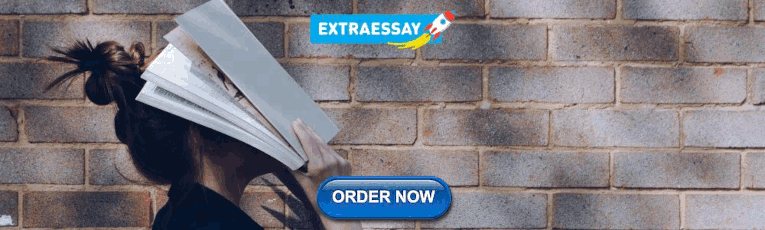
Plug-and-Play Animations: Crafting Lively Websites with asterisk/ui Components
Abhiroop Reddy - Sep 18

AR Surface Detection and Virtual Object Placement in Android Apps
Parth - Sep 18
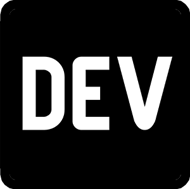
We're a place where coders share, stay up-to-date and grow their careers.
Unsupported browser
This site was designed for modern browsers and tested with Internet Explorer version 10 and later.
It may not look or work correctly on your browser.
Get Started With Laravel 8

Next lesson playing in 5 seconds
4.6 using mass assignment.
We can use mass assignment to easily create and update records in the database. It is dangerous, however, so in this lesson I'll teach you how to use mass assignment safely.
1. Introduction 2 lessons, 07:30
1.1 introduction 01:53, 1.2 set up your environment 05:37, 2. basic routing 5 lessons, 40:40, 2.1 routing requests 07:07, 2.2 working with query data 09:37, 2.3 route url parameters 07:24, 2.4 routing to controllers 08:22, 2.5 creating a view 08:10, 3. the blade templating engine 7 lessons, 45:30, 3.1 introducing layouts 08:15, 3.2 working with static resources 05:03, 3.3 generating urls for routes 03:26, 3.4 organizing views 09:41, 3.5 using blade directives 07:37, 3.6 showing and linking data 07:31, 3.7 setting up the database 03:57, 4. working with data 6 lessons, 48:45, 4.1 creating migrations and models 10:08, 4.2 saving database records 08:57, 4.3 validating user input 07:38, 4.4 updating data 07:04, 4.5 using type hints and request classes 08:50, 4.6 using mass assignment 06:08, 5. conclusion 1 lesson, 01:03, 5.1 conclusion 01:03.
We can also use mass assignment to clean up and simplify our code. There are, however, some issues that we will need to discuss. But, first, what is mass assignment? Well, whenever we have created or updated a record in the database, we have done so by assigning the columns individually. We are being very explicit as to what we are setting and then we are saving that record, that is individual assignment. Mass assignment is doing all those things, all at once. So, the code to do so would look like this. In order to create a record, we would use our modal class. It has a method called Create, and then we would just pass in the data that we needed to create that record. And that's it, we no longer have to set name, brand, year made, or saved, or anything like that. This one line of code will do all that for us. And the same is true for update. So if we look at the update method, we still need to use our record object that we have in this guitar variable, but we would call an update method. We would, once again, pass in the data that we wanted to update, and that's it. Everything else is done for us. That is mass assignment. So, the first thing about mass assignment is that the data that we are passing to either the update method or the create method needs to have keys that are the same names as the columns in the database. Like, for example, we have name in the database, but our form has guitar-name. The database has year_made and the form has just year. So, in order to use mass assignment, we need to change the field names in our forms so that they match the column names. That's not that big of a deal, so, we can go ahead and do that. Now, as you're doing this, don't forget to change the values of the four attributes for the appropriate labels. That was for the year made. Well, it's now year made, and for the name. Let's do the same thing inside of the edit view. And we also need to change the guitar form request class because we used these form field names there. And we did so, really, in two places. If we look at the rules, we need to modify those field names so that we have name and then year made. But when it comes to prepare for validation, we need to do the same thing. Now, since we just have a name property, now we can use the property syntax and then we will change year made. We also need to change the keys here to match, but that's going to make everything work. So with these changes in place, we should be able to create and update these records. So let's go ahead and let's try that. Let's go to the Create form. And let's add a Strat, because we don't have one, yet. The brand is Fender, and the year made, let's just do 2021. And whenever we submit this, we are going to get an error. And it says, add a name to fillable property to allow mass assignment. So, here's the thing about mass assignment, it's very dangerous. You are taking the request data. And even though we are validating it, which is very good, but we are just kind of blindly passing that onto the update and the create methods. Saying, here database, take this information and create or update a record. And that's really not a big deal for the data that we are working with, but imagine that you are building a system that is working with some sensitive information like user accounts. And while you are validating that information, you aren't really checking everything. Maybe the users supplied some extra fields in the request that would then be populated in the database, if there was a matching column name. There's a lot of things that can go wrong with mass mssignment. So it is something that we have to opt into. And if we look at this message, again, it says add name to fillable property. So what this means is, we go to our guitar model and we are going to add a property, it's a protected property called fillable. And this is, simply, an array that contains the columns that are okay to mass assign. In our case, that's just about every one of them. So that means name, brand, and year made. But if we left any one of these off, then eloquent will not allow that column to be assigned to value using mass assignment. We would have to do so explicitly, like we did before, by assigning a value to that column. But, as I said, the data that we are working with is okay to mass assign. There's nothing sensitive, nothing that's going to break or cause some kind of vulnerability within our application. So, now, we can go back. Let's refresh and we'll just hit Continue here, so that it will resubmit that form. And then everything should work. We should see our list of guitars, and here we can see the Strat that we added. And, of course, if we wanted to test the editing of that, all we have to do is go to the edit form for that guitar, let's add edited to the name and then we will submit. And, once again, we're going to see that that works. Because now that we sent that fillable property in our guitar model, this works for creating and updating. It's a one-time set thing. So there's nothing wrong with using Mass Assignment, just be very careful when you do so. If there are columns in your database that control how the application behaves or controls a user's access to certain parts of your application, don't make those columns fillable. It adds a little extra work on your part, but it makes your code much safer.

