Async/await
There’s a special syntax to work with promises in a more comfortable fashion, called “async/await”. It’s surprisingly easy to understand and use.
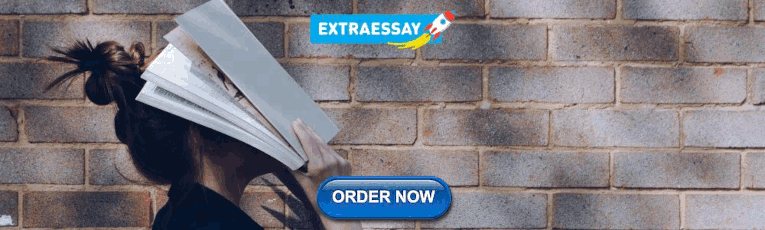
Async functions
Let’s start with the async keyword. It can be placed before a function, like this:
The word “async” before a function means one simple thing: a function always returns a promise. Other values are wrapped in a resolved promise automatically.
For instance, this function returns a resolved promise with the result of 1 ; let’s test it:
…We could explicitly return a promise, which would be the same:
So, async ensures that the function returns a promise, and wraps non-promises in it. Simple enough, right? But not only that. There’s another keyword, await , that works only inside async functions, and it’s pretty cool.
The syntax:
The keyword await makes JavaScript wait until that promise settles and returns its result.
Here’s an example with a promise that resolves in 1 second:
The function execution “pauses” at the line (*) and resumes when the promise settles, with result becoming its result. So the code above shows “done!” in one second.
Let’s emphasize: await literally suspends the function execution until the promise settles, and then resumes it with the promise result. That doesn’t cost any CPU resources, because the JavaScript engine can do other jobs in the meantime: execute other scripts, handle events, etc.
It’s just a more elegant syntax of getting the promise result than promise.then . And, it’s easier to read and write.
If we try to use await in a non-async function, there would be a syntax error:
We may get this error if we forget to put async before a function. As stated earlier, await only works inside an async function.
Let’s take the showAvatar() example from the chapter Promises chaining and rewrite it using async/await :
- We’ll need to replace .then calls with await .
- Also we should make the function async for them to work.
Pretty clean and easy to read, right? Much better than before.
In modern browsers, await on top level works just fine, when we’re inside a module. We’ll cover modules in article Modules, introduction .
For instance:
If we’re not using modules, or older browsers must be supported, there’s a universal recipe: wrapping into an anonymous async function.
Like promise.then , await allows us to use thenable objects (those with a callable then method). The idea is that a third-party object may not be a promise, but promise-compatible: if it supports .then , that’s enough to use it with await .
Here’s a demo Thenable class; the await below accepts its instances:
If await gets a non-promise object with .then , it calls that method providing the built-in functions resolve and reject as arguments (just as it does for a regular Promise executor). Then await waits until one of them is called (in the example above it happens in the line (*) ) and then proceeds with the result.
To declare an async class method, just prepend it with async :
The meaning is the same: it ensures that the returned value is a promise and enables await .
Error handling
If a promise resolves normally, then await promise returns the result. But in the case of a rejection, it throws the error, just as if there were a throw statement at that line.
…is the same as this:
In real situations, the promise may take some time before it rejects. In that case there will be a delay before await throws an error.
We can catch that error using try..catch , the same way as a regular throw :
In the case of an error, the control jumps to the catch block. We can also wrap multiple lines:
If we don’t have try..catch , then the promise generated by the call of the async function f() becomes rejected. We can append .catch to handle it:
If we forget to add .catch there, then we get an unhandled promise error (viewable in the console). We can catch such errors using a global unhandledrejection event handler as described in the chapter Error handling with promises .
When we use async/await , we rarely need .then , because await handles the waiting for us. And we can use a regular try..catch instead of .catch . That’s usually (but not always) more convenient.
But at the top level of the code, when we’re outside any async function, we’re syntactically unable to use await , so it’s a normal practice to add .then/catch to handle the final result or falling-through error, like in the line (*) of the example above.
When we need to wait for multiple promises, we can wrap them in Promise.all and then await :
In the case of an error, it propagates as usual, from the failed promise to Promise.all , and then becomes an exception that we can catch using try..catch around the call.
The async keyword before a function has two effects:
- Makes it always return a promise.
- Allows await to be used in it.
The await keyword before a promise makes JavaScript wait until that promise settles, and then:
- If it’s an error, an exception is generated — same as if throw error were called at that very place.
- Otherwise, it returns the result.
Together they provide a great framework to write asynchronous code that is easy to both read and write.
With async/await we rarely need to write promise.then/catch , but we still shouldn’t forget that they are based on promises, because sometimes (e.g. in the outermost scope) we have to use these methods. Also Promise.all is nice when we are waiting for many tasks simultaneously.
Rewrite using async/await
Rewrite this example code from the chapter Promises chaining using async/await instead of .then/catch :
The notes are below the code:
The function loadJson becomes async .
All .then inside are replaced with await .
We can return response.json() instead of awaiting for it, like this:
Then the outer code would have to await for that promise to resolve. In our case it doesn’t matter.
The error thrown from loadJson is handled by .catch . We can’t use await loadJson(…) there, because we’re not in an async function.
Rewrite "rethrow" with async/await
Below you can find the “rethrow” example. Rewrite it using async/await instead of .then/catch .
And get rid of the recursion in favour of a loop in demoGithubUser : with async/await that becomes easy to do.
There are no tricks here. Just replace .catch with try..catch inside demoGithubUser and add async/await where needed:
Call async from non-async
We have a “regular” function called f . How can you call the async function wait() and use its result inside of f ?
P.S. The task is technically very simple, but the question is quite common for developers new to async/await.
That’s the case when knowing how it works inside is helpful.
Just treat async call as promise and attach .then to it:
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
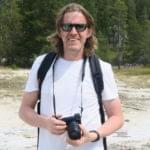
A Beginner’s Guide to JavaScript async/await, with Examples
Share this article
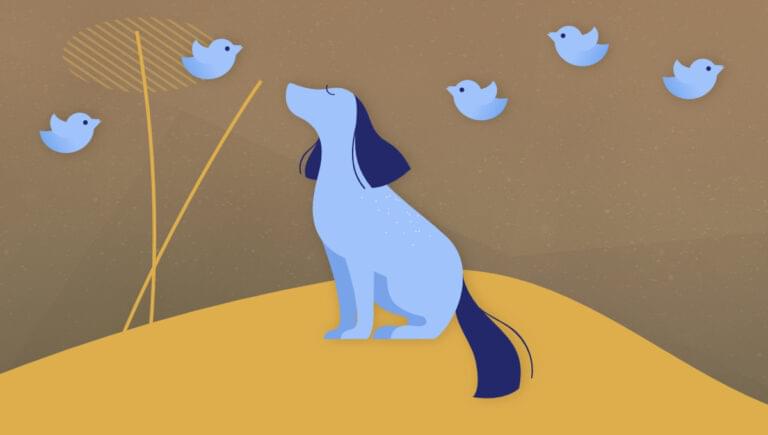
How to Create a JavaScript Async Function
Javascript await/async uses promises under the hood, error handling in async functions, running asynchronous commands in parallel, asynchronous awaits in synchronous loops, top-level await, write asynchronous code with confidence, faqs about javascript async/await.
The async and await keywords in JavaScript provide a modern syntax to help us handle asynchronous operations. In this tutorial, we’ll take an in-depth look at how to use async/await to master flow control in our JavaScript programs.
The async keyword
The await keyword, different ways of declaring async functions, switching from promises to async/await.
- Making use of catch() on the function call
In JavaScript, some operations are asynchronous . This means that the result or value they produce isn’t immediately available.
Consider the following code:
The JavaScript interpreter won’t wait for the asynchronous fetchDataFromApi function to complete before moving on to the next statement. Consequently, it logs Finished fetching data before logging the actual data returned from the API.
In many cases, this isn’t the desired behavior. Luckily, we can use the async and await keywords to make our program wait for the asynchronous operation to complete before moving on.
This functionality was introduced to JavaScript in ES2017 and is supported in all modern browsers .
For a comprehensive introduction on JavaScript and asynchronous programming, check out our free book: Learn to Code with JavaScript .
Let’s take a closer look at the data fetching logic in our fetchDataFromApi function. Data fetching in JavaScript is a prime example of an asynchronous operation.
Using the Fetch API , we could do something like this:
Here, we’re fetching a programming joke from the JokeAPI . The API’s response is in JSON format, so we extract that response once the request completes (using the json() method), then log the joke to the console.
Please note that the JokeAPI is a third-party API, so we can’t guarantee the quality of jokes that will be returned!
If we run this code in your browser, or in Node (version 17.5+ using the --experimental-fetch flag), we’ll see that things are still logged to the console in the wrong order.
Let’s change that.
The first thing we need to do is label the containing function as being asynchronous. We can do this by using the async keyword, which we place in front of the function keyword:
Asynchronous functions always return a promise (more on that later), so it would already be possible to get the correct execution order by chaining a then() onto the function call:
If we run the code now, we see something like this:
But we don’t want to do that! JavaScript’s promise syntax can get a little hairy, and this is where async/await shines: it enables us to write asynchronous code with a syntax which looks more like synchronous code and which is more readable.
The next thing to do is to put the await keyword in front of any asynchronous operations within our function. This will force the JavaScript interpreter to “pause” execution and wait for the result. We can assign the results of these operations to variables:
We also need to wait for the result of calling the fetchDataFromApi function:
Unfortunately, if we try to run the code now, we’ll encounter an error:
This is because we can’t use await outside of an async function in a non-module script. We’ll get into this in more detail later , but for now the easiest way to solve the problem is by wrapping the calling code in a function of its own, which we’ll also mark as async :
If we run the code now, everything should output in the correct order:
The fact that we need this extra boilerplate is unfortunate, but in my opinion the code is still easier to read than the promise-based version.
The previous example uses two named function declarations (the function keyword followed by the function name), but we aren’t limited to these. We can also mark function expressions, arrow functions and anonymous functions as being async .
If you’d like a refresher on the difference between function declarations and function expressions, check out our guide on when to use which .
Async function expression
A function expression is when we create a function and assign it to a variable. The function is anonymous, which means it doesn’t have a name. For example:
This would work in exactly the same way as our previous code.
Async arrow function
Arrow functions were introduced to the language in ES6. They’re a compact alternative to function expressions and are always anonymous. Their basic syntax is as follows:
To mark an arrow function as asynchronous, insert the async keyword before the opening parenthesis.
For example, an alternative to creating an additional init function in the code above would be to wrap the existing code in an IIFE , which we mark as async :
There’s not a big difference between using function expressions or function declarations: mostly it’s just a matter of preference. But there are a couple of things to be aware of, such as hoisting, or the fact that an arrow function doesn’t bind its own this value. You can check the links above for more details.
As you might have already guessed, async/await is, to a large extent, syntactic sugar for promises. Let’s look at this in a little more detail, as a better understanding of what’s happening under the hood will go a long way to understanding how async/await works.
If you’re not sure what promises are, or if you’d like a quick refresher, check out our promises guide .
The first thing to be aware of is that an async function will always return a promise, even if we don’t explicitly tell it to do so. For example:
This logs the following:
A promise can be in one of three states: pending , fulfilled , or rejected . A promise starts life in a pending state. If the action relating to the promise is successful, the promise is said to be fulfilled . If the action is unsuccessful, the promise is said to be rejected . Once a promise is either fulfilled or rejected, but not pending, it’s also considered settled .
When we use the await keyword inside of an async function to “pause” function execution, what’s really happening is that we’re waiting for a promise (either explicit or implicit) to settle into a resolved or a rejected state.
Building on our above example, we can do the following:
Because the echo function returns a promise and the await keyword inside the getValue function waits for this promise to fulfill before continuing with the program, we’re able to log the desired value to the console.
Promises are a big improvement to flow control in JavaScript and are used by several of the newer browser APIs — such as the Battery status API , the Clipboard API , the Fetch API , the MediaDevices API , and so on.
Node has also added a promisify function to its built-in util module that converts code that uses callback functions to return promises. And as of v10, functions in Node’s fs module can return promises directly.
So why does any of this matter to us?
Well, the good news is that any function that returns a promise can be used with async/await . I’m not saying that we should async/await all the things (this syntax does have its downsides, as we’ll see when we get on to error handling), but we should be aware that this is possible.
We’ve already seen how to alter our promise-based fetch call at the top of the article to work with async/await , so let’s have a look at another example. Here’s a small utility function to get the contents of a file using Node’s promise-based API and its readFile method.
Using Promise.then() :
With async/await that becomes:
Note: this is making use of a feature called top-level await , which is only available within ES modules. To run this code, save the file as index.mjs and use a version of Node >= 14.8.
Although these are simple examples, I find the async/await syntax easier to follow. This becomes especially true when dealing with multiple then() statements and with error handling thrown in to the mix. I wouldn’t go as far as converting existing promise-based code to use async/await , but if that’s something you’re interested in, VS Code can do it for you .
There are a couple of ways to handle errors when dealing with async functions. Probably the most common is to use a try...catch block, which we can wrap around asynchronous operations and catch any errors which occur.
In the following example, note how I’ve altered the URL to something that doesn’t exist:
This will result in the following message being logged to the console:
This works because fetch returns a promise. When the fetch operation fails, the promise’s reject method is called and the await keyword converts that unhanded rejection to a catchable error.
However, there are a couple of problems with this method. The main criticism is that it’s verbose and rather ugly. Imagine we were building a CRUD app and we had a separate function for each of the CRUD methods (create, read, update, destroy). If each of these methods performed an asynchronous API call, we’d have to wrap each call in its own try...catch block. That’s quite a bit of extra code.
The other problem is that, if we haven’t used the await keyword, this results in an unhandled promise rejection:
The code above logs the following:
Unlike await , the return keyword doesn’t convert promise rejections to catchable errors.
Making Use of catch() on the function call
Every function that returns a promise can make use of a promise’s catch method to handle any promise rejections which might occur.
With this simple addition, the code in the above example will handle the error gracefully:
And now this outputs the following:
As to which strategy to use, I agree with the advice of Valeri Karpov . Use try/catch to recover from expected errors inside async functions, but handle unexpected errors by adding a catch() to the calling function.
When we use the await keyword to wait for an asynchronous operation to complete, the JavaScript interpreter will accordingly pause execution. While this is handy, this might not always be what we want. Consider the following code:
Here we are making two API calls to get the number of GitHub stars for React and Vue respectively. While this works just fine, there’s no reason for us to wait for the first resolved promise before we make the second fetch request. This would be quite a bottleneck if we were making many requests.
To remedy this, we can reach for Promise.all , which takes an array of promises and waits for all promises to be resolved or for any one of them to be rejected:
Much better!
At some point, we’ll try calling an asynchronous function inside a synchronous loop. For example:
This won’t work as expected, as forEach will only invoke the function without waiting for it to complete and the following will be logged to the console:
The same thing applies to many of the other array methods, such as map , filter and reduce .
Luckily, ES2018 introduced asynchronous iterators, which are just like regular iterators except their next() method returns a promise. This means we can use await within them. Let’s rewrite the above code using one of these new iterators — for…of :
Now the process function outputs everything in the correct order:
As with our previous example of awaiting asynchronous fetch requests, this will also come at a performance cost. Each await inside the for loop will block the event loop, and the code should usually be refactored to create all the promises at once, then get access to the results using Promise.all() .
There is even an ESLint rule which complains if it detects this behavior.
Finally, let’s look at something called top-level await. This is was introduced to the language in ES2022 and has been available in Node as of v14.8.
We’ve already been bitten by the problem that this aims to solve when we ran our code at the start of the article. Remember this error?
This happens when we try to use await outside of an async function. For example, at the top level of our code:
Top-level await solves this problem, making the above code valid, but only within an ES module . If we’re working in the browser, we could add this code to a file called index.js , then load it into our page like so:
And things will work as expected — with no need for a wrapper function or the ugly IIFE.
Things get more interesting in Node. To declare a file as an ES module, we should do one of two things. One option is to save with an .mjs extension and run it like so:
The other option is to set "type": "module" in the package.json file:
Top-level await also plays nicely with dynamic imports — a function-like expression that allows us to load an ES module asynchronously. This returns a promise, and that promise resolves into a module object, meaning we can do something like this:
The dynamic imports option also lends itself well to lazy loading in combination with frameworks such as React and Vue. This enables us to reduce our initial bundle size and time to interactive metric.
In this article, we’ve looked at how you can manage the control flow of your JavaScript program using async/await . We’ve discussed the syntax, how async/await works under the hood, error handling, and a few gotchas. If you’ve made it this far, you’re now a pro. 🙂
Writing asynchronous code can be hard, especially for beginners, but now that you have a solid understanding of the techniques, you should be able to employ them to great effect.
Happy coding!
If you have any questions or comments, let me know on Twitter .
What is the main purpose of using async/await in JavaScript?
The main purpose of using async/await in JavaScript is to simplify the behavior of using promises synchronously and to perform some behavior on a group of Promises. It essentially allows you to work with Promises in a more comfortable synchronous manner. Async/await makes it easier to read (and therefore understand) asynchronous code. It makes it look like it’s synchronous, but it’s asynchronous and non-blocking behind the scenes.
Can I use async/await with any function in JavaScript?
No, you can’t use async/await with just any function. The function needs to be declared as async. This means that the function will return a Promise. If the function returns a value, the Promise will be resolved with the value, but if the function throws an exception, the Promise will be rejected with that value. You can then use await to wait for the Promise to be resolved or rejected.
What happens if I don’t handle rejections in async/await?
If a Promise is rejected and the rejection is not handled, it will result in an unhandled promise rejection. This is similar to an uncaught exception, and most JavaScript environments will report this as an error. It’s important to always handle Promise rejections when using async/await.
Can I use async/await in a loop?
Yes, you can use async/await in a loop. However, be aware that if you’re using await in a loop, each iteration will wait for the Promise to resolve before moving on to the next one. This means that if you’re not careful, your async functions could end up running in series instead of in parallel.
How can I handle errors in async/await?
You can handle errors in async/await using try/catch blocks. The try block contains the code that might throw an exception, and the catch block contains the code to handle the error. This is a similar concept to error handling in synchronous code.
Can I use Promise methods with async/await?
Yes, you can use Promise methods like .then(), .catch(), and .finally() with async/await. These methods can be useful for handling the results of async functions, especially when dealing with multiple Promises.
Can I use async/await in all browsers?
As of now, async/await is supported in most modern browsers, including Chrome, Firefox, Safari, and Edge. However, it’s not supported in Internet Explorer. If you need to support older browsers, you might need to use a transpiler like Babel to convert your async/await code into ES5 code.
Can I use async/await with callbacks?
No, async/await cannot be used with callbacks directly. Async/await is designed to work with Promises, not callbacks. However, you can convert a callback-based function into a Promise-based one, and then use async/await with that.
Can I use multiple awaits in a single async function?
Yes, you can use multiple await expressions in a single async function. Each await expression will pause the execution of the async function and wait for the Promise to resolve or reject, then resume the execution and return the resolved value.
Can I use async/await in Node.js?
Yes, you can use async/await in Node.js. As of Node.js 7.6.0, async/await is fully supported. This allows you to write asynchronous code in a more synchronous style, which can make your Node.js code easier to read and understand.
Network admin, freelance web developer and editor at SitePoint .
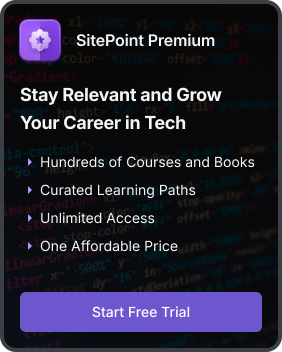
Home » JavaScript Tutorial » JavaScript async/await
JavaScript async/await
Summary : in this tutorial, you will learn how to write asynchronous code using JavaScript async / await keywords.
Note that to understand how the async / await works, you need to know how promises work.
Introduction to JavaScript async / await keywords
In the past, to handle asynchronous operations, you used the callback functions . However, nesting many callback functions can make your code more difficult to maintain, resulting in a notorious issue known as callback hell.
Suppose that you need to perform three asynchronous operations in the following sequence:
- Select a user from the database.
- Get the user’s services from an API.
- Calculate the service cost based on the services from the server.
The following functions illustrate the three tasks. Note that we use the setTimeout() function to simulate the asynchronous operation.
The following shows the nested callback functions:
To avoid this callback hell issue, ES6 introduced the promises that allow you to write asynchronous code in more manageable ways.
First, you need to return a Promise in each function:
Then, you chain the promises :
ES2017 introduced the async / await keywords that build on top of promises, allowing you to write asynchronous code that looks more like synchronous code and is more readable. Technically speaking, the async / await is syntactic sugar for promises.
If a function returns a Promise, you can place the await keyword in front of the function call, like this:
The await will wait for the Promise returned from the f() to settle. The await keyword can be used only inside the async functions.
The following defines an async function that calls the three asynchronous operations in sequence:
As you can see, the asynchronous code now looks like the synchronous code.
Let’s dive into the async / await keywords.
The async keyword
The async keyword allows you to define a function that handles asynchronous operations.
To define an async function, you place the async keyword in front of the function keyword as follows:
Asynchronous functions execute asynchronously via the event loop . It always returns a Promise .
In this example, because the sayHi() function returns a Promise , you can consume it, like this:
You can also explicitly return a Promise from the sayHi() function as shown in the following code:
The effect is the same.
Besides the regular functions, you can use the async keyword in the function expressions:
arrow functions :
and methods of classes:
The await keyword
You use the await keyword to wait for a Promise to settle either in a resolved or rejected state. You can use the await keyword only inside an async function:
In this example, the await keyword instructs the JavaScript engine to wait for the sayHi() function to complete before displaying the message.
Note that if you use the await operator outside of an async function, you will get an error.
Error handling
If a promise resolves, the await promise returns the result. However, when the promise is rejected, the await promise will throw an error as if there were a throw statement.
The following code:
… is the same as this:
In a real scenario, it will take a while for the promise to throw an error.
You can catch the error by using the try...catch statement, the same way as a regular throw statement:
It’s possible to catch errors caused by one or more await promise ‘s:
In this tutorial, you have learned how to use the JavaScript async / await keyword to write asynchronous code looks like synchronous code.

Async and Await in JavaScript
In this tutorial, we will explore more about Asynchronous programming in JavaScript by learning about Async and Await .
Understanding Async and Await
The async/await syntax is a special feature designed to simplify working with promise objects in JavaScript. It offers a cleaner and more intuitive way to write asynchronous code.
What problem does async/await solve?
The main problem with promises is the chaining of then() and catch() methods. When dealing with multiple promises, the code can become cluttered with numerous then method chains. This is where async/await comes in.
Here is an example of promise chaining:
Now let's see how we can write same code using async/await:
You can see how cleaner the code looks when using async/await.
Async Function
To use async/await in JavaScript, we need to declare an async function .
The async keyword is used to declare an async function. Inside this function, we can use the await keyword. The await keyword is used to wait for a promise to resolve or reject.
Note : The await keyword can only be used inside an async function .
Let's see an example of an async function:
Error Handling in async/await
Just like promises, we can use try/catch block to handle errors in async/await.
Let's see an example:
Async/Await with different Functions
Async/await can be used with different types of functions, like arrow functions, IIFE etc.
Benefits of Async/Await
The async/await feature offers several advantages over traditional callback or promise-based asynchronous programming:
- Readability : The code written using async/await is more readable and easier to understand, as it resembles synchronous code.
- Error Handling : Error handling becomes simpler and more straightforward, as exceptions can be caught using try/catch blocks.
- Debugging : Debugging asynchronous code becomes easier, as the flow of execution is more apparent and predictable.
JavaScript's async/await feature provides developers with a concise and intuitive way to handle asynchronous operations.
By using the async keyword and the await keyword, you can write asynchronous code that is easier to read, write, and maintain. With its simplified syntax and improved error handling, async/await has become an indispensable tool in modern JavaScript development.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
for await...of
The for await...of statement creates a loop iterating over async iterable objects as well as sync iterables . This statement can only be used in contexts where await can be used, which includes inside an async function body and in a module .
Receives a value from the sequence on each iteration. May be either a declaration with const , let , or var , or an assignment target (e.g. a previously declared variable, an object property, or a destructuring assignment pattern ). Variables declared with var are not local to the loop, i.e. they are in the same scope the for await...of loop is in.
An async iterable or sync iterable. The source of the sequence of values on which the loop operates.
A statement to be executed on every iteration. May reference variable . You can use a block statement to execute multiple statements.
Description
When a for await...of loop iterates over an iterable, it first gets the iterable's [Symbol.asyncIterator]() method and calls it, which returns an async iterator . If the @asyncIterator method does not exist, it then looks for an [Symbol.iterator]() method, which returns a sync iterator . The sync iterator returned is then wrapped into an async iterator by wrapping every object returned from the next() , return() , and throw() methods into a resolved or rejected promise, with the value property resolved if it's also a promise. The loop then repeatedly calls the final async iterator's next() method and awaits the returned promise, producing the sequence of values to be assigned to variable .
A for await...of loop exits when the iterator has completed (the awaited next() result is an object with done: true ). Like other looping statements, you can use control flow statements inside statement :
- break stops statement execution and goes to the first statement after the loop.
- continue stops statement execution and goes to the next iteration of the loop.
If the for await...of loop exited early (e.g. a break statement is encountered or an error is thrown), the return() method of the iterator is called to perform any cleanup. The returned promise is awaited before the loop exits.
for await...of generally functions the same as the for...of loop and shares many of the same syntax and semantics. There are a few differences:
- for await...of works on both sync and async iterables, while for...of only works on sync iterables.
- for await...of can only be used in contexts where await can be used, which includes inside an async function body and in a module . Even when the iterable is sync, the loop still awaits the return value for every iteration, leading to slower execution due to repeated promise unwrapping.
- If the iterable is a sync iterable that yields promises, for await...of would produce a sequence of resolved values, while for...of would produce a sequence of promises. (However, beware of error handling and cleanup — see Iterating over sync iterables and generators )
- For for await...of , the variable can be the identifier async (e.g. for await (async of foo) ); for...of forbids this case.
Iterating over async iterables
You can also iterate over an object that explicitly implements async iterable protocol:
Iterating over async generators
Since the return values of async generator functions conform to the async iterable protocol, they can be looped using for await...of .
For a more concrete example of iterating over an async generator using for await...of , consider iterating over data from an API.
This example first creates an async iterable for a stream of data, then uses it to find the size of the response from the API.
Iterating over sync iterables and generators
for await...of loop also consumes sync iterables and generators. In that case it internally awaits emitted values before assign them to the loop control variable.
Note: Be aware of yielding rejected promises from a sync generator. In such case, for await...of throws when consuming the rejected promise and DOESN'T CALL finally blocks within that generator. This can be undesirable if you need to free some allocated resources with try/finally .
To make finally blocks of a sync generator always called, use the appropriate form of the loop — for await...of for the async generator and for...of for the sync one — and await yielded promises explicitly inside the loop.
Specifications
Specification |
---|
Browser compatibility
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Symbol.asyncIterator
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Promise and Promise Chaining
Javascript setTimeout()
JavaScript try...catch...finally Statement
JavaScript async/await
We use the async keyword with a function to represent that the function is an asynchronous function. The async function returns a promise .
The syntax of async function is:
- name - name of the function
- parameters - parameters that are passed to the function
Example: Async Function
In the above program, the async keyword is used before the function to represent that the function is asynchronous.
Since this function returns a promise, you can use the chaining method then() like this:
In the above program, the f() function is resolved and the then() method gets executed.
JavaScript await Keyword
The await keyword is used inside the async function to wait for the asynchronous operation.
The syntax to use await is:
The use of await pauses the async function until the promise returns a result (resolve or reject) value. For example,
In the above program, a Promise object is created and it gets resolved after 4000 milliseconds. Here, the asyncFunc() function is written using the async function.
The await keyword waits for the promise to be complete (resolve or reject).
Hence, hello is displayed only after promise value is available to the result variable.
In the above program, if await is not used, hello is displayed before Promise resolved .
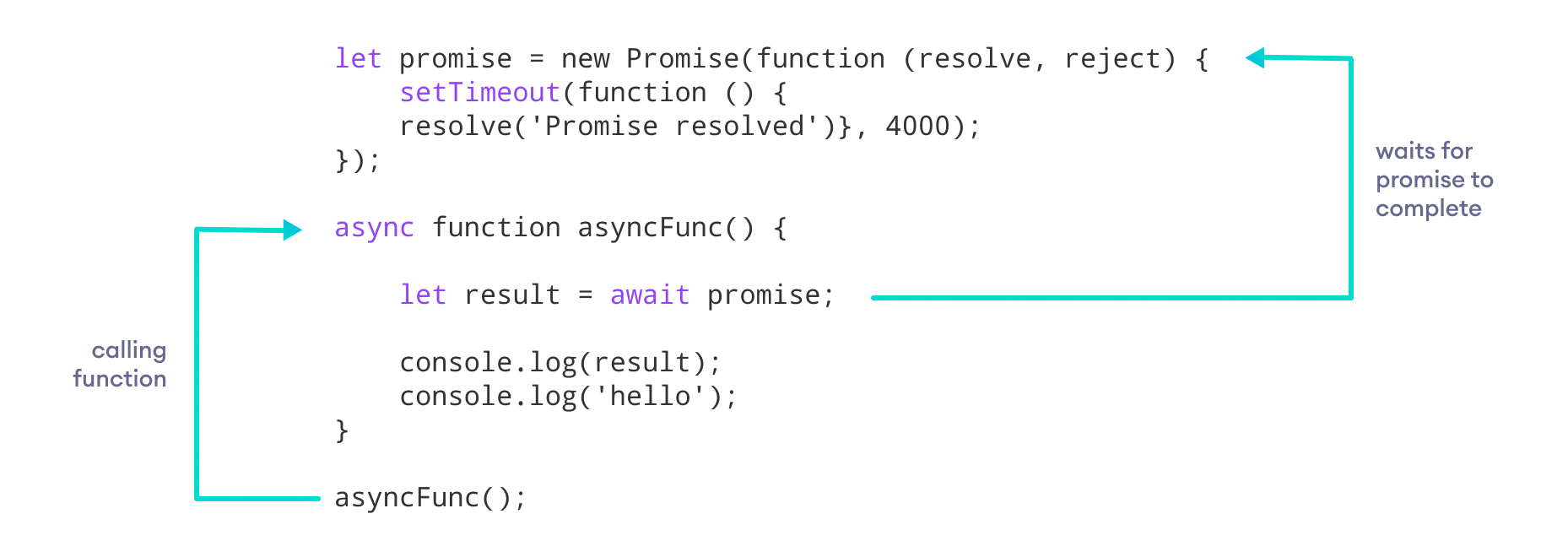
Note : You can use await only inside of async functions.
The async function allows the asynchronous method to be executed in a seemingly synchronous way. Though the operation is asynchronous, it seems that the operation is executed in synchronous manner.
This can be useful if there are multiple promises in the program. For example,
In the above program, await waits for each promise to be complete.
Error Handling
While using the async function, you write the code in a synchronous manner. And you can also use the catch() method to catch the error. For example,
The other way you can handle an error is by using try/catch block. For example,
In the above program, we have used try/catch block to handle the errors. If the program runs successfully, it will go to the try block. And if the program throws an error, it will go to the catch block.
To learn more about try/catch in detail, visit JavaScript JavaScript try/catch .
Benefits of Using async Function
- The code is more readable than using a callback or a promise.
- Error handling is simpler.
- Debugging is easier.
Note : These two keywords async/await were introduced in the newer version of JavaScript (ES8). Some older browsers may not support the use of async/await. To learn more, visit JavaScript async/await browser support .
Table of Contents
- Introduction
- Example of async Function
- The await keyword
- Error handling
- Benefits of async function
Sorry about that.
Related Tutorials
JavaScript Tutorial
Async JavaScript: From Callbacks, to Promises, to Async/Await
One of my favorite sites is BerkshireHathaway.com - it's simple, effective, and has been doing its job well since it launched in 1997. Even more remarkable, over the last 20 years, there's a good chance this site has never had a bug. Why? Because it's all static. It's been pretty much the same since it launched over 20 years ago. Turns out sites are pretty simple to build if you have all of your data up front. Unfortunately, most sites now days don't. To compensate for this, we've invented "patterns" for handling fetching external data for our apps. Like most things, these patterns each have tradeoffs that have changed over time. In this post, we'll break down the pros and cons of three of the most common patterns, Callbacks , Promises , and Async/Await and talk about their significance and progression from a historical context.
Let's start with the OG of these data fetching patterns, Callbacks.
I'm going to assume you know exactly 0 about callbacks. If I'm assuming wrong, just scroll down a bit.
When I was first learning to program, it helped me to think about functions as machines. These machines can do anything you want them to. They can even accept input and return a value. Each machine has a button on it that you can press when you want the machine to run, ().
Whether I press the button, you press the button, or someone else presses the button doesn't matter. Whenever the button is pressed, like it or not, the machine is going to run.
In the code above we assign the add function to three different variables, me , you , and someoneElse . It's important to note that the original add and each of the variables we created are pointing to the same spot in memory. They're literally the exact same thing under different names. So when we invoke me , you , or someoneElse , it's as if we're invoking add .
Now, what if we take our add machine and pass it to another machine? Remember, it doesn't matter who presses the () button, if it's pressed, it's going to run.
Your brain might have got a little weird on this one, nothing new is going on here though. Instead of "pressing the button" on add , we pass add as an argument to addFive , rename it addReference , and then we "press the button" or invoke it.
This highlights some important concepts of the JavaScript language. First, just as you can pass a string or a number as an argument to a function, so too can you pass a reference to a function as an argument. When you do this the function you're passing as an argument is called a callback function and the function you're passing the callback function to is called a higher order function .
Because vocabulary is important, here's the same code with the variables re-named to match the concepts they're demonstrating.
This pattern should look familiar, it's everywhere. If you've ever used any of the JavaScript Array methods, you've used a callback. If you've ever used lodash, you've used a callback. If you've ever used jQuery, you've used a callback.
In general, there are two popular use cases for callbacks. The first, and what we see in the .map and _.filter examples, is a nice abstraction over transforming one value into another. We say "Hey, here's an array and a function. Go ahead and get me a new value based on the function I gave you". The second, and what we see in the jQuery example, is delaying execution of a function until a particular time. "Hey, here's this function. Go ahead and invoke it whenever the element with an id of btn is clicked." It's this second use case that we're going to focus on, "delaying execution of a function until a particular time".
Right now we've only looked at examples that are synchronous. As we talked about at the beginning of this post, most of the apps we build don't have all the data they need up front. Instead, they need to fetch external data as the user interacts with the app. We've just seen how callbacks can be a great use case for this because, again, they allow you to "delay execution of a function until a particular time". It doesn't take much imagination to see how we can adapt that sentence to work with data fetching. Instead of delaying execution of a function until a particular time , we can delay execution of a function until we have the data we need . Here's probably the most popular example of this, jQuery's getJSON method.
We can't update the UI of our app until we have the user's data. So what do we do? We say, "Hey, here's an object. If the request succeeds, go ahead and call success passing it the user's data. If it doesn't, go ahead and call error passing it the error object. You don't need to worry about what each method does, just be sure to call them when you're supposed to". This is a perfect demonstration of using a callback for async requests.
At this point, we've learned about what callbacks are and how they can be beneficial both in synchronous and asynchronous code. What we haven't talked yet is the dark side of callbacks. Take a look at this code below. Can you tell what's happening?
If it helps, you can play around with the live version here .
Notice we've added a few more layers of callbacks. First, we're saying don't run the initial AJAX request until the element with an id of btn is clicked. Once the button is clicked, we make the first request. If that request succeeds, we make a second request. If that request succeeds, we invoke the updateUI method passing it the data we got from both requests. Regardless of if you understood the code at first glance or not, objectively it's much harder to read than the code before. This brings us to the topic of "Callback Hell".
As humans, we naturally think sequentially. When you have nested callbacks inside of nested callbacks, it forces you out of your natural way of thinking. Bugs happen when there's a disconnect between how your software is read and how you naturally think.
Like most solutions to software problems, a commonly prescribed approach for making "Callback Hell" easier to consume is to modularize your code.
OK, the function names help us understand what's going on, but is it objectively "better"? Not by much. We've put a band-aid over the readability issue of Callback Hell. The problem still exists that we naturally think sequentially and, even with the extra functions, nested callbacks break us out of that sequential way of thinking.
The next issue of callbacks has to do with inversion of control . When you write a callback, you're assuming that the program you're giving the callback to is responsible and will call it when (and only when) it's supposed to. You're essentially inverting the control of your program over to another program. When you're dealing with libraries like jQuery, lodash, or even vanilla JavaScript, it's safe to assume that the callback function will be invoked at the correct time with the correct arguments. However, for many third-party libraries, callback functions are the interface for how you interact with them. It's entirely plausible that a third party library could, whether on purpose or accidentally, break how they interact with your callback.
Since you're not the one calling criticalFunction , you have 0 control over when and with what argument it's invoked. Most of the time this isn't an issue, but when it is, it's a big one.
Have you ever been to a busy restaurant without a reservation? When this happens, the restaurant needs a way to get back in contact with you when a table opens up. Historically, they'd just take your name and yell it when your table was ready. Then, as naturally occurs, they decided to start getting fancy. One solution was, instead of taking your name, they'd take your number and text you once a table opened up. This allowed you to be out of yelling range but more importantly, it allowed them to target your phone with ads whenever they wanted. Sound familiar? It should! OK, maybe it shouldn't. It's a metaphor for callbacks! Giving your number to a restaurant is just like giving a callback function to a third party service. You expect the restaurant to text you when a table opens up, just like you expect the third party service to invoke your function when and how they said they would. Once your number or callback function is in their hands though, you've lost all control.
Thankfully, there is another solution that exists. One that, by design, allows you to keep all the control. You've probably even experienced it before - it's that little buzzer thing they give you. You know, this one.
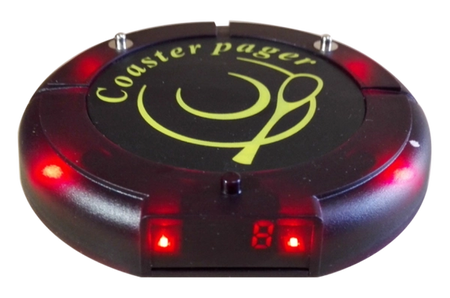
If you've never used one before, the idea is simple. Instead of taking your name or number, they give you this device. When the device starts buzzing and glowing, your table is ready. You can still do whatever you'd like as you're waiting for your table to open up, but now you don't have to give up anything. In fact, it's the exact opposite. They have to give you something. There is no inversion of control.
The buzzer will always be in one of three different states - pending , fulfilled , or rejected .
pending is the default, initial state. When they give you the buzzer, it's in this state.
fulfilled is the state the buzzer is in when it's flashing and your table is ready.
rejected is the state the buzzer is in when something goes wrong. Maybe the restaurant is about to close or they forgot someone rented out the restaurant for the night.
Again, the important thing to remember is that you, the receiver of the buzzer, have all the control. If the buzzer gets put into fulfilled , you can go to your table. If it gets put into fulfilled and you want to ignore it, cool, you can do that too. If it gets put into rejected , that sucks but you can go somewhere else to eat. If nothing ever happens and it stays in pending , you never get to eat but you're not actually out anything.
Now that you're a master of the restaurant buzzer thingy, let's apply that knowledge to something that matters.
If giving the restaurant your number is like giving them a callback function, receiving the little buzzy thing is like receiving what's called a "Promise".
As always, let's start with why . Why do Promises exist? They exist to make the complexity of making asynchronous requests more manageable. Exactly like the buzzer, a Promise can be in one of three states, pending , fulfilled or rejected . Unlike the buzzer, instead of these states representing the status of a table at a restaurant, they represent the status of an asynchronous request.
If the async request is still ongoing, the Promise will have a status of pending . If the async request was successfully completed, the Promise will change to a status of fulfilled . If the async request failed, the Promise will change to a status of rejected . The buzzer metaphor is pretty spot on, right?
Now that you understand why Promises exist and the different states they can be in, there are three more questions we need to answer.
- How do you create a Promise?
- How do you change the status of a promise?
- How do you listen for when the status of a promise changes?
1) How do you create a Promise?
This one is pretty straight forward. You create a new instance of Promise .
2) How do you change the status of a promise?
The Promise constructor function takes in a single argument, a (callback) function. This function is going to be passed two arguments, resolve and reject .
resolve - a function that allows you to change the status of the promise to fulfilled
reject - a function that allows you to change the status of the promise to rejected .
In the code below, we use setTimeout to wait 2 seconds and then invoke resolve . This will change the status of the promise to fulfilled .
We can see this change in action by logging the promise right after we create it and then again roughly 2 seconds later after resolve has been called.
Notice the promise goes from <pending> to <resolved> .
3) How do you listen for when the status of a promise changes?
In my opinion, this is the most important question. It's cool we know how to create a promise and change its status, but that's worthless if we don't know how to do anything after the status changes.
One thing we haven't talked about yet is what a promise actually is. When you create a new Promise , you're really just creating a plain old JavaScript object. This object can invoke two methods, then , and catch . Here's the key. When the status of the promise changes to fulfilled , the function that was passed to .then will get invoked. When the status of a promise changes to rejected , the function that was passed to .catch will be invoked. What this means is that once you create a promise, you'll pass the function you want to run if the async request is successful to .then . You'll pass the function you want to run if the async request fails to .catch .
Let's take a look at an example. We'll use setTimeout again to change the status of the promise to fulfilled after two seconds (2000 milliseconds).
If you run the code above you'll notice that roughly 2 seconds later, you'll see "Success!" in the console. Again the reason this happens is because of two things. First, when we created the promise, we invoked resolve after ~2000 milliseconds - this changed the status of the promise to fulfilled . Second, we passed the onSuccess function to the promises' .then method. By doing that we told the promise to invoke onSuccess when the status of the promise changed to fulfilled which it did after ~2000 milliseconds.
Now let's pretend something bad happened and we wanted to change the status of the promise to rejected . Instead of calling resolve , we would call reject .
Now this time instead of the onSuccess function being invoked, the onError function will be invoked since we called reject .
Now that you know your way around the Promise API, let's start looking at some real code.
Remember the last async callback example we saw earlier?
Is there any way we could use the Promise API here instead of using callbacks? What if we wrap our AJAX requests inside of a promise? Then we can simply resolve or reject depending on how the request goes. Let's start with getUser .
Nice. Notice that the parameters of getUser have changed. Instead of receiving id , onSuccess , and onFailure , it just receives id . There's no more need for those other two callback functions because we're no longer inverting control. Instead, we use the Promise's resolve and reject functions. resolve will be invoked if the request was successful, reject will be invoked if there was an error.
Next, let's refactor getWeather . We'll follow the same strategy here. Instead of taking in onSuccess and onFailure callback functions, we'll use resolve and reject .
Looking good. Now the last thing we need to update is our click handler. Remember, here's the flow we want to take.
- Get the user's information from the Github API.
- Use the user's location to get their weather from the Yahoo Weather API.
- Update the UI with the user's info and their weather.
Let's start with #1 - getting the user's information from the Github API.
Notice that now instead of getUser taking in two callback functions, it returns us a promise that we can call .then and .catch on. If .then is called, it'll be called with the user's information. If .catch is called, it'll be called with the error.
Next, let's do #2 - Use the user's location to get their weather.
Notice we follow the exact same pattern we did in #1 but now we invoke getWeather passing it the user object we got from userPromise .
Finally, #3 - Update the UI with the user's info and their weather.
Here's the full code you can play around with.
Our new code is better , but there are still some improvements we can make. Before we can make those improvements though, there are two more features of promises you need to be aware of, chaining and passing arguments from resolve to then .
Both .then and .catch will return a new promise. That seems like a small detail but it's important because it means that promises can be chained.
In the example below, we call getPromise which returns us a promise that will resolve in at least 2000 milliseconds. From there, because .then will return a promise, we can continue to chain our .then s together until we throw a new Error which is caught by the .catch method.
Cool, but why is this so important? Remember back in the callback section we talked about one of the downfalls of callbacks being that they force you out of your natural, sequential way of thinking. When you chain promises together, it doesn't force you out of that natural way of thinking because chained promises are sequential. getPromise runs then logA runs then logB runs then... .
Just so you can see one more example, here's a common use case when you use the fetch API. fetch will return you a promise that will resolve with the HTTP response. To get the actual JSON, you'll need to call .json . Because of chaining, we can think about this in a sequential manner.
Now that we know about chaining, let's refactor our getUser / getWeather code from earlier to use it.
It looks much better, but now we're running into an issue. Can you spot it? In the second .then we want to call updateUI . The problem is we need to pass updateUI both the user and the weather . Currently, how we have it set up, we're only receiving the weather , not the user . Somehow we need to figure out a way to make it so the promise that getWeather returns is resolved with both the user and the weather .
Here's the key. resolve is just a function. Any arguments you pass to it will be passed along to the function given to .then . What that means is that inside of getWeather , if we invoke resolve ourself, we can pass to it weather and user . Then, the second .then method in our chain will receive both user and weather as an argument.
You can play around with the final code here
It's in our click handler where you really see the power of promises shine compared to callbacks.