Fix : Add [_token] to fillable property to allow mass assignment Laravel
Mar 8, 2021
If you are working with Laravel and you encounter the following error while creating a new record in the database using Laravel Models
Add [_token] to fillable property to allow mass assignment on [App\Models\Post].
A mass assignment exception is usually caused because you didn't specify the fillable (or guarded the opposite) attributes in your model. Do this:
This way you also don't have to worry about _token because only the specified fields will be filled and saved in the db no matter what other stuff you pass to the model.
Go to list of users who liked
More than 3 years have passed since last update.
Add [ ] to fillable property to allow mass assignment onの解決方法
laravelでレコード追加機能を実装しデータベース反映まで確認済みだったのですが、諸事情によりidのカラム名を変更後、再度レコード追加しようとした際に今回のエラーが発生しました。
このエラーはデータベースにデータを保存する時に発生するエラーで、原因はEloquentで Mass Assignment の設定をしていないからのようです。
Mass Assignment (マス アサインメント) とは
Webアプリケーションのアクティブなレコードパターンが悪用され、パスワード、許可された権限、または管理者のステータスなど、ユーザーが通常はアクセスを許可すべきでないデータ項目を変更するコンピュータの脆弱性。
Web上から入力されてきた値を制限することで不正なパラメータを防ぐ仕組みになっていて、laravelでは Mass Assignment の対策がされていることから今回のエラーが発生しました。
エラーで指摘されたモデルに、下記2つの方法でどちらかを設定することで解決できます。
ホワイトリスト方式:保存したいカラム名を設定
ブラックリスト方式:書き込みを禁止したいカラム名を設定
私の場合、今回はホワイトリスト方式でレコード追加時の入力項目であるカラムを全て設定したことで解決しました!
参考記事はこちら https://qiita.com/mutturiprin/items/fd542128355f08727e45 https://nextrevolution.jp/add-to-fillable-property-to-allow-mass-assignment-on/
Go to list of comments
Register as a new user and use Qiita more conveniently
- You get articles that match your needs
- You can efficiently read back useful information
- You can use dark theme
Instantly share code, notes, and snippets.
rajucs / Add [_token] to fillable property to allow mass assignment on
- Download ZIP
- Star ( 2 ) 2 You must be signed in to star a gist
- Fork ( 0 ) 0 You must be signed in to fork a gist
- Embed Embed this gist in your website.
- Share Copy sharable link for this gist.
- Clone via HTTPS Clone using the web URL.
- Learn more about clone URLs
- Save rajucs/cea745d625be736e1ac0b19eba48f1aa to your computer and use it in GitHub Desktop.
Illuminate\Database\Eloquent\MassAssignmentException | |
Add [_token] to fillable property to allow mass assignment on [App\Model\Customer]. | |
Solution: | |
you need to fill the model | |
From: | |
namespace App\Model; | |
use Illuminate\Database\Eloquent\Model; | |
class Customer extends Model | |
{ | |
protected $table = 'customers'; | |
protected $fillable = [ | |
]; | |
} | |
To: | |
namespace App\Model; | |
use Illuminate\Database\Eloquent\Model; | |
class Customer extends Model | |
{ | |
protected $table = 'customers'; | |
protected $fillable = [ | |
'name', | |
'email', | |
'number', | |
'address', | |
'postcode', | |
'upazilla_id', | |
'district_id', | |
'division_id', | |
'country', | |
'created_by_id' | |
]; | |
} |
YercoZunigaLagos commented Mar 23, 2021
u save me <3
Sorry, something went wrong.
antoniojobs commented Mar 30, 2021
Você também me salvou S2.
WeinnySoares commented Jul 22, 2022
My solution was different, I added protected $guarded = ['id']; in the first line of the model
jesccy commented Apr 25, 2024
Same worked for me
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
MassAssignmentException in Laravel
I am a Laravel newbie. I want to seed my database. When I run the seed command I get an exception
What am I doing wrong. The command I use is:
My seed class is as follows:
11 Answers 11
Read this section of Laravel doc : http://laravel.com/docs/eloquent#mass-assignment
Laravel provides by default a protection against mass assignment security issues. That's why you have to manually define which fields could be "mass assigned" :
Warning : be careful when you allow the mass assignment of critical fields like password or role . It could lead to a security issue because users could be able to update this fields values when you don't want to.