Following that logic feels natural because it's how we're used to thinking, sequentially. getUser then getWeather then update the UI with the data .
Now it's clear that promises drastically increase the readability of our asynchronous code, but is there a way we can make it even better? Assume that you were on the TC39 committee and you had all the power to add new features to the JavaScript language. What steps, if any, would you take to improve this code?
As we've discussed, the code reads pretty nicely. Just as our brains work, it's in a sequential order. One issue that we did run into was that we needed to thread the data ( users ) from the first async request all the way through to the last .then . This wasn't a big deal, but it made us change up our getWeather function to also pass along users . What if we just wrote our asynchronous code the same way which we write our synchronous code? If we did, that problem would go away entirely and it would still read sequentially. Here's an idea.
Well, that would be nice. Our asynchronous code looks exactly like our synchronous code. There's no extra steps our brain needs to take because we're already very familiar with this way of thinking. Sadly, this obviously won't work. As you know, if we were to run the code above, user and weather would both just be promises since that's what getUser and getWeather return. But remember, we're on TC39. We have all the power to add any feature to the language we want. As is, this code would be really tricky to make work. We'd have to somehow teach the JavaScript engine to know the difference between asynchronous function invocations and regular, synchronous function invocations on the fly. Let's add a few keywords to our code to make it easier on the engine.
First, let's add a keyword to the main function itself. This could clue the engine to the fact that inside of this function, we're going to have some asynchronous function invocations. Let's use async for this.
Cool. That seems reasonable. Next let's add another keyword to let the engine know exactly when a function being invoked is asynchronous and is going to return a promise. Let's use await . As in, "Hey engine. This function is asynchronous and returns a promise. Instead of continuing on like you typically do, go ahead and 'await' the eventual value of the promise and return it before continuing". With both of our new async and await keywords in play, our new code will look like this.
Pretty slick. We've invented a reasonable way to have our asynchronous code look and behave as if it were synchronous. Now the next step is to actually convince someone on TC39 that this is a good idea. Lucky for us, as you probably guessed by now, we don't need to do any convincing because this feature is already part of JavaScript and it's called Async/Await .
Don't believe me? Here's our live code now that we've added Async/Await to it. Feel free to play around with it.
async functions return a promise
Now that you've seen the benefit of Async/Await, let's discuss some smaller details that are important to know. First, anytime you add async to a function, that function is going to implicitly return a promise.
Even though getPromise is literally empty, it'll still return a promise since it was an async function.
If the async function returns a value, that value will also get wrapped in a promise. That means you'll have to use .then to access it.
await without async is bad
If you try to use the await keyword inside of a function that isn't async , you'll get an error.
Here's how I think about it. When you add async to a function it does two things. It makes it so the function itself returns (or wraps what gets returned in) a promise and makes it so you can use await inside of it.
Error Handling
You may have noticed we cheated a little bit. In our original code we had a way to catch any errors using .catch . When we switched to Async/Await, we removed that code. With Async/Await, the most common approach is to wrap your code in a try/catch block to be able to catch the error.
Before you leave
I know, another newsletter pitch - but hear me out. Most JavaScript newsletters are terrible. When’s the last time you actually looked forward to getting one? Even worse, when’s the last time you actually read one? We wanted to change that.
We call it Bytes , but others call it their favorite newsletter .
Delivered to 214,708 developers every Monday and Thursday
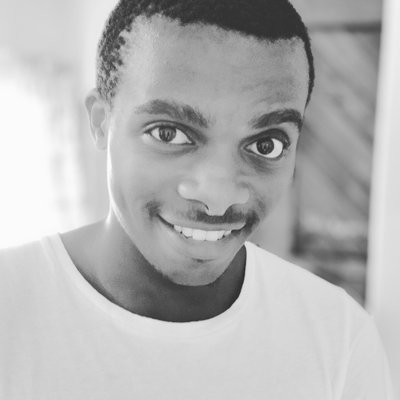
@ sduduzo_g
This is the first ever newsletter that I open a music playlist for and maximize my browser window just to read it in peace. Kudos to @uidotdev for great weekly content.
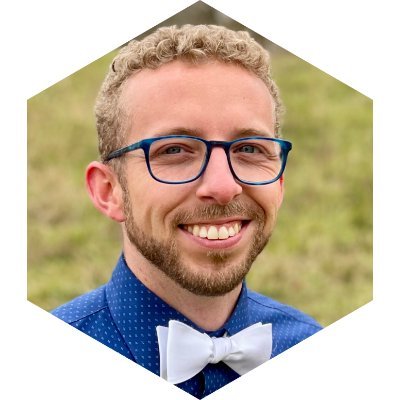
Brandon Bayer
The Bytes newsletter is a work of art! It’s the only dev newsletter I’m subscribed too. They somehow take semi boring stuff and infuse it with just the right amount of comedy to make you chuckle.
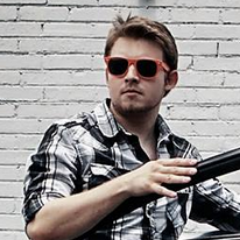
John Hawley
@ johnhawly
Bytes has been my favorite newsletter since its inception. It’s my favorite thing I look forward to on Mondays. Goes great with a hot cup of coffee!
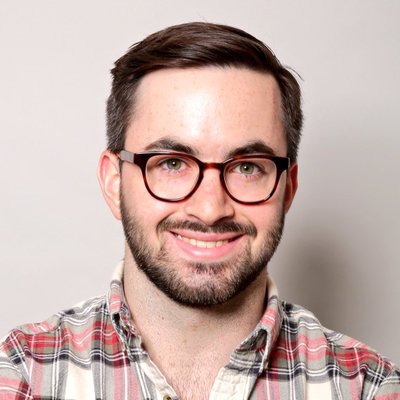
Garrett Green
@ garrettgreen
I subscribe to A LOT of dev (especially JS/TS/Node) newsletters and Bytes by @uidotdev is always such a welcomed, enjoyable change of pace to most (funny, lighthearted, etc) but still comprehensive/useful.
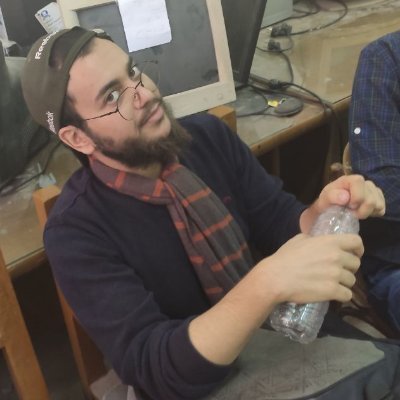
@ mhashim6_
Literally the only newsletter I’m waiting for every week.
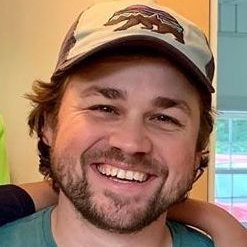
Grayson Hicks
@ graysonhicks
Bytes is the developer newsletter I most look forward to each week. Great balance of content and context! Thanks @uidotdev.
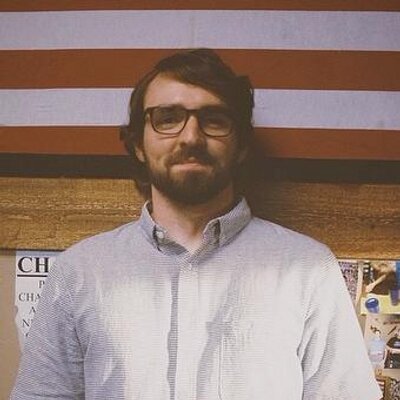
Mitchell Wright
@ mitchellbwright
I know I’ve said it before, but @tylermcginnis doesn’t miss with the Bytes email. If you’re a developer, you really need to subscribe
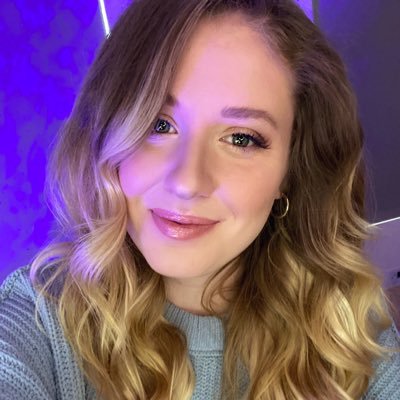
Ali Spittel
Can I just say that I giggle every time I get the @uidotdev email each week? You should definitely subscribe.
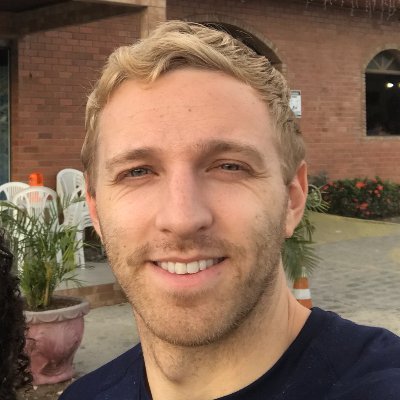
@ thefinnomenon
Every JavaScript programmer should be subscribed to the newsletter from @uidotdev. Not only do they manage to succinctly cover the hot news in the JavaScript world for the week but it they manage to add a refreshing humor to it all.
Async and Await
Javascript course, introduction.
Asynchronous code can become difficult to follow when it has a lot of things going on. async and await are two keywords that can help make asynchronous read more like synchronous code. This can help code look cleaner while keeping the benefits of asynchronous code.
For example, the two code blocks below do the exact same thing. They both get information from a server, process it, and return a promise.
The second example looks much more like the kind of functions you are used to writing. However, did you notice the async keyword before the function declaration? How about the await keyword before server.getPeople() ?
If you’d like to try running these functions on your own, paste the following code block representing a server before the function definitions. How this “server” works is not important and is just an abstraction. The goal here is so that you can see that both functions behave exactly the same and return a promise.
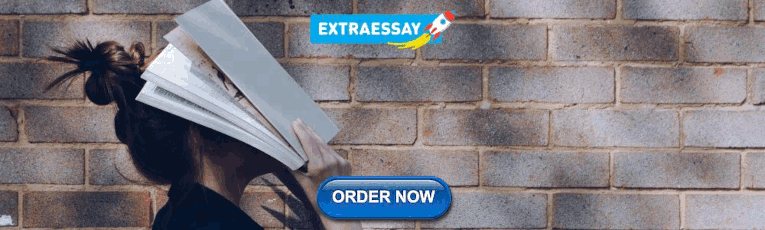
Lesson overview
This section contains a general overview of topics that you will learn in this lesson.
- Explain how you declare an async function.
- Explain what the async keyword does.
- Explain what the await keyword does.
- Explain what an async function returns.
- Explain what happens when an error is thrown inside an async function.
- Explain how you can handle errors inside an async function.
The async keyword
The async keyword is what lets the JavaScript engine know that you are declaring an asynchronous function. This is required to use await inside any function. When a function is declared with async , it automatically returns a promise; returning in an async function is the same as resolving a promise. Likewise, throwing an error will reject the promise.
An important thing to understand is async functions are just syntactical sugar for promises .
The async keyword can also be used with any of the ways a function can be created. Said differently: it is valid to use an async function anywhere you can use a normal function. Below you will see some examples that may not be intuitive. If you don’t understand them, come back and take a look when you are done with the assignments.
The await keyword
await does the following: it tells JavaScript to wait for an asynchronous action to finish before continuing the function. It’s like a ‘pause until done’ keyword. The await keyword is used to get a value from a function where you would normally use .then() . Instead of calling .then() after the asynchronous function, you would assign a variable to the result using await . Then you can use the result in your code as you would in your synchronous code.
Error handling
Handling errors in async functions is very easy. Promises have the .catch() method for handling rejected promises, and since async functions just return a promise, you can call the function, and append a .catch() method to the end.
But there is another way: the mighty try/catch block! If you want to handle the error directly inside the async function, you can use try/catch just like you would inside synchronous code.
Doing this can look messy, but it is a very easy way to handle errors without appending .catch() after your function calls. How you handle the errors is up to you, and which method you use should be determined by how your code was written. You will get a feel for what needs to be done over time. The assignments will also help you understand how to handle your errors.
Remember the Giphy API practice project? (If not, you should go back and complete the API lesson). We are going to convert the promise based code into async/await compatible code. Here’s a refresher of the code we are starting with:
Since await does not work on the global scope, we will have to create an async function that wraps our API call to Giphy.
Now that we have a function that is asynchronous, we can then start refactoring from using promises to using await :
Since response is still the same object we have passed to the .then() block at the start, we still need to use the .json() method, which in turn returns a promise. Because .json() returns a promise, we can use await to assign the response to a variable.
To use this function, we just need to call it with getCats() in our code.
This code will behave exactly like the code from the last lesson; it just looks a bit different after refactoring. async/await are very useful tools when it comes to cleaning up asynchronous JavaScript code. It is important to remember async/await are just promises written in a different way. Do the assignments below, and dive deeper into the understanding of async/await .
- Read this Async and Await article for a solid introduction to async/await. This Async and Await examples article also has some good examples of its use.
- Watch this Async and Await video by Wes Bos for a good overview on async/await and its purpose, along with a special trick.
Knowledge check
The following questions are an opportunity to reflect on key topics in this lesson. If you can’t answer a question, click on it to review the material, but keep in mind you are not expected to memorize or master this knowledge.
- How do you declare an async function?
- What does the async keyword do?
- What does the await keyword do?
- What is returned from an async function?
- What happens when an error is thrown inside an async function?
- How can you handle errors inside an async function?
Additional resources
This section contains helpful links to related content. It isn’t required, so consider it supplemental.
- This Change promises to async/await video is an example of how you can change callbacks, to promises, to async/await.
- This Promises, Async and Await video gives a comprehensive view of Promises, async, and await.
- For a more interactive explanation and example, try a Scrim on async and await .
- This blog post about Promises From The Ground Up delves into how Promises work.
Support us!
The odin project is funded by the community. join us in empowering learners around the globe by supporting the odin project.
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript async.
"async and await make promises easier to write"
async makes a function return a Promise
await makes a function wait for a Promise
Async Syntax
The keyword async before a function makes the function return a promise:
Is the same as:
Here is how to use the Promise:
Try it Yourself »
Or simpler, since you expect a normal value (a normal response, not an error):
Await Syntax
The await keyword can only be used inside an async function.
The await keyword makes the function pause the execution and wait for a resolved promise before it continues:
Advertisement
Let's go slowly and learn how to use it.
Basic Syntax
The two arguments (resolve and reject) are pre-defined by JavaScript.
We will not create them, but call one of them when the executor function is ready.
Very often we will not need a reject function.
Example without reject
Waiting for a timeout, waiting for a file, browser support.
ECMAScript 2017 introduced the JavaScript keywords async and await .
The following table defines the first browser version with full support for both:
Chrome 55 | Edge 15 | Firefox 52 | Safari 11 | Opera 42 |
Dec, 2016 | Apr, 2017 | Mar, 2017 | Sep, 2017 | Dec, 2016 |

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
JavaScript Async/Await Tutorial – Learn Callbacks, Promises, and Async/Await in JS by Making Ice Cream 🍧🍨🍦
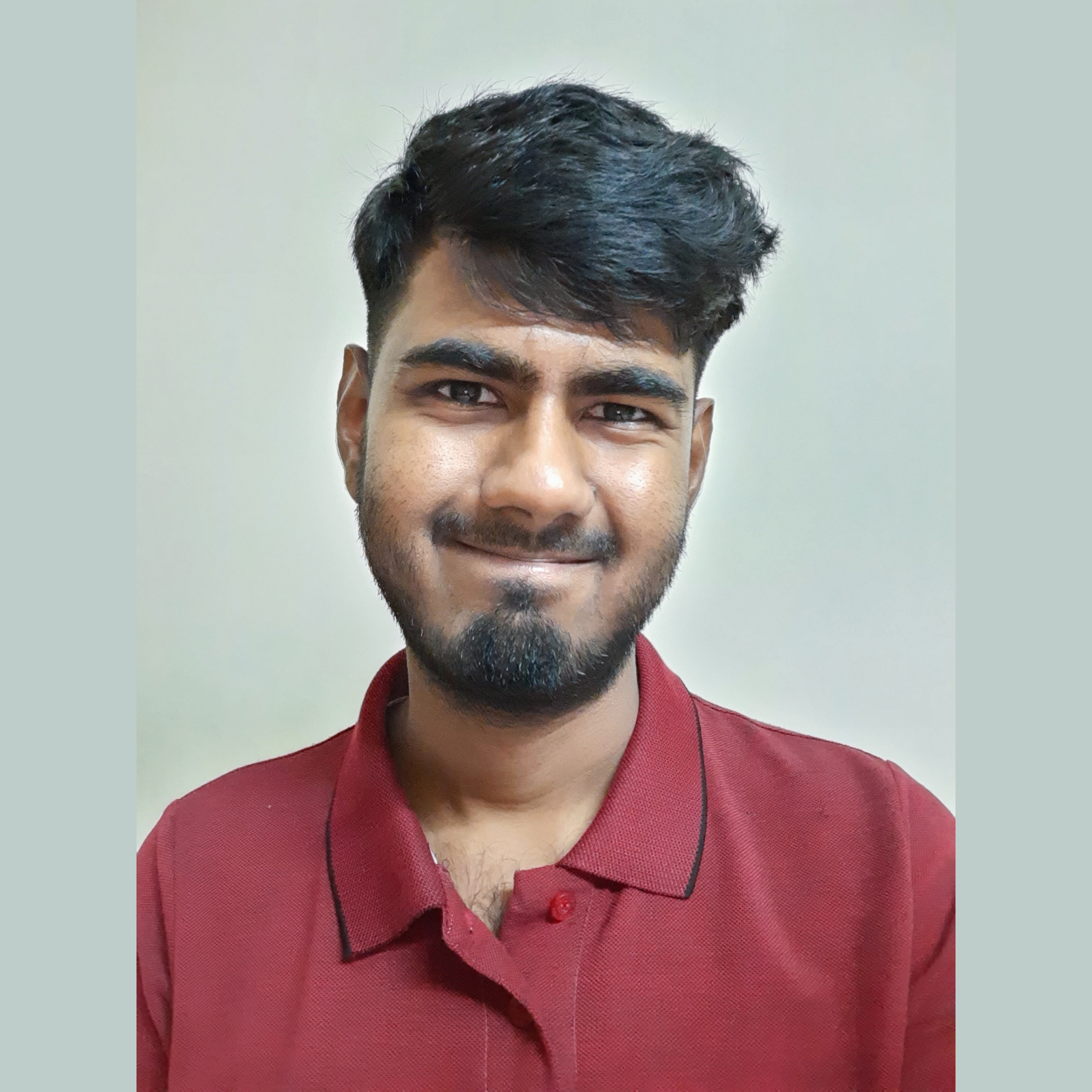
Today we're going to build and run an ice cream shop and learn asynchronous JavaScript at the same time. Along the way, you'll learn how to use:
- Async / Await
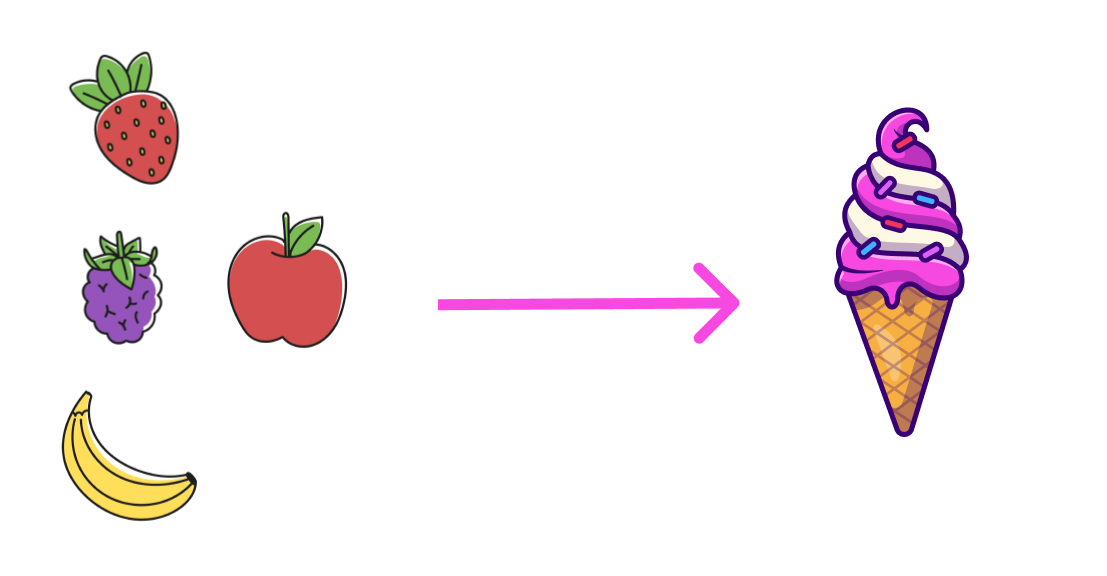
Here's what we'll cover in this article:
What is asynchronous javascript, synchronous vs asynchronous javascript.
- How Callbacks Work in JavaScript
- How Promises Work in JavaScript
- How Async / Await Works in JavaScript
So let's dive in!