- 7 -1 While this works, the solution from Pascalculator is better in that it un-guards only when the mass-assignment is needed and not for the lifetime of the application. – emragins Commented Oct 10, 2014 at 3:51
- 1 You are right, in the specific context of database seeding, it should be better to unguard only during the seeding. But, because this answer seems to become a reference topic about MassAssignmentException and because it think my answer is a good generic solution, I will keep it as is. – Alexandre Butynski Commented Apr 7, 2015 at 10:27
- Alexandre, I'm finding it hard to follow your logic. If you agree, you might as well change your own answer, even more so because it is becoming a reference topic. The answer you have suggested will indeed work, but isn't in compliance with the Laravel convention. – Pascalculator Commented Apr 12, 2015 at 11:41
- 4 I keep my answer as is because I think it's a good general pattern (I use it in my projects) and because I think it isn't more or less in compliance with the Laravel convention than your answer (see the doc). But, because plurality of opinions is great and because your solution can be as good as mine, I upvoted it and I encourage others to read it :) – Alexandre Butynski Commented Apr 14, 2015 at 8:52
- 'password' => bcrypt('45678') – Douglas.Sesar Commented Aug 21, 2015 at 17:16
I am using Laravel 4.2.
the error you are seeing
indeed is because the database is protected from filling en masse, which is what you are doing when you are executing a seeder. However, in my opinion, it's not necessary (and might be insecure) to declare which fields should be fillable in your model if you only need to execute a seeder.
In your seeding folder you have the DatabaseSeeder class:
This class acts as a facade, listing all the seeders that need to be executed. If you call the UsersTableSeeder seeder manually through artisan, like you did with the php artisan db:seed --class="UsersTableSeeder" command, you bypass this DatabaseSeeder class.
In this DatabaseSeeder class the command Eloquent::unguard(); allows temporary mass assignment on all tables, which is exactly what you need when you are seeding a database. This unguard method is only executed when you run the php aristan db:seed command, hence it being temporary as opposed to making the fields fillable in your model (as stated in the accepted and other answers).
All you need to do is add the $this->call('UsersTableSeeder'); to the run method in the DatabaseSeeder class and run php aristan db:seed in your CLI which by default will execute DatabaseSeeder.
Also note that you are using a plural classname Users, while Laraval uses the the singular form User. If you decide to change your class to the conventional singular form, you can simply uncomment the //$this->call('UserTableSeeder'); which has already been assigned but commented out by default in the DatabaseSeeder class.
- 4 For the paranoid, and the purists: you will also find appreciation in using \Eloquent::reguard(); , for after your Mass Assignments are complete. – CenterOrbit Commented Jun 2, 2015 at 20:53
To make all fields fillable , just declare on your class:
This will enable you to call fill method without declare each field.
- I don't want to use specific field in array. what should I do? – PramodA Commented Dec 13, 2021 at 13:40
Just add Eloquent::unguard(); in the top of the run method when you do a seed, no need to create an $fillable array in all the models you have to seed.
Normally this is already specified in the DatabaseSeeder class. However because you're calling the UsersTableSeeder directly:
php artisan db:seed --class="UsersTableSeeder"
Eloquent::unguard(); isn't being called and gives the error.

I used this and have no problem:
I was getting the MassAssignmentException when I have extends my model like this.
I was trying to insert array like this
Issue has been resolve when I created Upload Model as
Reference https://github.com/aidkit/aidkit/issues/2#issuecomment-21055670
User proper model in your controller file.

if you have table and fields on database you can simply use this command :

This is not a good way when you want to seeding database. Use faker instead of hard coding, and before all this maybe it's better to truncate tables.
Consider this example :

Use the fillable to tell laravel which fields can be filled using an array. By default, Laravel does not allow database fields to be updated via an array
The opposite of fillable is guardable .

If you use the OOP method of inserting, you don't need to worry about mass-action/fillable properties:
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php laravel or ask your own question .
- The Overflow Blog
- Looking under the hood at the tech stack that powers multimodal AI
- Featured on Meta
- Join Stack Overflow’s CEO and me for the first Stack IRL Community Event in...
- User activation: Learnings and opportunities
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Establishing Chirality For a 4D Person?
- Some of them "have no hair"
- “…[it] became a ______ for me.” Why is "gift" the right answer?
- GeometricScene not working when too many polygons are given
- corresponding author not as the last author in physics or engineering
- Removing undermount sink
- What is a “bearded” oyster?
- Intra Schengen passport check non EU Wizzair 2024
- Can science or history be used to examine religions claims despite it being a naturalist enterprise that denies the existence of the supernatural?
- Is it ethical to request partial reimbursement to present something from my previous position?
- Is "Canada's nation's capital" a mistake?
- "First et al.", many authors with same surname, and IEEE citations
- If morality is real and has causal power, could science detect the moment the "moral ontology" causes a measurable effect on the physical world?
- Using dd with nvme device
- Is it possible to make sand from bones ? Would it have the same properties as regular sand?
- Closed unit disk is connected
- Number theory: Can all rational numbers >1 be expressed as a product of rational numbers >1?
- The graph of a continuous function is a topological manifold
- Why did mire/bog skis fall out of use?
- Big bang and the horizon problem
- Is there a "hard problem of aesthetics?"
- Would a material that could absorb 99.5% of light be able to protect someone from Night Vision?
- How to interpret odds ratio for variables that range from 0 to 1
- Does it ever make sense to have a one-to-one obligatory relationship in a relational database?
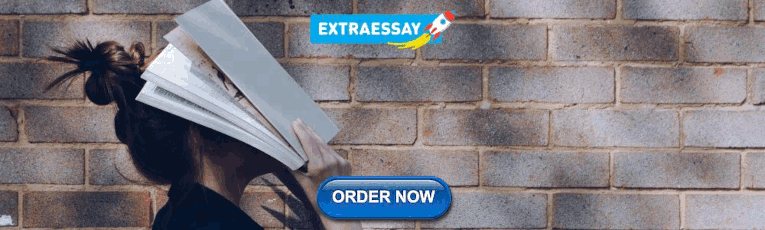
IMAGES
VIDEO
COMMENTS
However, before using the create method, you will need to specify either a fillable or guarded property on your model class. These properties are required because all Eloquent models are protected against mass assignment vulnerabilities by default.
Add [title] to fillable property to allow mass assignment on [App\Profile] Ask Question Asked 5 years, 1 month ago. Modified 1 year, 7 months ago. Viewed 6k times 2 I am trying to create edit profile, but when I click on edit profile button I'm getting below error: Illuminate \ Database \ Eloquent \ MassAssignmentException Add [title] to ...
39. Mass assignment is a process of sending an array of data that will be saved to the specified model at once. In general, you don't need to save data on your model on one by one basis, but rather in a single process. Mass assignment is good, but there are certain security problems behind it.
To allow mass assignment of these fields, you need to add them to the fillable property in your model. To fix the error, update your Game model to include _token and _method in the fillable property:
Discover in depth solution to Add [title] to fillable property to allow mass assignment on [App\\Models\\Post]. in laravel in PHP programming language. Follow our expert step-by-step guidance in PHP to improve your coding and debugging skills and efficiency.