You can watch this tutorial on YouTube as well if you like:
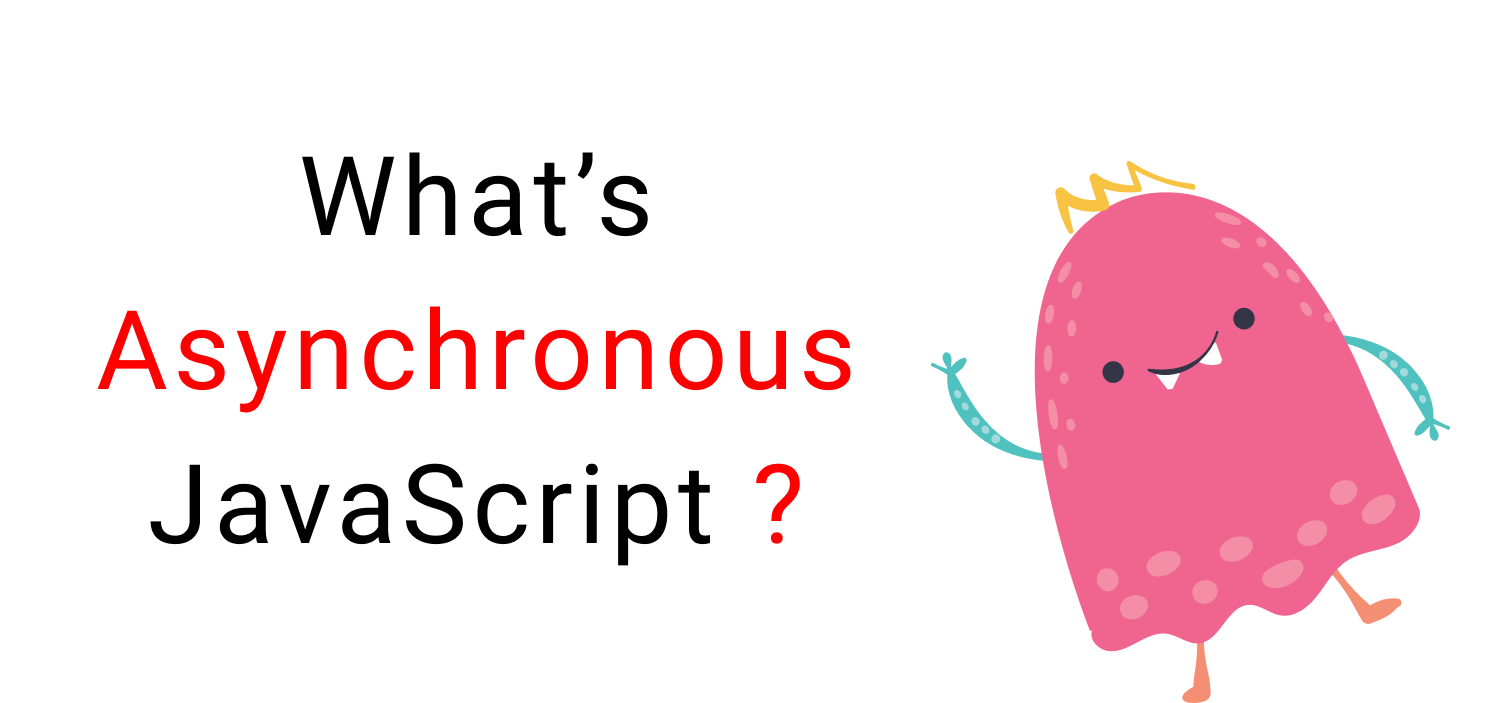
If you want to build projects efficiently, then this concept is for you.
The theory of async JavaScript helps you break down big complex projects into smaller tasks.
Then you can use any of these three techniques – callbacks, promises or Async/await – to run those small tasks in a way that you get the best results.
Let's dive in!🎖️
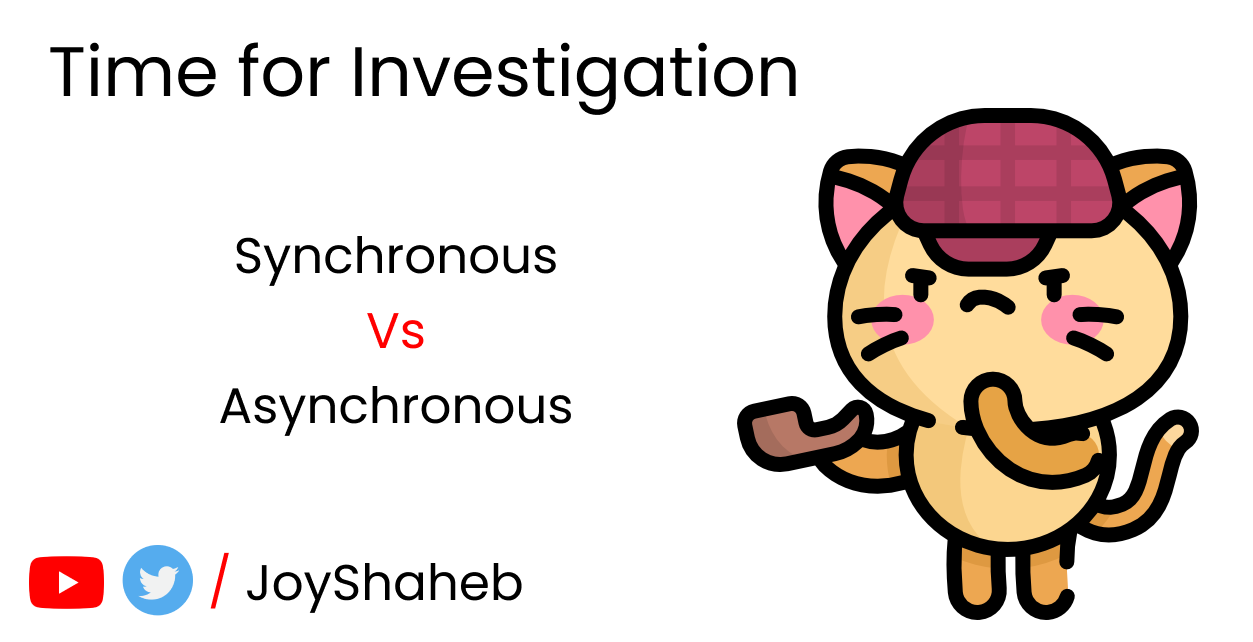
What is a Synchronous System?
In a synchronous system, tasks are completed one after another.
Think of this as if you have just one hand to accomplish 10 tasks. So, you have to complete one task at a time.
Take a look at the GIF 👇 – one thing is happening at a time here:
You'll see that until the first image is loaded completely, the second image doesn't start loading.
Well, JavaScript is by default Synchronous [single threaded] . Think about it like this – one thread means one hand with which to do stuff.
What is an Asynchronous System?
In this system, tasks are completed independently.
Here, imagine that for 10 tasks, you have 10 hands. So, each hand can do each task independently and at the same time.
Take a look at the GIF 👇 – you can see that each image loads at the same time.
Again, all the images are loading at their own pace. None of them is waiting for any of the others.
To Summarize Synchronous vs Asynchronous JS:
When three images are on a marathon, in a:
- Synchronous system, three images are in the same lane. One can't overtake the other. The race is finished one by one. If image number 2 stops, the following image stops.
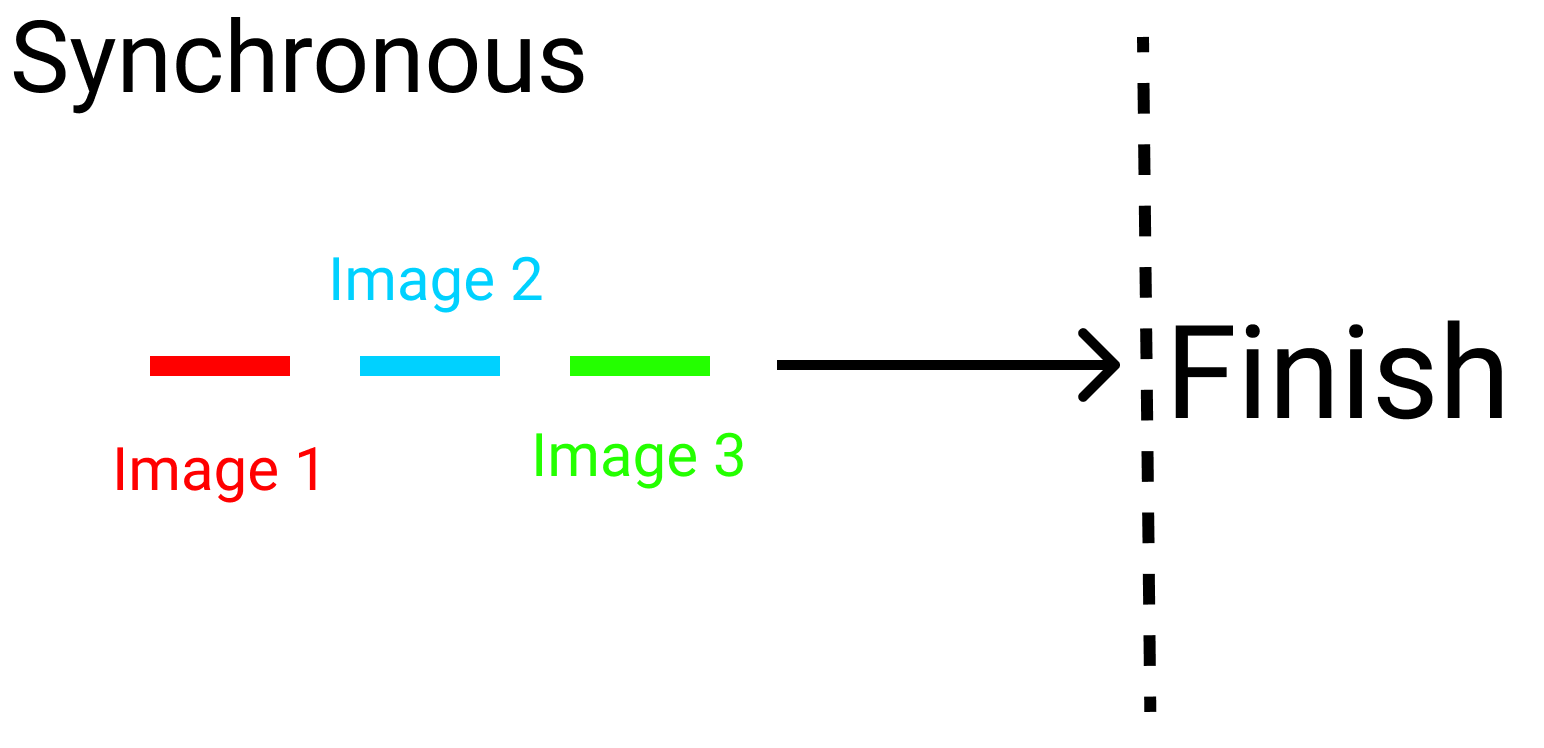
- Asynchronous system, the three images are in different lanes. They'll finish the race on their own pace. Nobody stops for anybody:
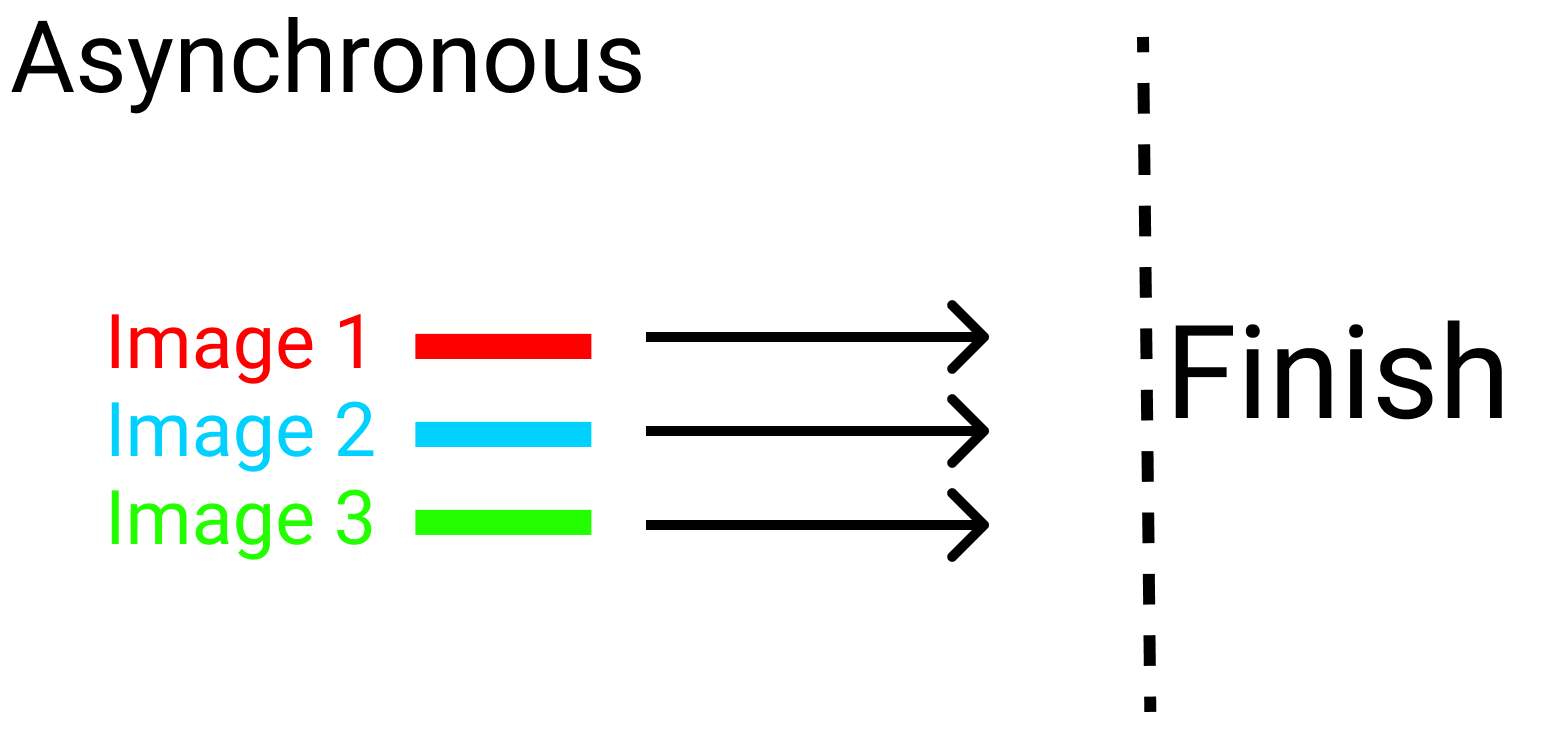
Synchronous and Asynchronous Code Examples
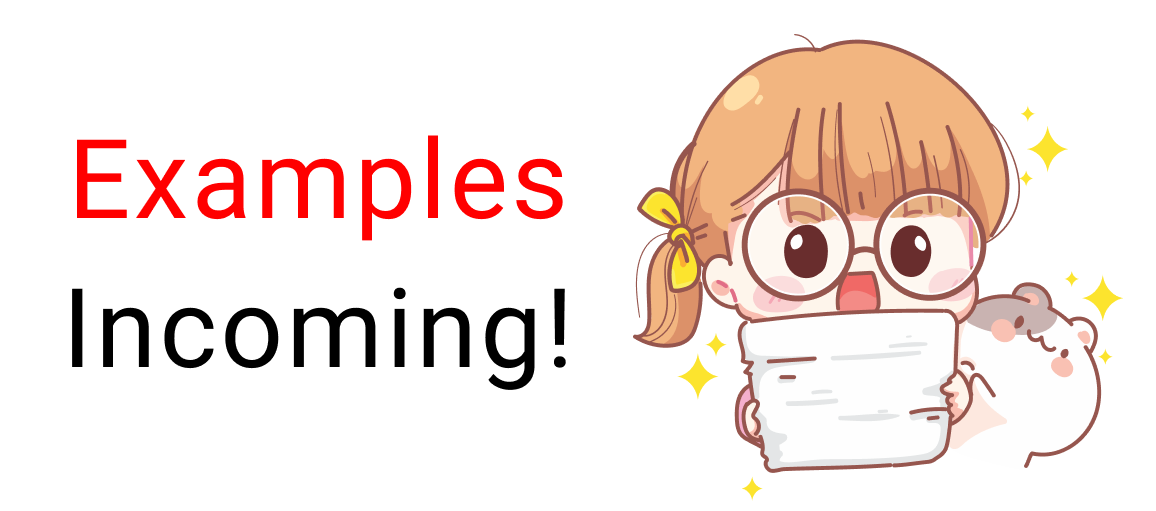
Before starting our project, let's look at some examples and clear up any doubts.
Synchronous Code Example
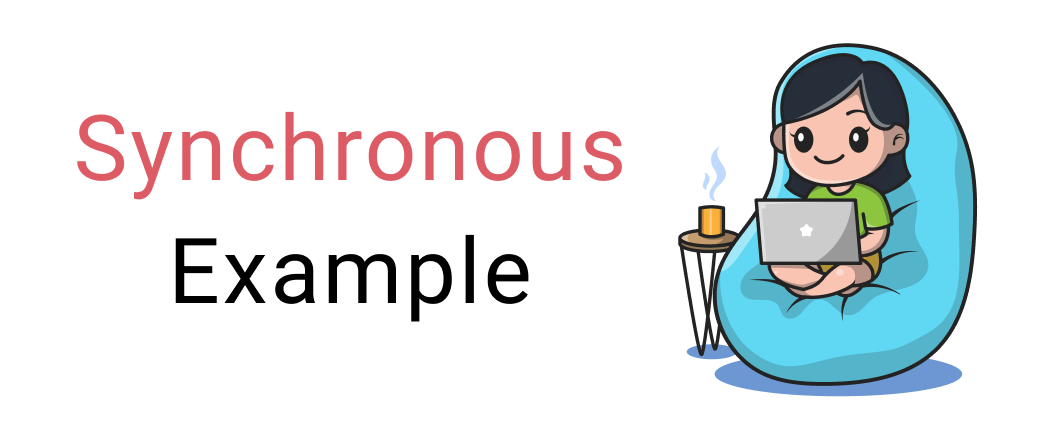
To test a synchronous system, write this code in JavaScript:
Here's the result in the console: 👇
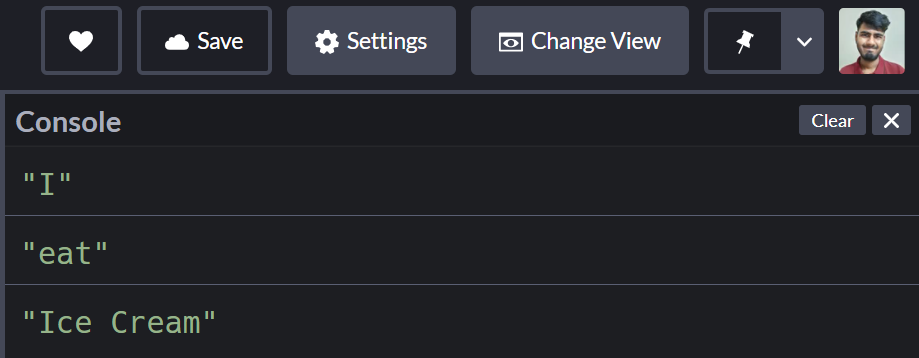
Asynchronous code example
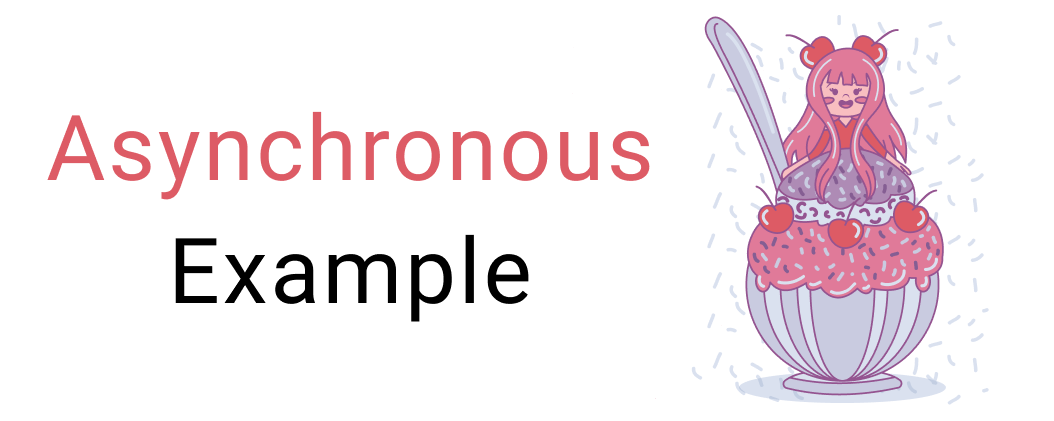
Let's say it takes two seconds to eat some ice cream. Now, let's test out an asynchronous system. Write the below code in JavaScript.
Note: Don't worry, we'll discuss the setTimeout() function later in this article.
And here's the result in the console: 👇
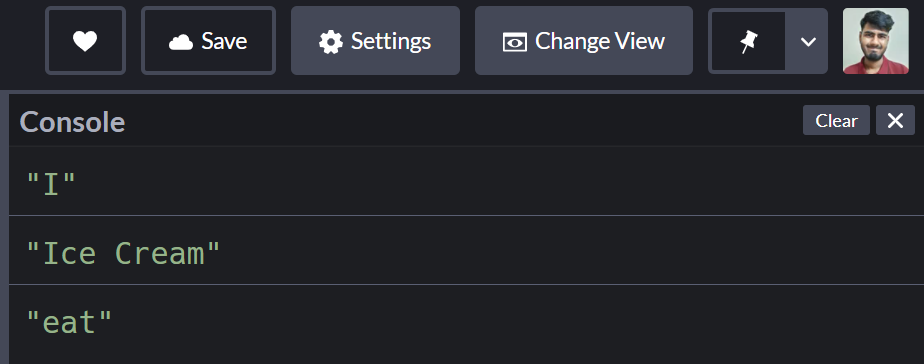
Now that you know the difference between synchronous and async operations, let's build our ice cream shop.
How to Setup our Project
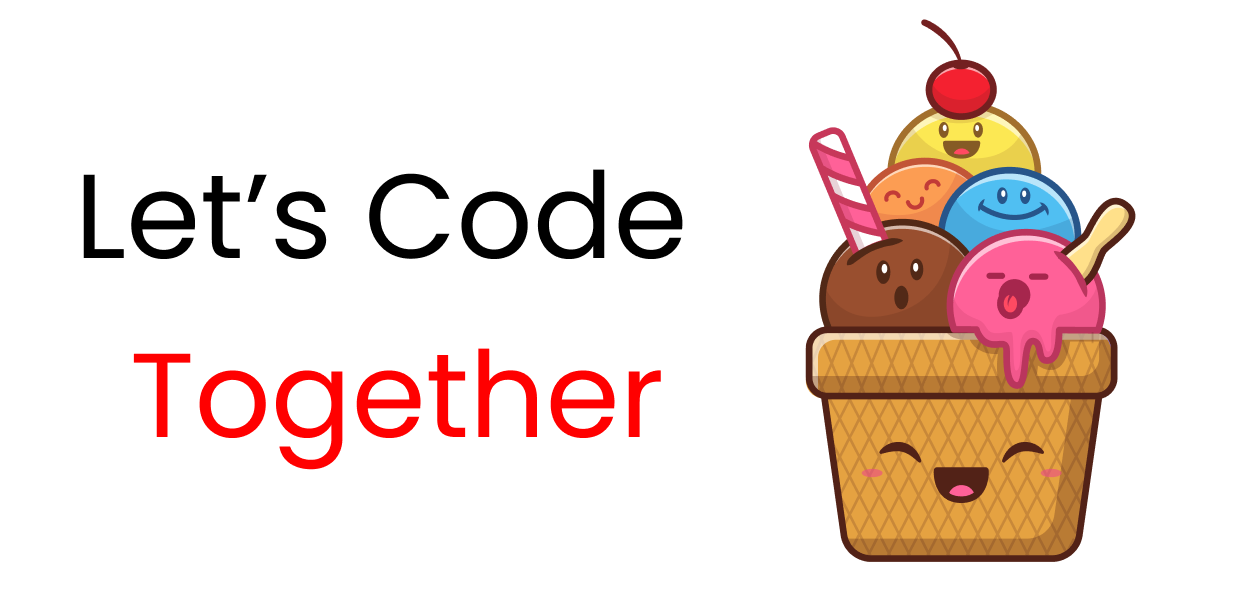
For this project you can just open Codepen.io and start coding. Or, you can do it in VS code or the editor of your choice.
Open the JavaScript section, and then open your developer console. We'll write our code and see the results in the console.
What are Callbacks in JavaScript?
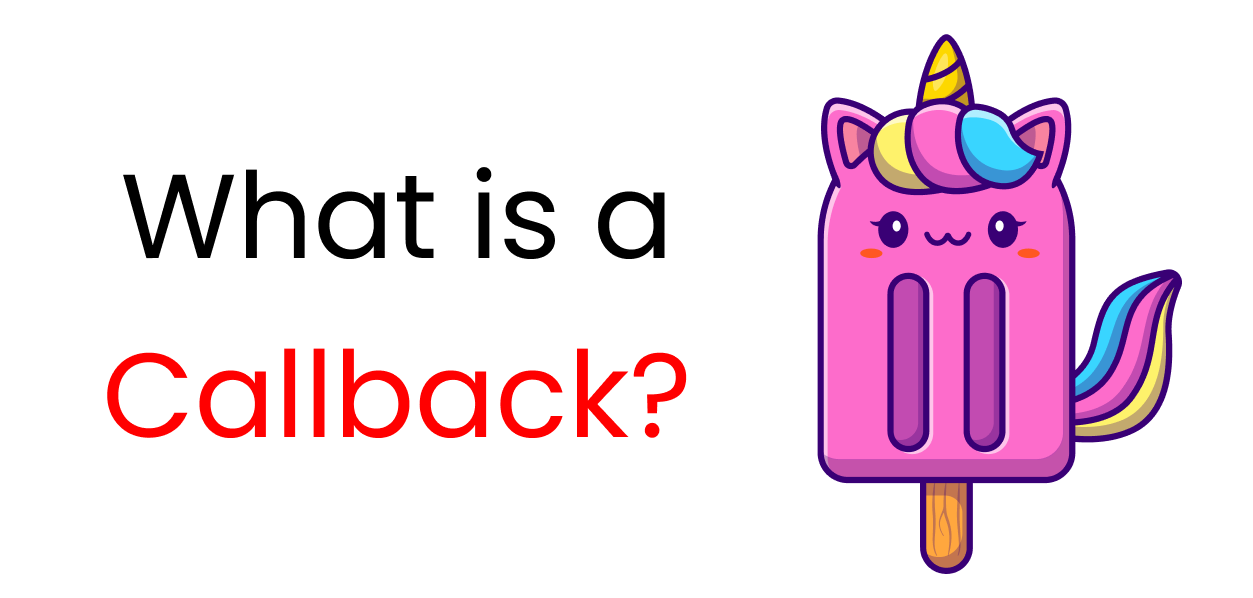
When you nest a function inside another function as an argument, that's called a callback.
Here's an illustration of a callback:
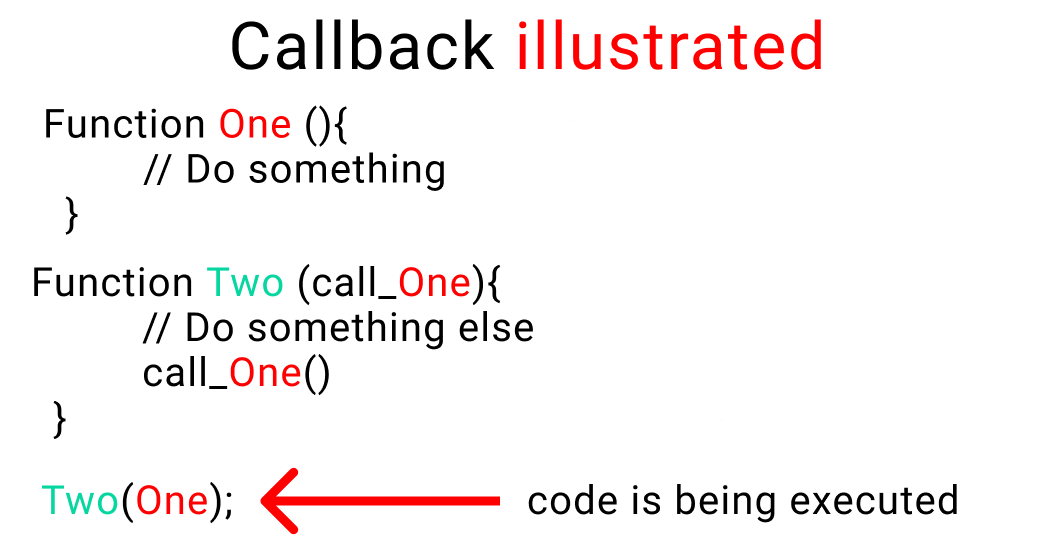
Don't worry, we'll see some examples of callbacks in a minute.
Why do we use callbacks?
When doing a complex task, we break that task down into smaller steps. To help us establish a relationship between these steps according to time (optional) and order, we use callbacks.
Take a look at this example:👇
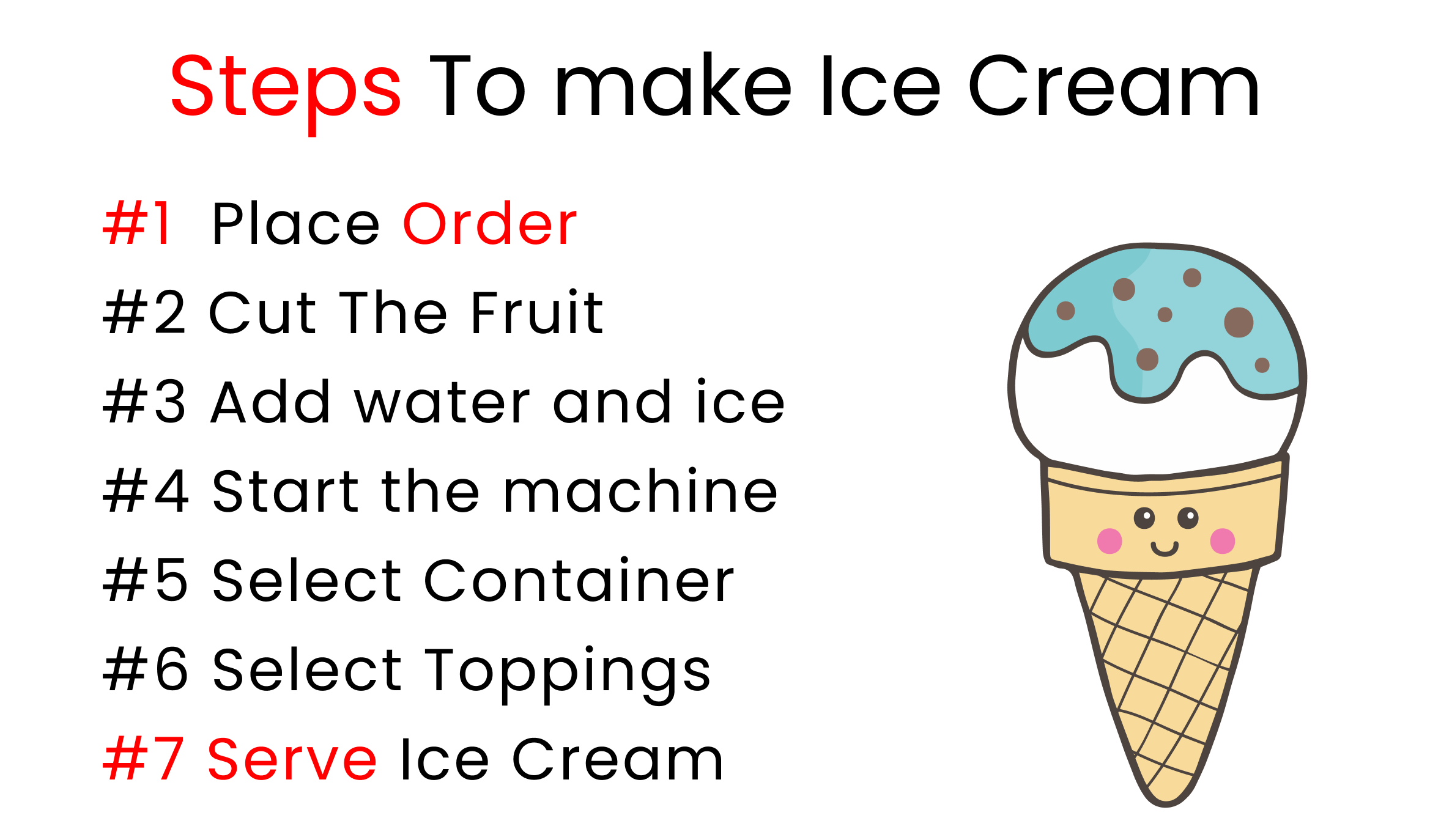
These are the small steps you need to take to make ice cream. Also note that in this example, the order of the steps and timing are crucial. You can't just chop the fruit and serve ice cream.
At the same time, if a previous step is not completed, we can't move on to the next step.
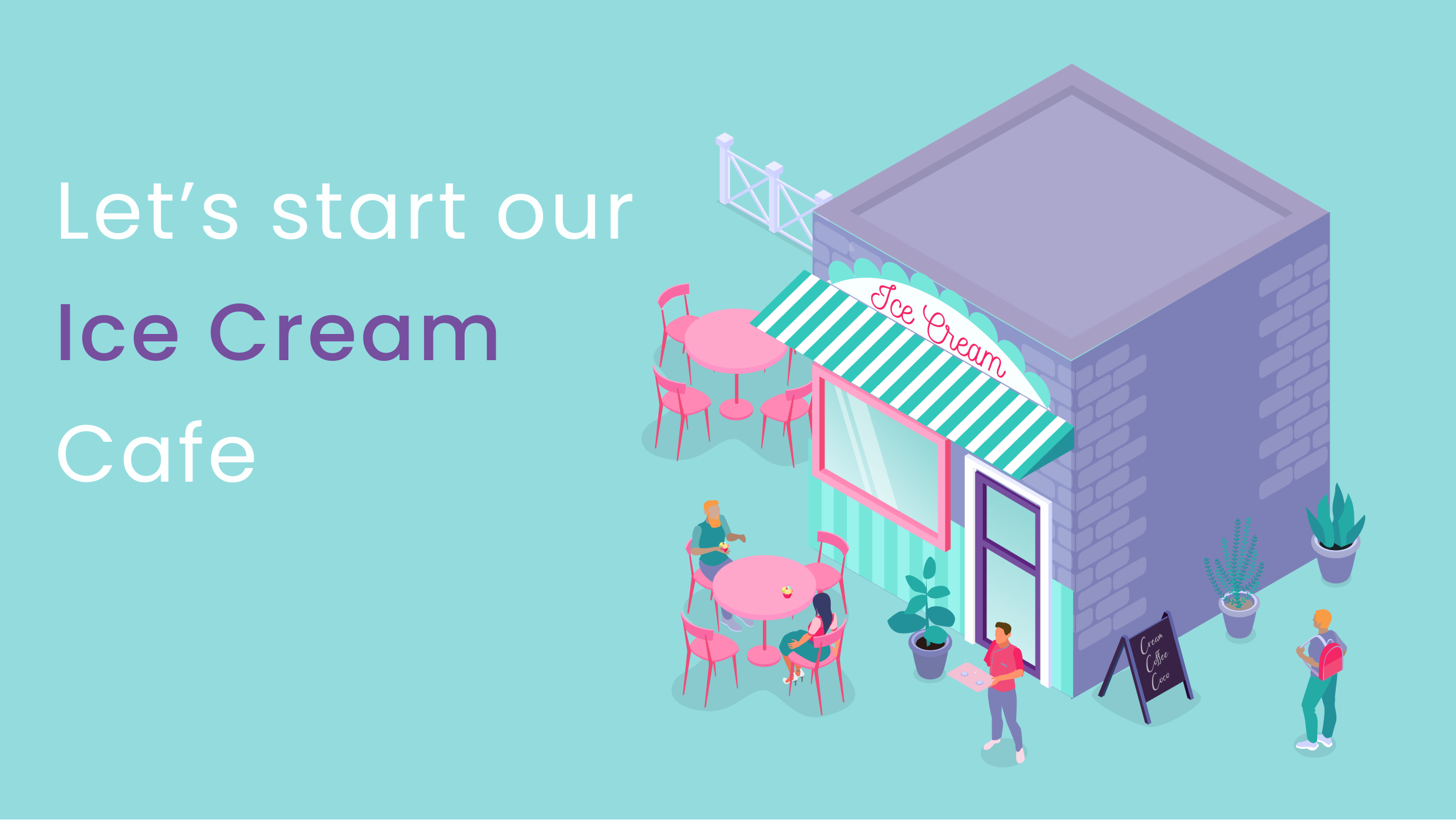
To explain that in more detail, let's start our ice cream shop business.
But Wait...
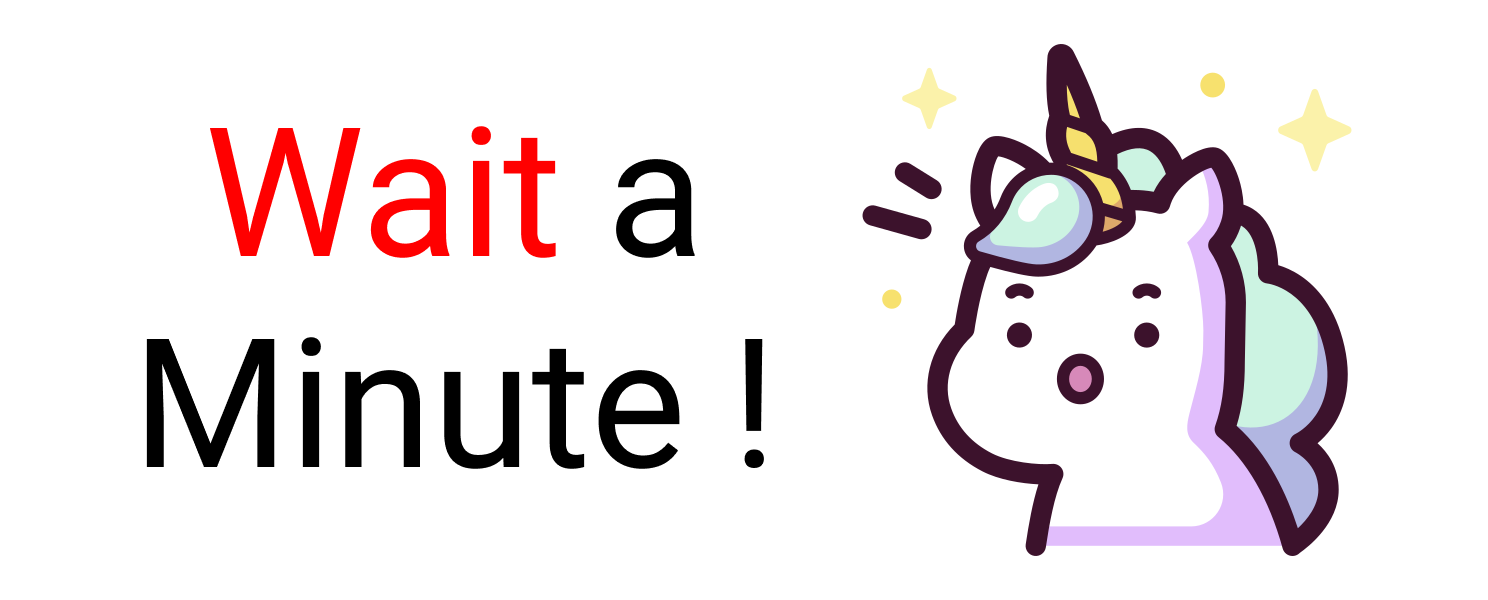
The shop will have two parts:
- The storeroom will have all the ingredients [Our Backend]
- We'll produce ice cream in our kitchen [The frontend]
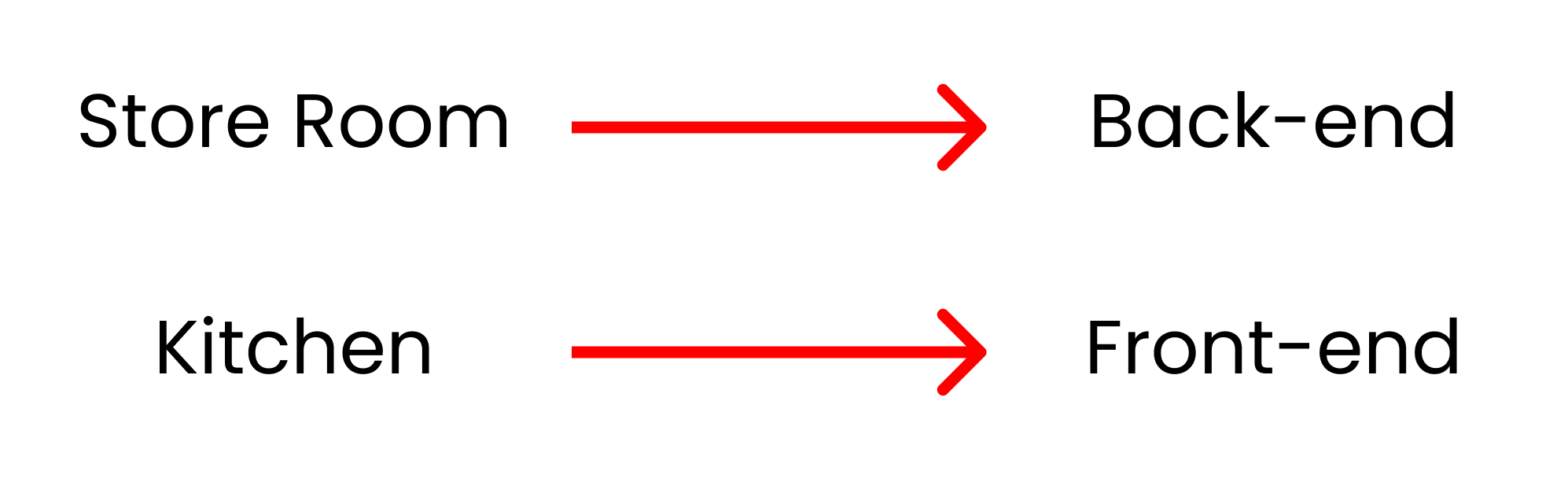
Let's store our data
Now, we're gonna store our ingredients inside an object. Let's start!