Let's Build a SaaS in Laravel There are endless tutorials online for how to build an idealized project, based on what's easy to teach. In this course, however, we're going to walk through the real-life, actual process of building a software-as-a-service, including the mistakes and misconceptions I ran into along the way.
In Laravel, mass assignment allows you to quickly create or update records by passing an array of attributes. While convenient, it can also expose your application to security risks if not handled correctly. That's where the fillable property comes in—it ensures that only specific fields can be mass-assigned, protecting sensitive data.
And it says, add a name to fillable property to allow mass assignment. So, here's the thing about mass assignment, it's very dangerous. You are taking the request data. And even though we are validating it, which is very good, but we are just kind of blindly passing that onto the update and the create methods. Saying, here database, take this ...
Arguments "Add [title] to fillable property to allow mass assignment on [App\\Post]." Laracasts Elite. Hall of Fame. Snapey. Posted 6 years ago. Best Answer . check you have no typos in your guarded statement. Make sure you dont have a fillable variable. 0. Reply . Level 1. joffpascual OP .
Add [_token] to fillable property to allow mass assignment on [App\Models\Post]. A mass assignment exception is usually caused because you didn't specify the fillable (or guarded the opposite) attributes in your model.
Laravel Version: 5.8 PHP Version: 7.3 Description: Add [_token] to fillable property to allow mass assignment on [App\Tipo_evento]. Tipo_evento.php namespace App; use Illuminate\Database\Eloquent\Model; class Tipo_evento extends Model { ...
[100% Working] Add [title] to fillable property to allow mass assignment on [App\Models\Category].#laravelupdate #updatereocrd #updatemodel #howtoupdatemode...
Level 2. Add [title] to fillable property to allow mass assignment on [App\Post]. 'title' => 'Post Title', 'content' => 'Post Content'. "Add [title] to fillable property to allow mass assignment on [App\Post]." I searched everywhere for solution but come up with nothing so for. Any help appreciated.
Package filament/filament Package Version v2.10.12 Laravel Version v9.2.0 Livewire Version v2.10.4 PHP Version 8.1.2 Bug description Using a model (country) as a resource (CountryResource) when it extends a base class (BaseModel) results...
Add [ ] to fillable property to allow mass assignment onの解決方法. laravelでレコード追加機能を実装しデータベース反映まで確認済みだったのですが、諸事情によりidのカラム名を変更後、再度レコード追加しようとした際に今回のエラーが発生しました。. このエラーは ...
Add [sku] to fillable property to allow mass assignment on [App\\Sku]" ...
Star. Fork. Raw. Add [_token] to fillable property to allow mass assignment on. Illuminate\Database\Eloquent\MassAssignmentException. Add [_token] to fillable property to allow mass assignment on [App\Model\Customer]. Solution:
"Add [name] to fillable property to allow mass assignment on [Illuminate\Foundation\Auth\User]." 0. Add [0] to fillable property to allow mass assignment on [App\Models\posts] Hot Network Questions Cutting a curve through a thick timber without waste
[BUG] Add [id] to fillable property to allow mass assignment #4918. Closed endachao opened this issue Sep 2, 2020 · 1 comment Closed [BUG] Add [id] to fillable property to allow mass assignment #4918. endachao opened this issue Sep 2, 2020 · 1 comment Labels. wontfix. Comments. Copy link
A massive community of programmers just like you. Think of Laracasts sort of like Netflix, but for developers. You could spend weeks binging, and still not get through all the content we have to offer.
Laravel provides by default a protection against mass assignment security issues. That's why you have to manually define which fields could be "mass assigned" : ... 'email', 'password']; } Warning : be careful when you allow the mass assignment of critical fields like password or role. It could lead to a security issue because users could be ...