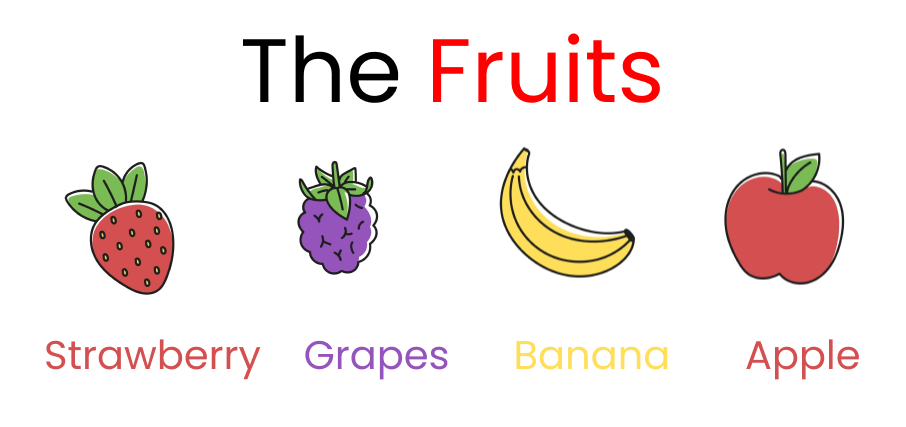
You can store the ingredients inside objects like this: 👇
Our other ingredients are here: 👇
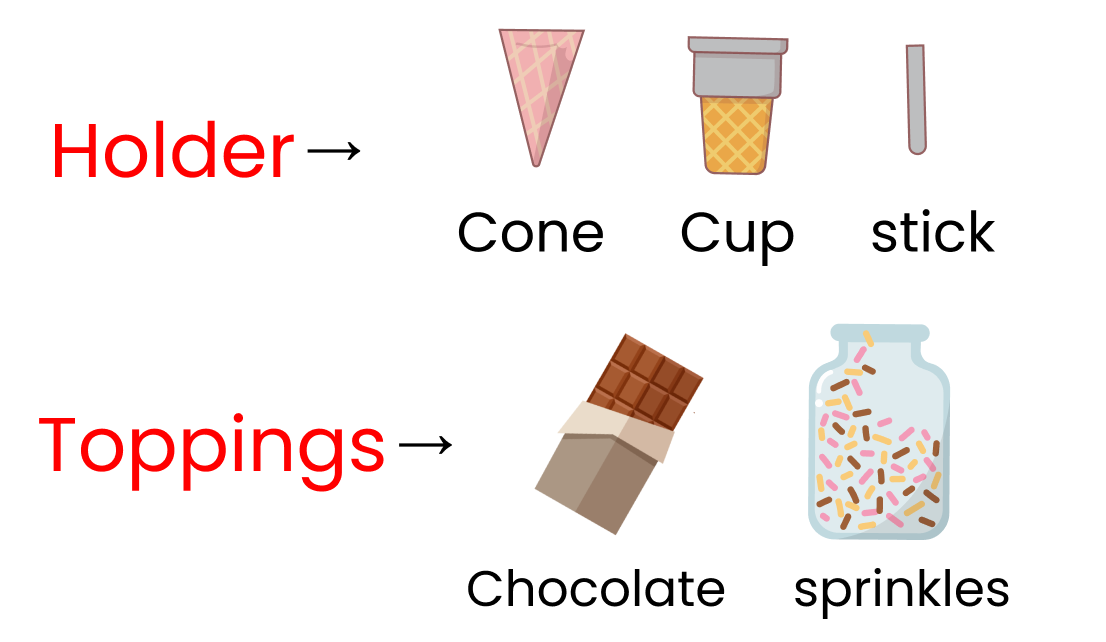
You can store these other ingredients in JavaScript objects like this: 👇
The entire business depends on what a customer orders . Once we have an order, we start production and then we serve ice cream. So, we'll create two functions ->
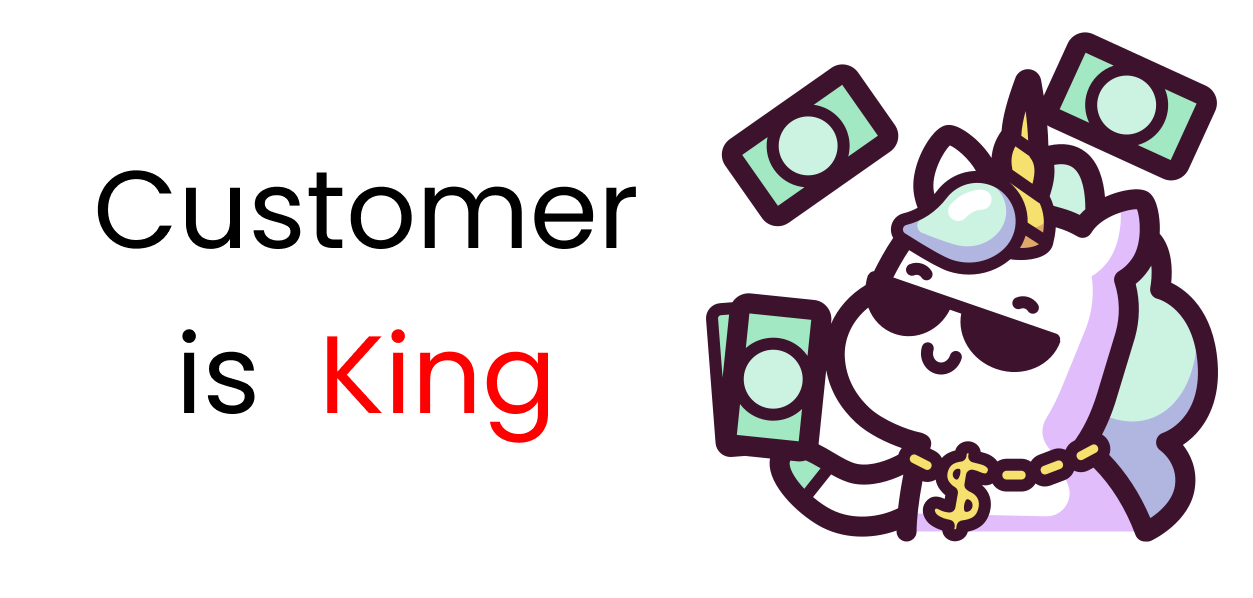
This is how it all works: 👇
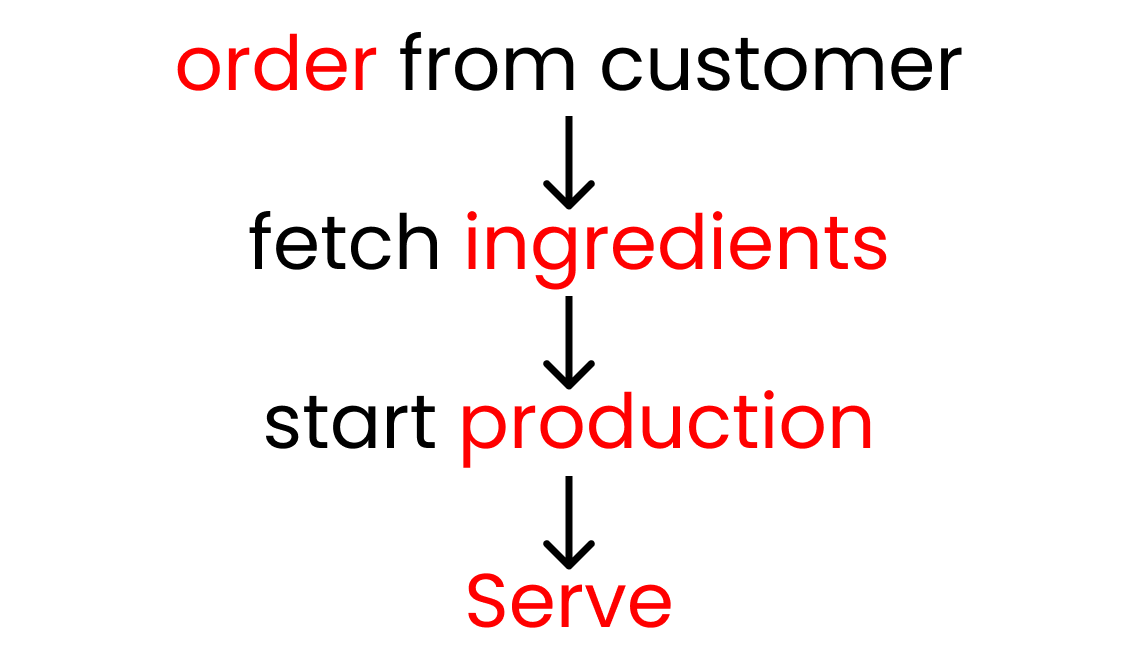
Let's make our functions. We'll use arrow functions here:
Now, let's establish a relationship between these two functions using a callback, like this: 👇
Let's do a small test
We'll use the console.log() function to conduct tests to clear up any doubts we might have regarding how we established the relationship between the two functions.
To run the test, we'll call the order function. And we'll add the second function named production as its argument.
Here's the result in our console 👇
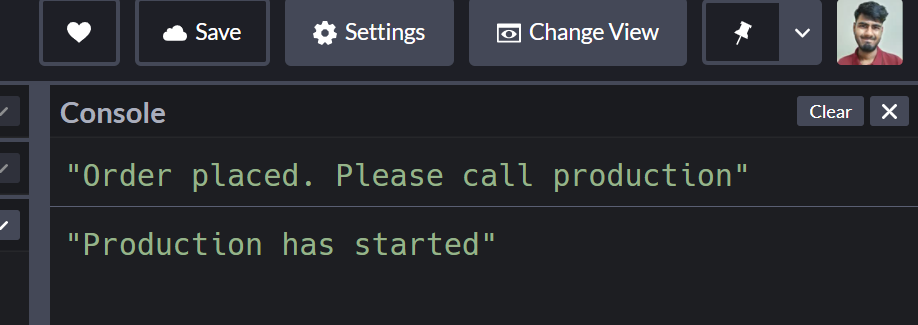
Take a break
So far so good – take a break!
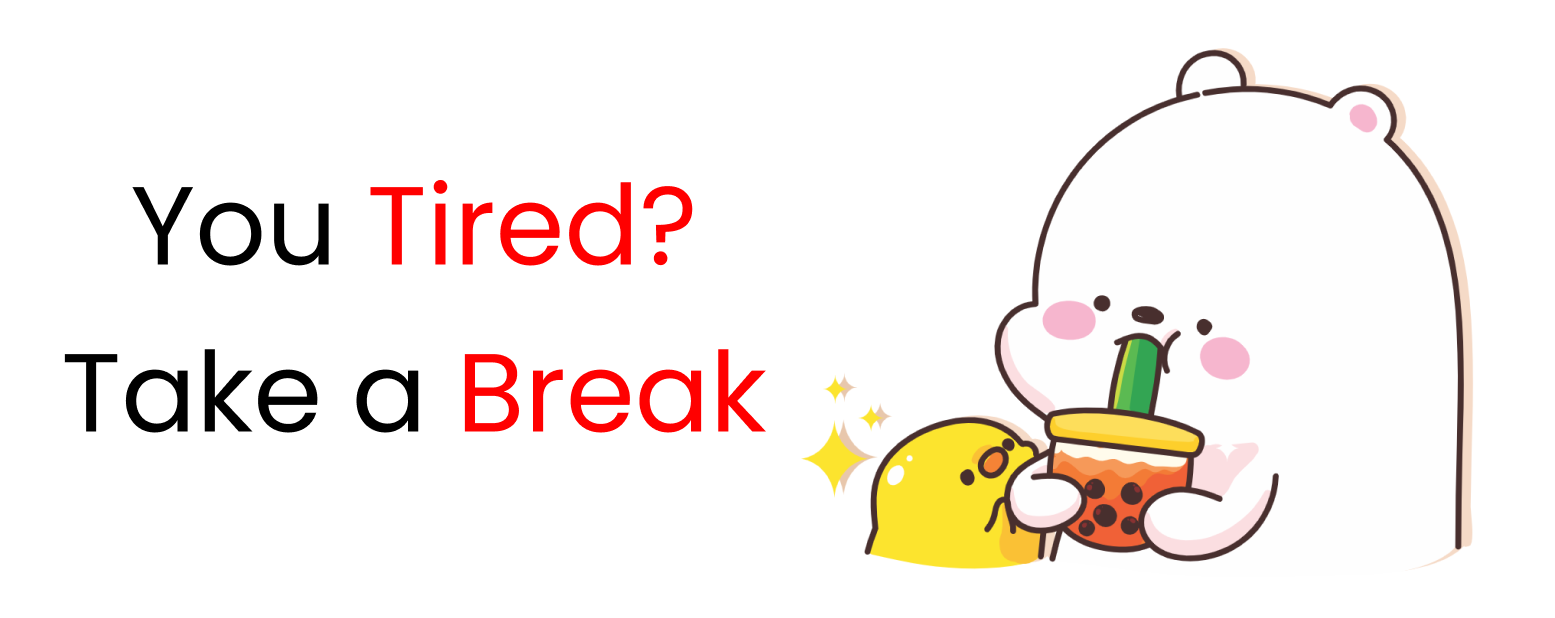
Clear out the console.log
Keep this code and remove everything [don't delete our stocks variable]. On our first function, pass another argument so that we can receive the order [Fruit name]:
Here are our steps, and the time each step will take to execute.
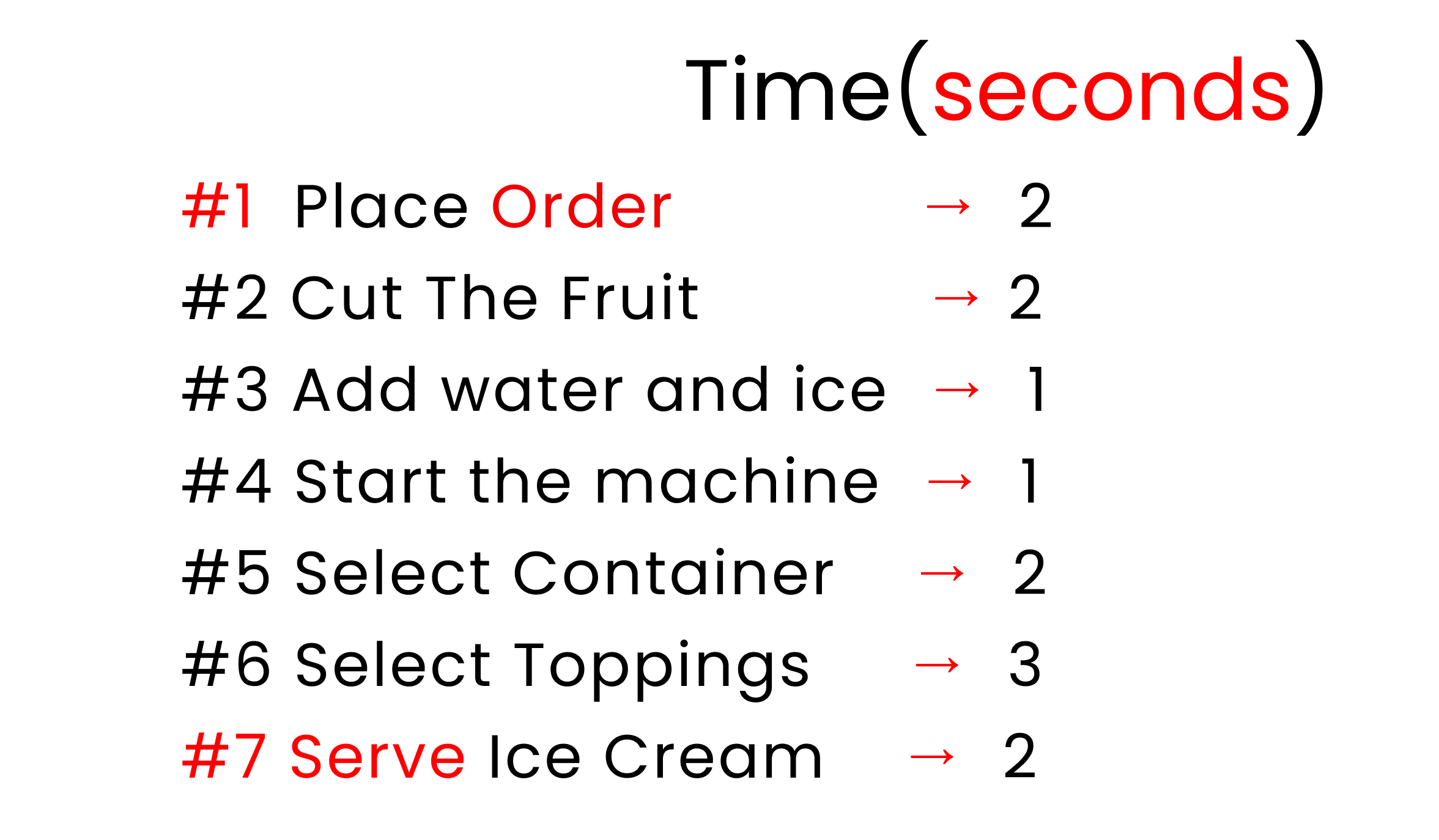
In this chart, you can see that step 1 is to place the order, which takes 2 seconds. Then step 2 is cut the fruit (2 seconds), step 3 is add water and ice (1 second), step 4 is to start the machine (1 second), step 5 is to select the container (2 seconds), step 6 is to select the toppings (3 seconds) and step 7, the final step, is to serve the ice cream which takes 2 seconds.
To establish the timing, the function setTimeout() is excellent as it is also uses a callback by taking a function as an argument.
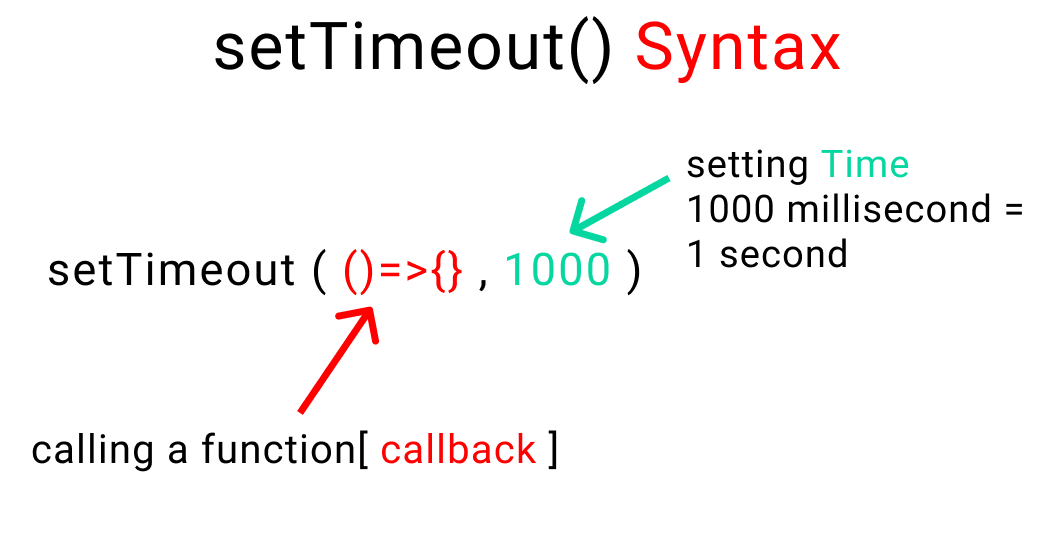
Now, let's select our fruit and use this function:
Note that the result is displayed after 2 seconds.
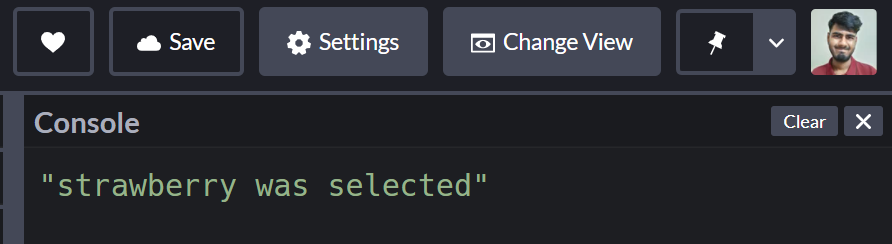
If you're wondering how we picked strawberry from our stock variable, here's the code with the format 👇
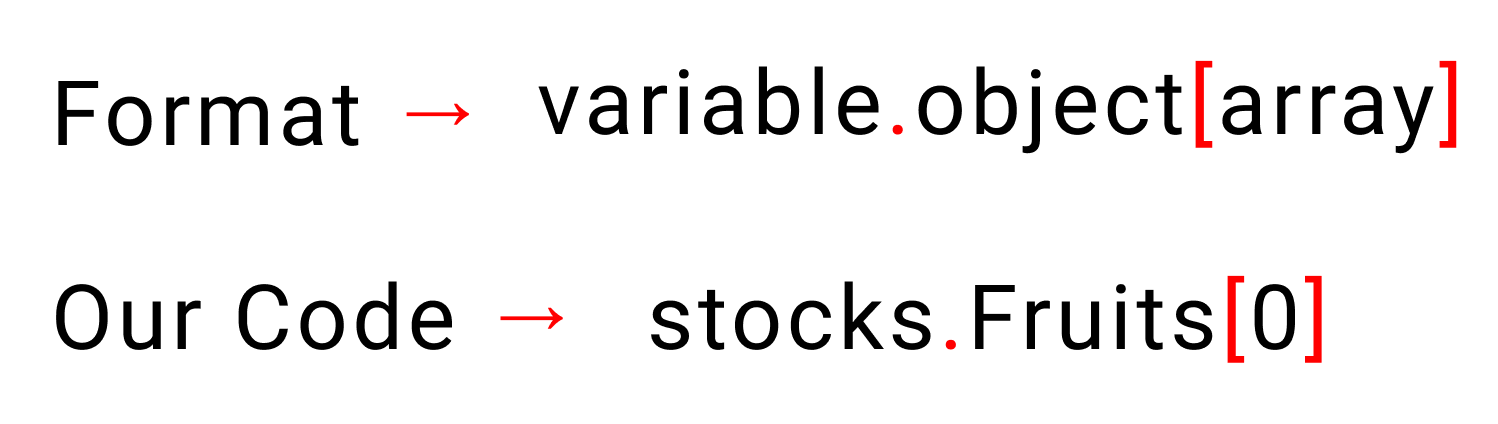
Don't delete anything. Now we'll start writing our production function with the following code.👇 We'll use arrow functions:
And here's the result 👇
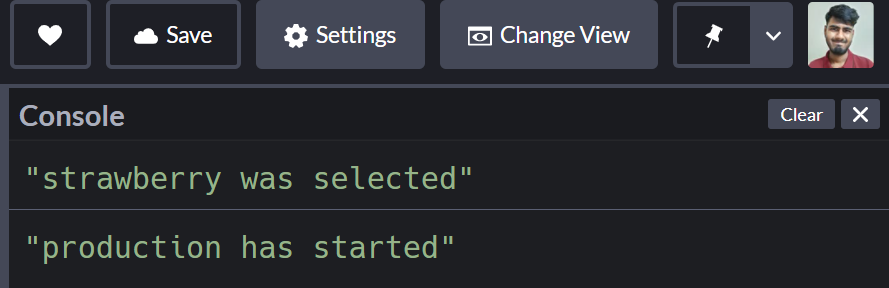
We'll nest another setTimeout function in our existing setTimeout function to chop the fruit. Like this: 👇
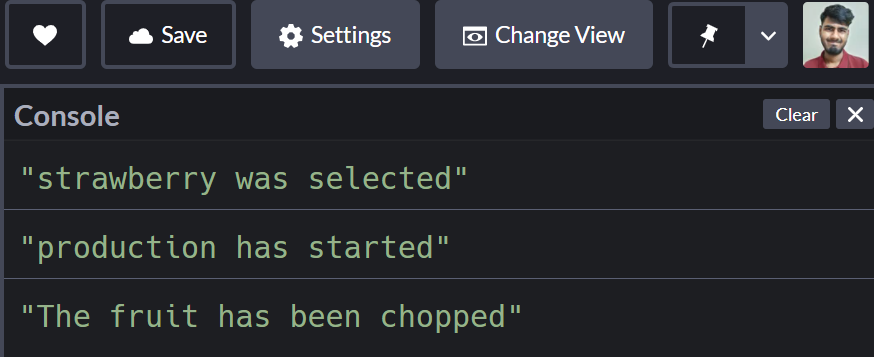
If you remember, here are our steps:
Let's complete our ice cream production by nesting a function inside another function – this is also known as a callback, remember?
And here's the result in the console 👇
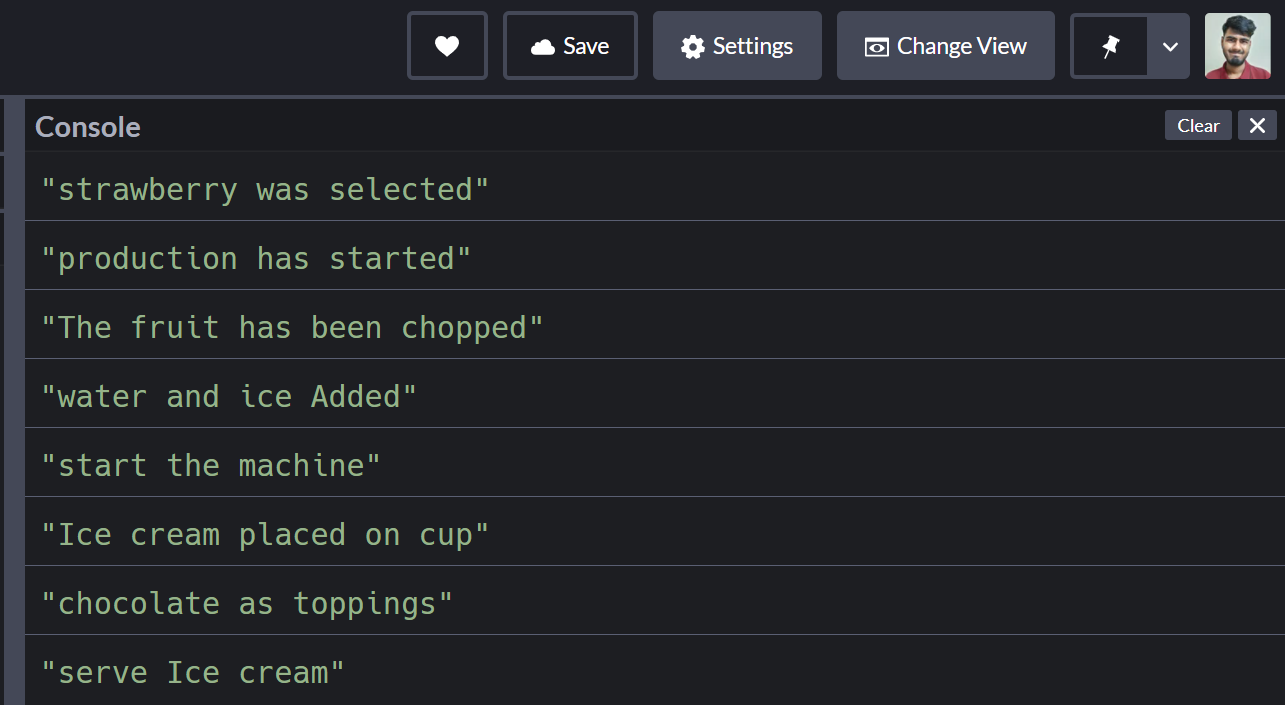
Feeling confused?
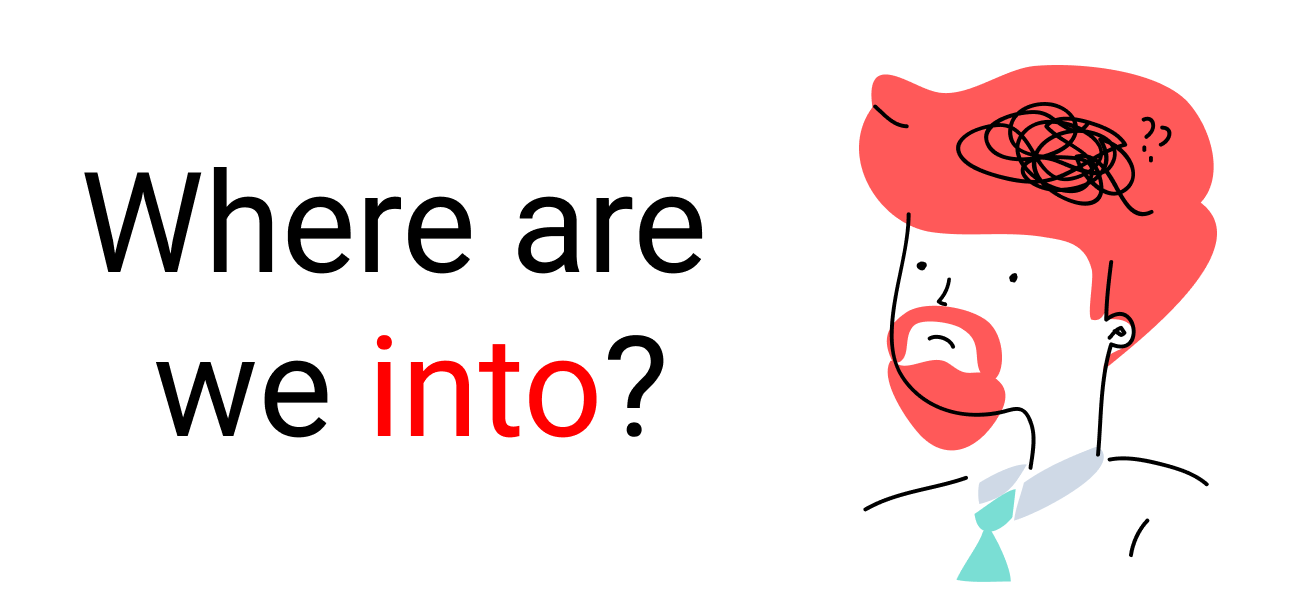
This is called callback hell. It looks something like this (remember that code just above?): 👇
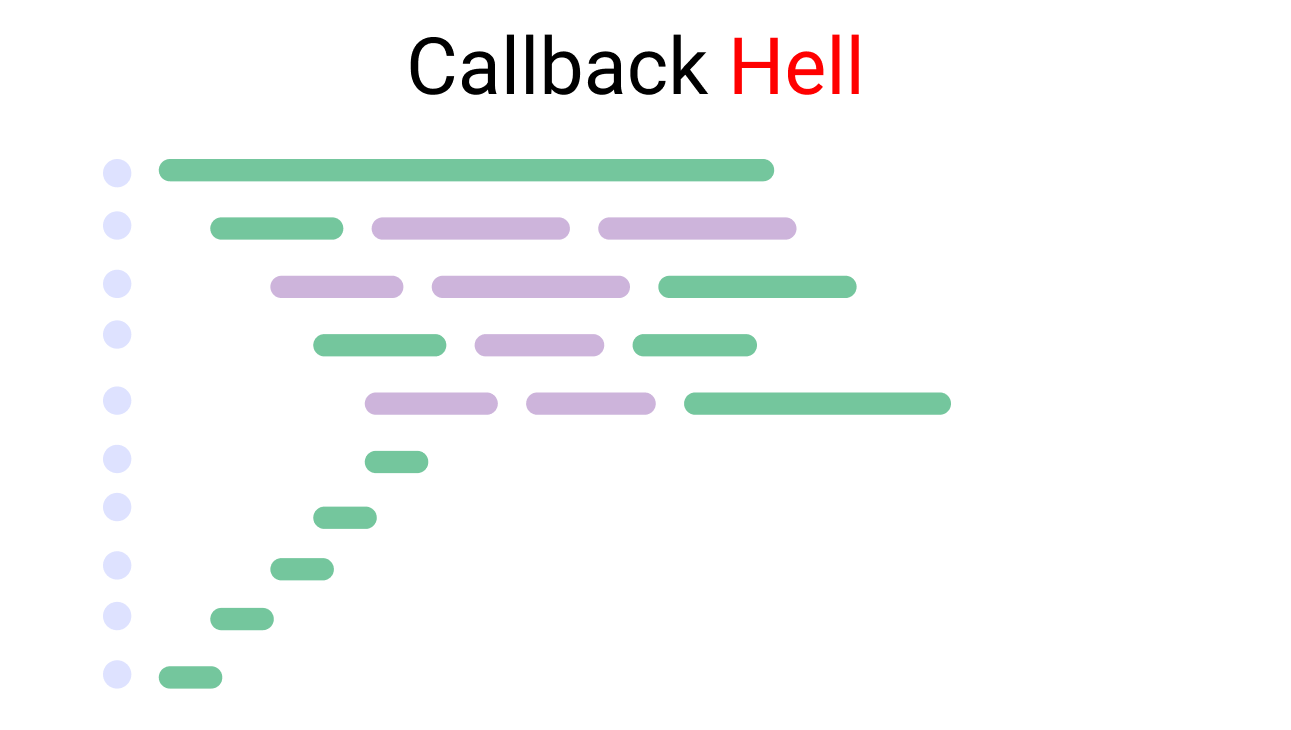
What's the solution to this?
How to Use Promises to Escape Callback Hell
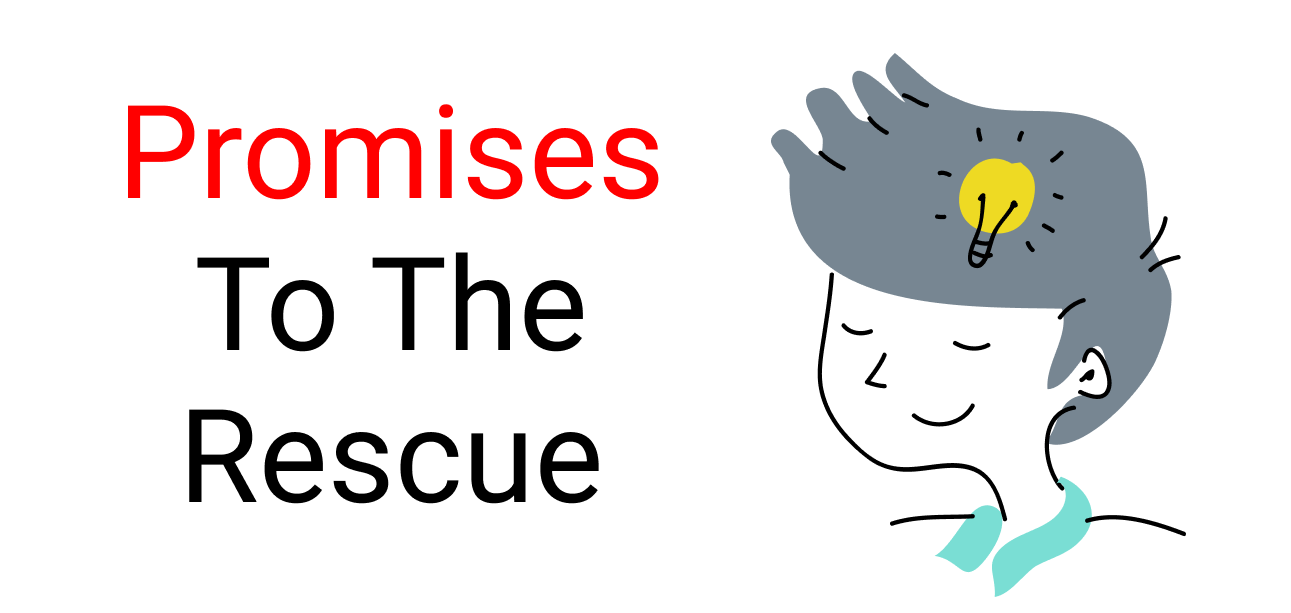
Promises were invented to solve the problem of callback hell and to better handle our tasks.
But first, take a break!
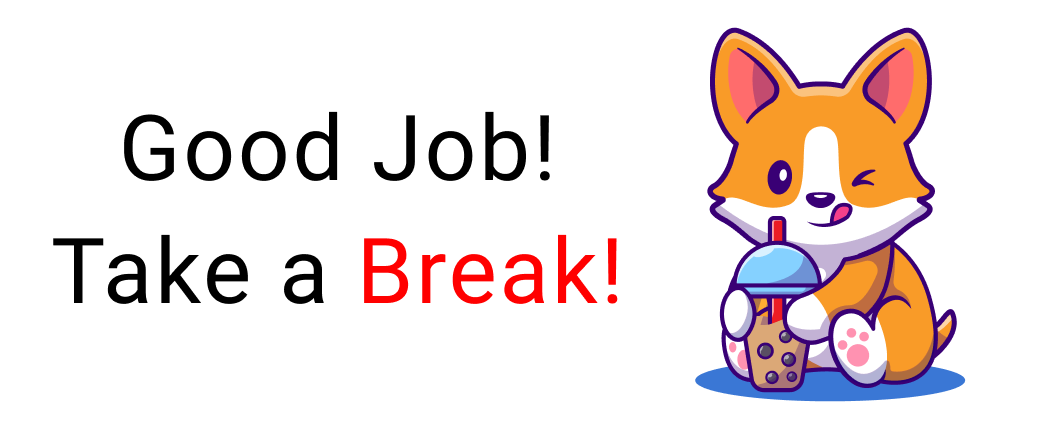
This is how a promise looks:
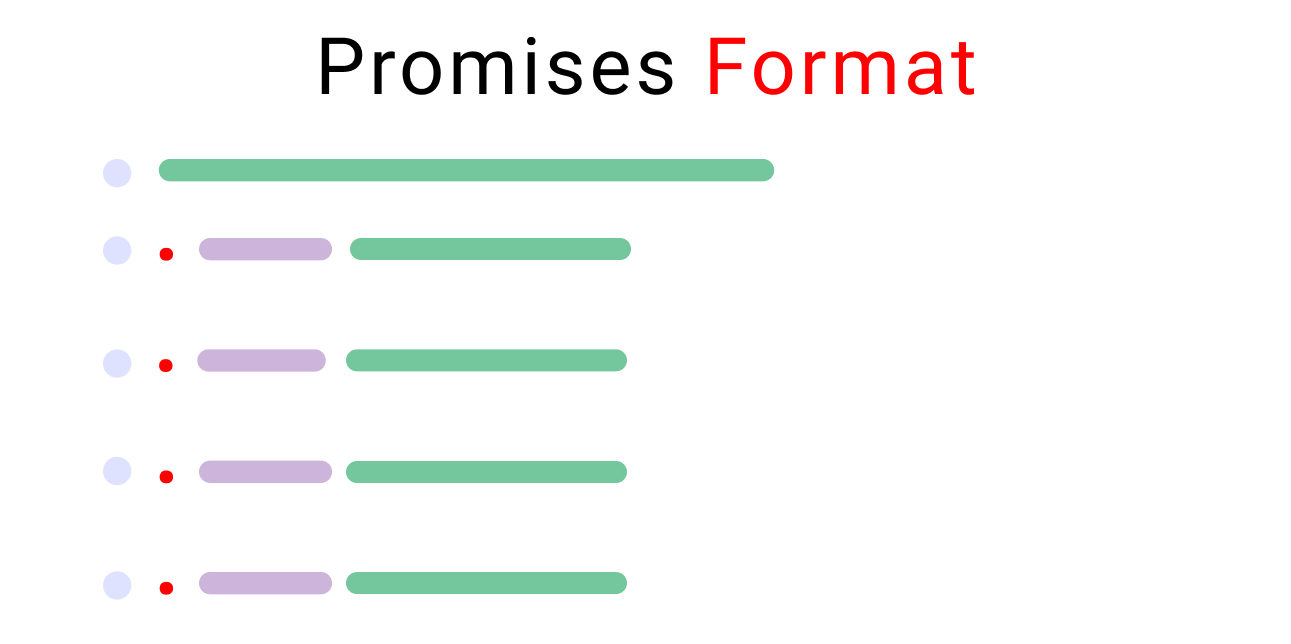
Let's dissect promises together.
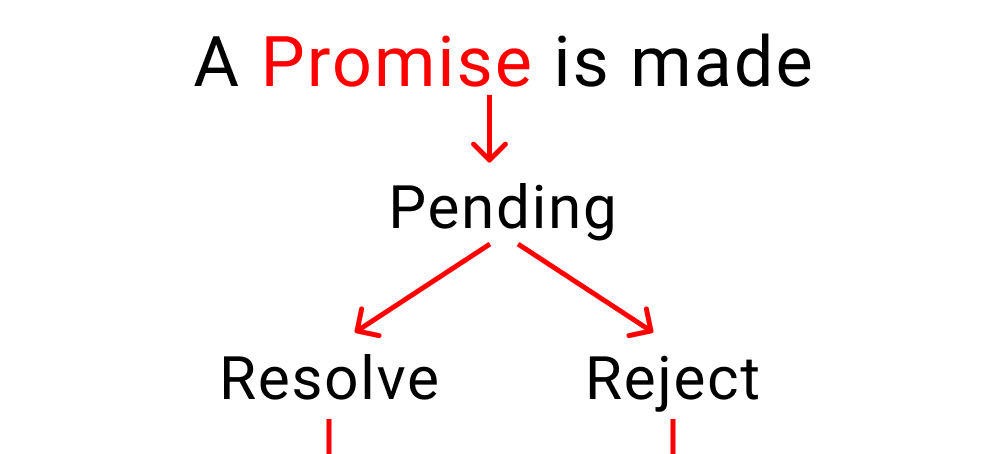
As the above charts show, a promise has three states:
- Pending: This is the initial stage. Nothing happens here. Think of it like this, your customer is taking their time giving you an order. But they haven't ordered anything yet.
- Resolved: This means that your customer has received their food and is happy.
- Rejected: This means that your customer didn't receive their order and left the restaurant.
Let's adopt promises to our ice cream production case study.
But wait...
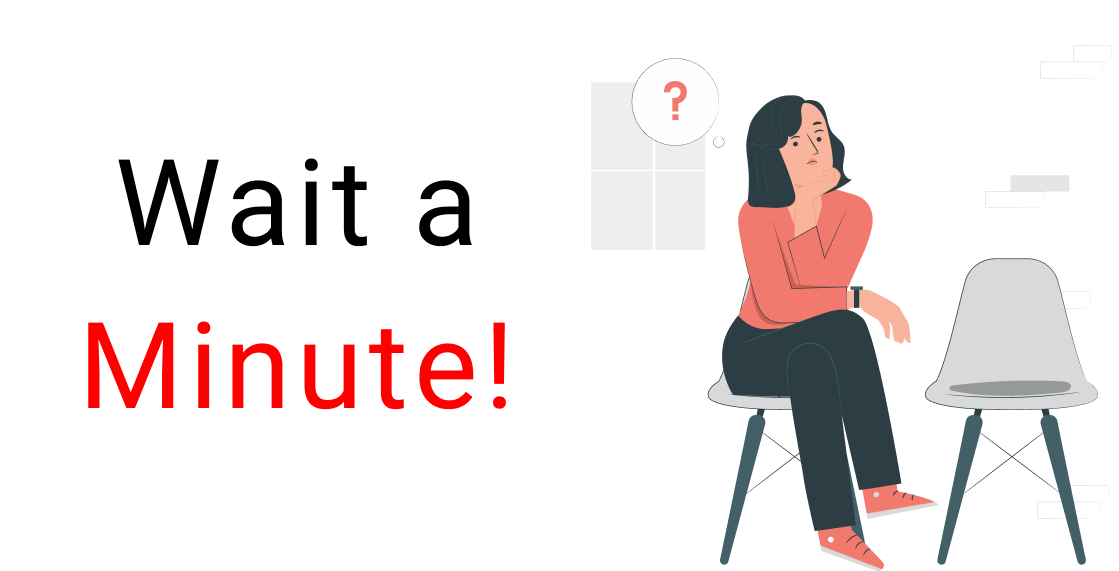
We need to understand four more things first ->
Relationship between time and work
Promise chaining, error handling.
- The .finally handler
Let's start our ice cream shop and understand each of these concepts one by one by taking baby steps.
If you remember, these are our steps and the time each takes to make ice cream"
For this to happen, let's create a variable in JavaScript: 👇
Now create a function named order and pass two arguments named time, work :
Now, we're gonna make a promise to our customer, "We will serve you ice-cream" Like this ->
Our promise has 2 parts:
- Resolved [ ice cream delivered ]
- Rejected [ customer didn't get ice cream ]
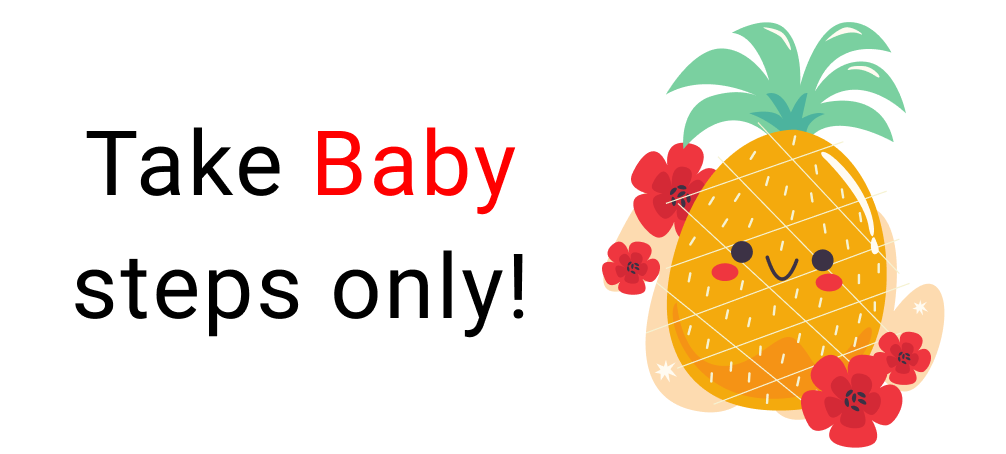
Let's add the time and work factors inside our promise using a setTimeout() function inside our if statement. Follow me 👇
Note: In real life, you can avoid the time factor as well. This is completely dependent on the nature of your work.
Now, we're gonna use our newly created function to start ice-cream production.
The result 👇 after 2 seconds looks like this:
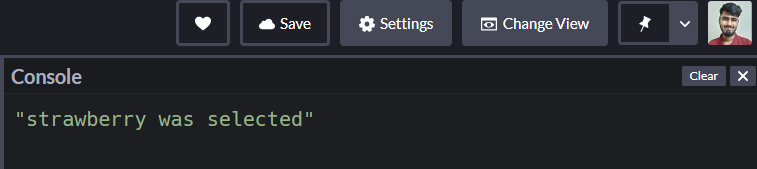
In this method, we defining what we need to do when the first task is complete using the .then handler. It looks something like this 👇
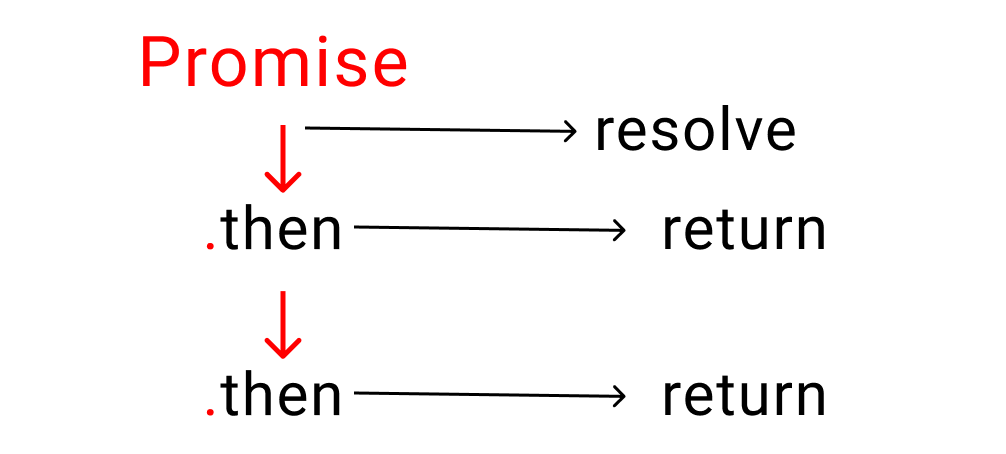
The .then handler returns a promise when our original promise is resolved.
Here's an Example:
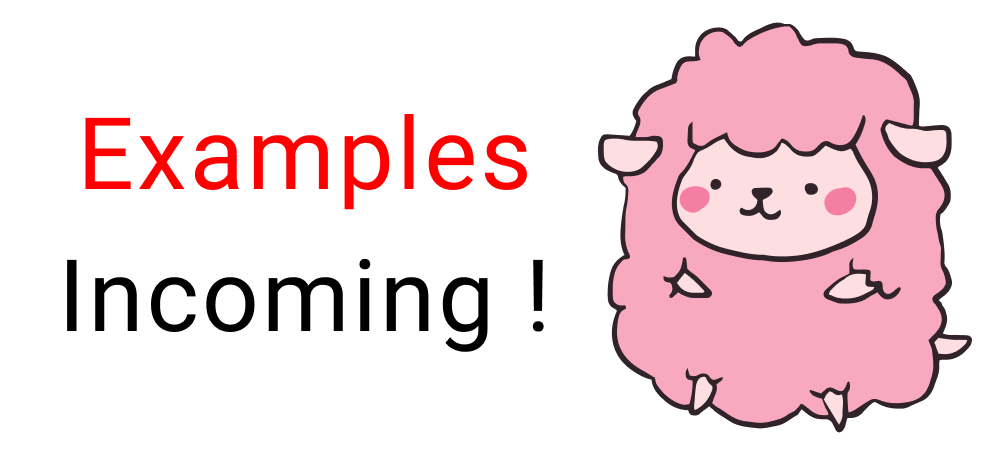
Let me make it simpler: it's similar to giving instructions to someone. You tell someone to " First do this, then do that, then this other thing, then.., then.., then..." and so on.
- The first task is our original promise.
- The rest of the tasks return our promise once one small bit of work is completed
Let's implement this on our project. At the bottom of your code write the following lines. 👇
Note: don't forget to write the return word inside your .then handler. Otherwise, it won't work properly. If you're curious, try removing the return once we finish the steps:
And here's the result: 👇
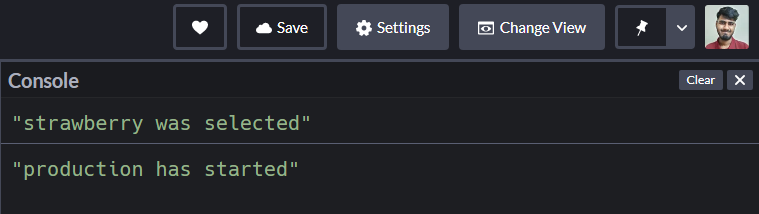
Using the same system, let's finish our project:👇
Here's the result: 👇
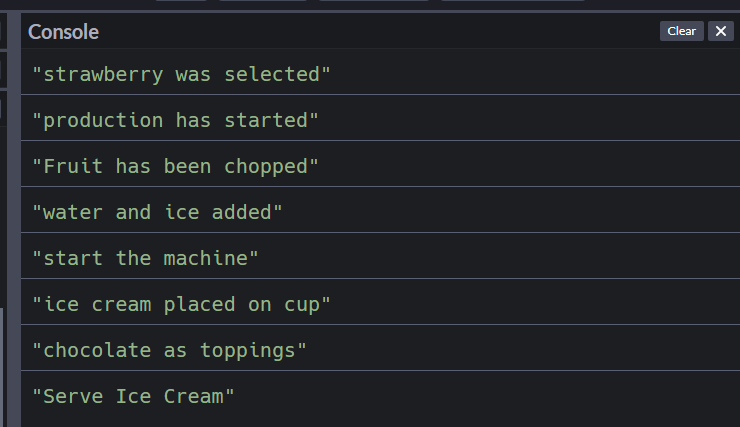
We need a way to handle errors when something goes wrong. But first, we need to understand the promise cycle:
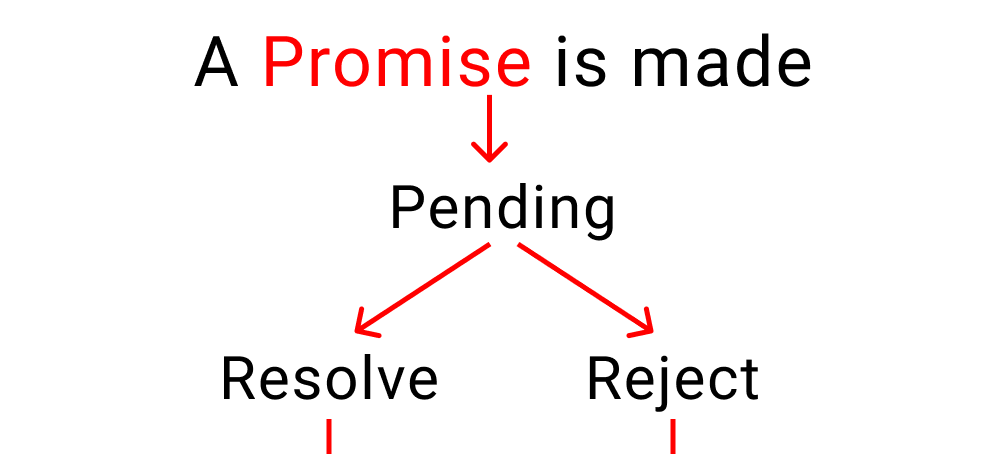
To catch our errors, let's change our variable to false.
Which means our shop is closed. We're not selling ice cream to our customers anymore.
To handle this, we use the .catch handler. Just like .then , it also returns a promise, but only when our original promise is rejected.
A small reminder here:
- .then works when a promise is resolved
- .catch works when a promise is rejected
Go down to the very bottom and write the following code:👇
Just remember that there should be nothing between your previous .then handler and the .catch handler.
Here's the result:👇
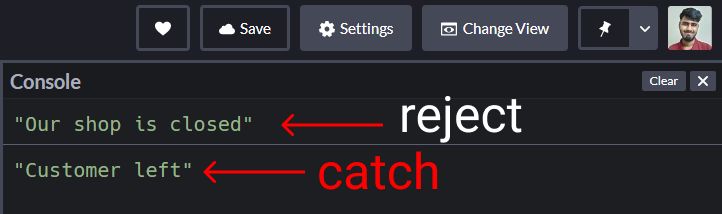
A couple things to note about this code:
- The 1st message is coming from the reject() part of our promise
- The 2nd message is coming from the .catch handler
How to use the .finally() handler
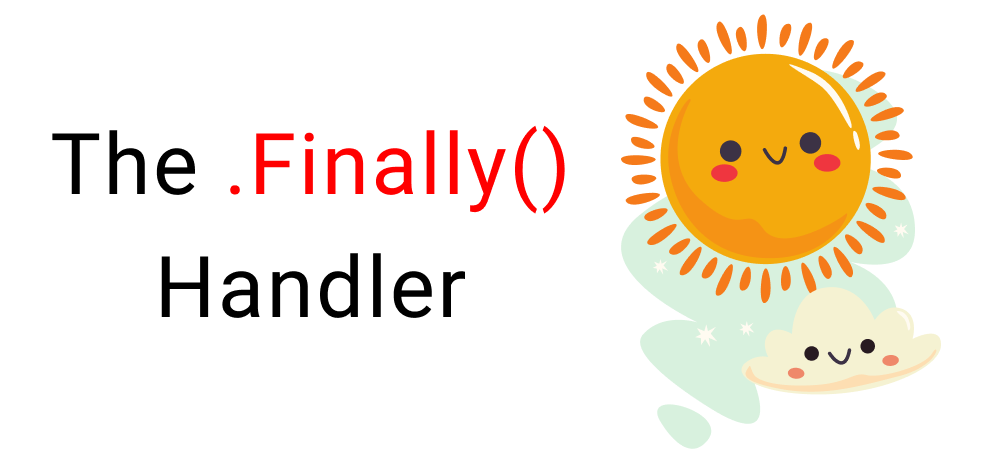
There's something called the finally handler which works regardless of whether our promise was resolved or rejected.
For example: whether we serve no customers or 100 customers, our shop will close at the end of the day
If you're curious to test this, come at very bottom and write this code: 👇
The result:👇
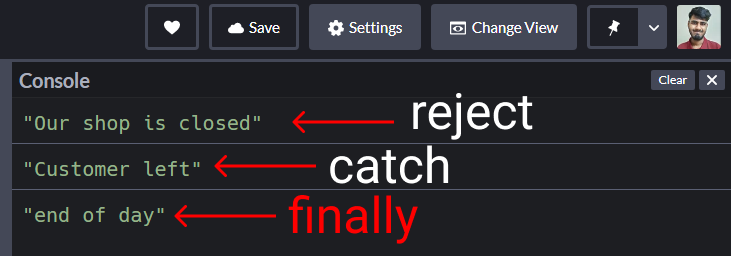
Everyone, please welcome Async / Await~
How Does Async / Await Work in JavaScript?
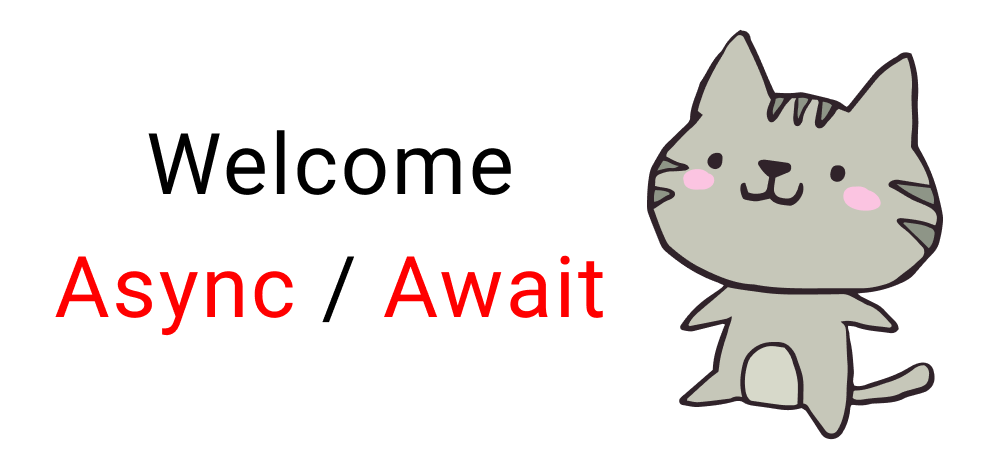
This is supposed to be the better way to write promises and it helps us keep our code simple and clean.
All you have to do is write the word async before any regular function and it becomes a promise.
But first, take a break
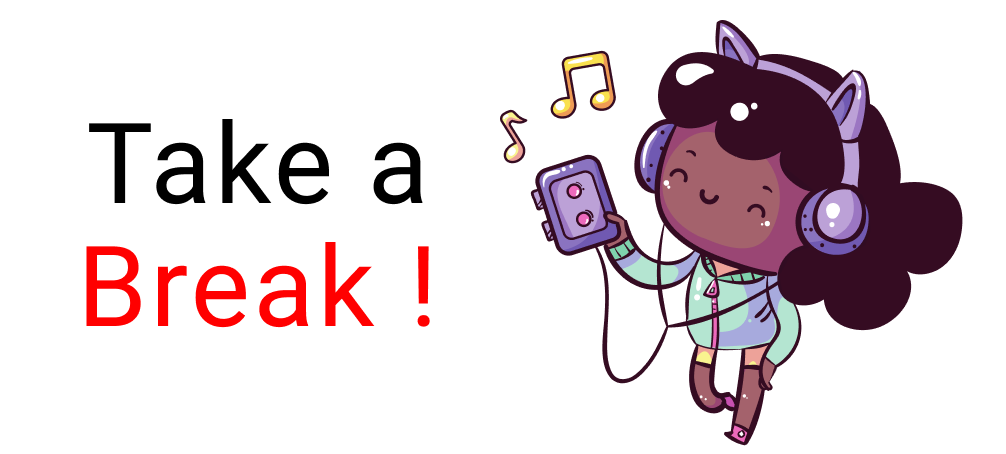
Let's have a look:👇
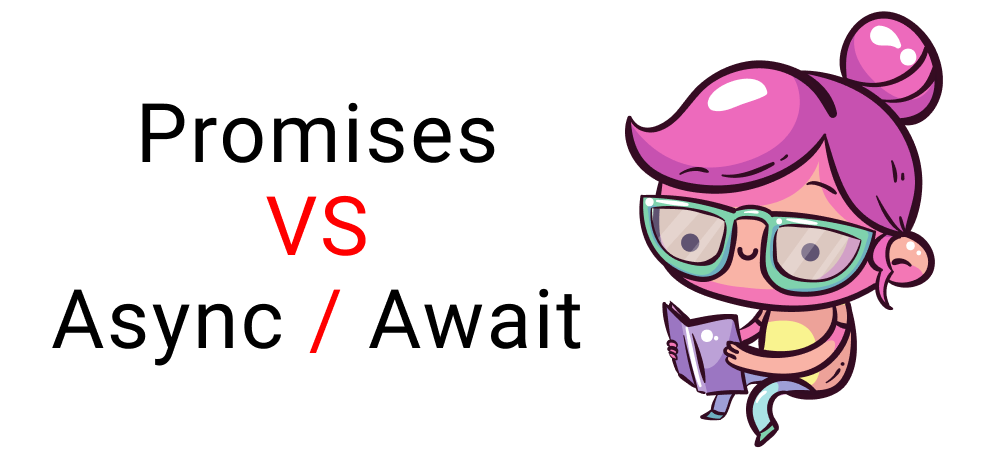
Promises vs Async/Await in JavaScript
Before async/await, to make a promise we wrote this:
Now using async/await, we write one like this:
But wait......
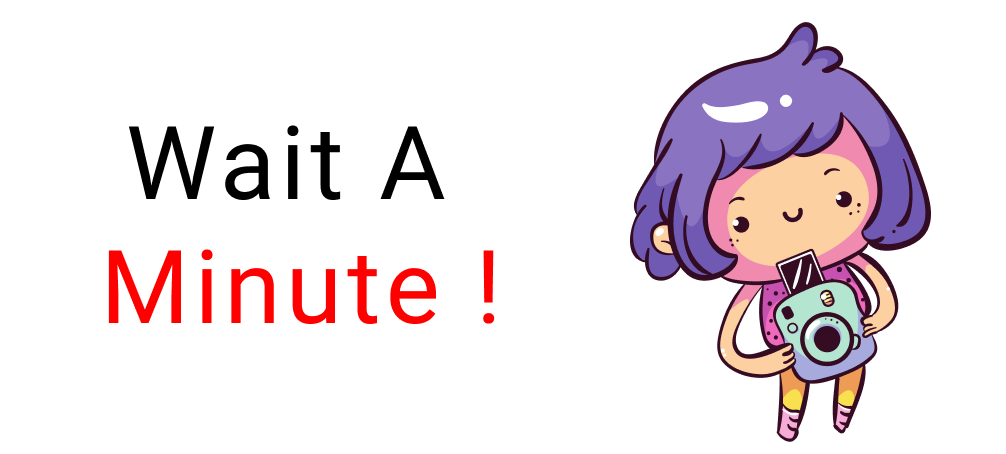
You need to understand ->
- How to use the try and catch keywords
- How to use the await keyword
How to use the Try and Catch keywords
We use the try keyword to run our code while we use catch to catch our errors. It's the same concept we saw when we looked at promises.
Let's see a comparison. We'll see a small demo of the format, then we'll start coding.
Promises in JS -> resolve or reject
We used resolve and reject in promises like this:
Async / Await in JS -> try, catch
When we're using async/await, we use this format:
Don't panic, we'll discuss the await keyword next.
Now hopefully you understand the difference between promises and Async / Await.
How to Use JavaScript's Await Keyword
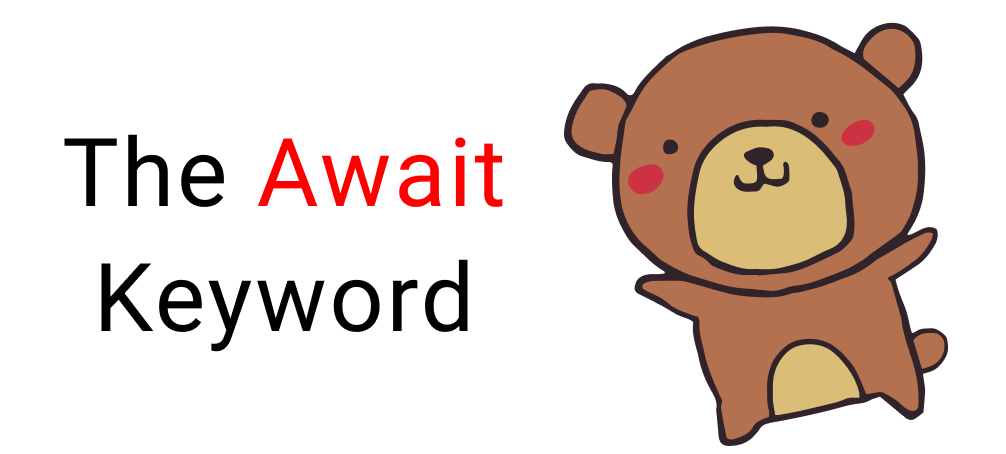
The keyword await makes JavaScript wait until a promise settles and returns its result.
How to use the await keyword in JavaScript
Let's go back to our ice cream shop. We don't know which topping a customer might prefer, chocolate or peanuts. So we need to stop our machine and go and ask our customer what they'd like on their ice cream.
Notice here that only our kitchen is stopped, but our staff outside the kitchen will still do things like:
- doing the dishes
- cleaning the tables
- taking orders, and so on.
An Await Keyword Code Example
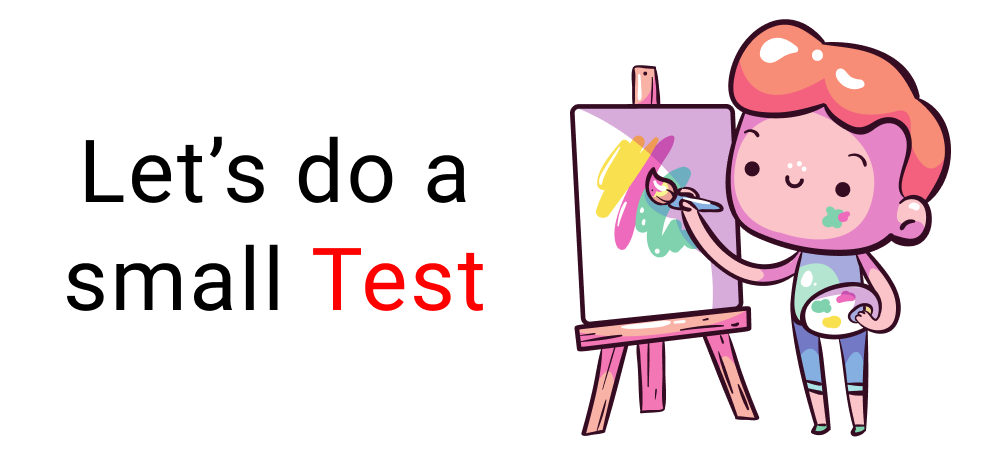
Let's create a small promise to ask which topping to use. The process takes three seconds.
Now, let's create our kitchen function with the async keyword first.
Let's add other tasks below the kitchen() call.
And here's the result:
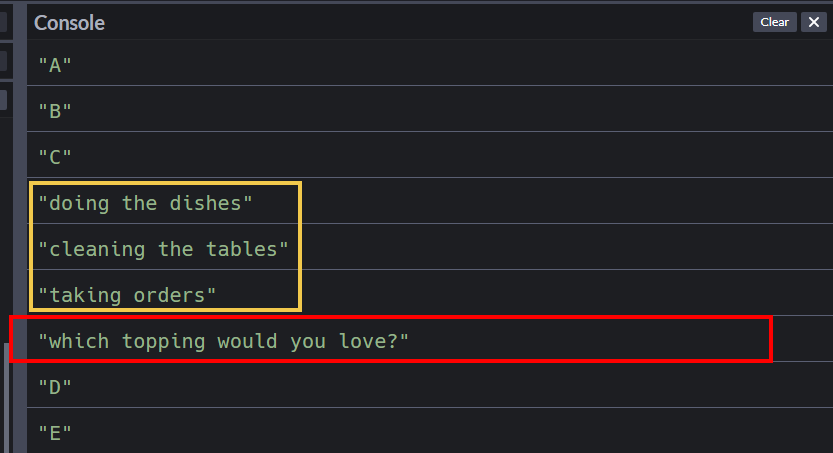
We are literally going outside our kitchen to ask our customer, "what is your topping choice?" In the mean time, other things still get done.
Once, we get their topping choice, we enter the kitchen and finish the job.
When using Async/ Await, you can also use the .then , .catch , and .finally handlers as well which are a core part of promises.
Let's open our Ice cream shop again
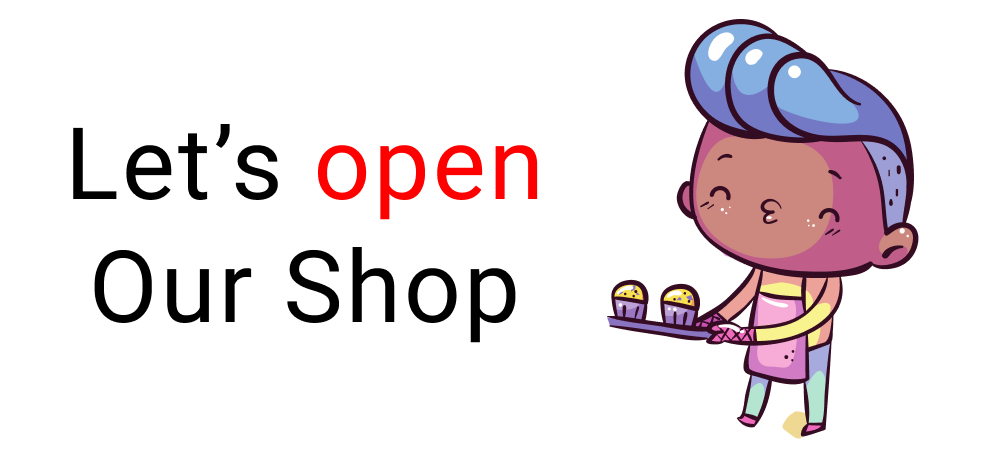
We're gonna create two functions ->
- kitchen : to make ice cream
- time : to assign the amount of time each small task will take.
Let's start! First, create the time function:
Now, let's create our kitchen:
Let's give small instructions and test if our kitchen function is working or not:
The result looks like this when the shop is open: 👇
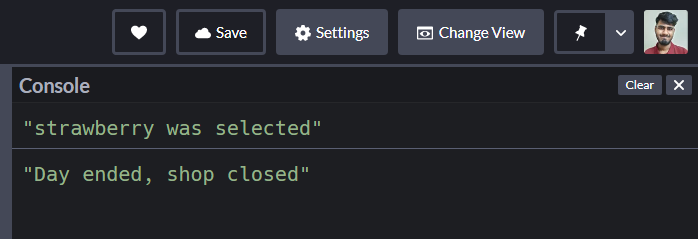
The result looks like this when the shop is closed: 👇
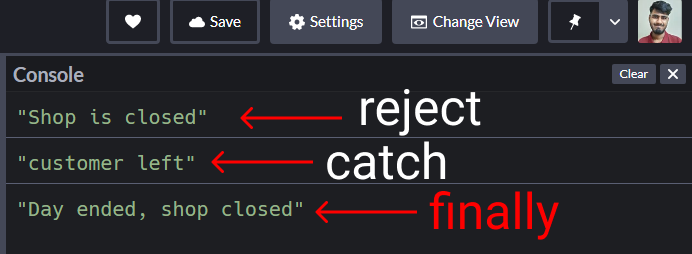
So far so good.
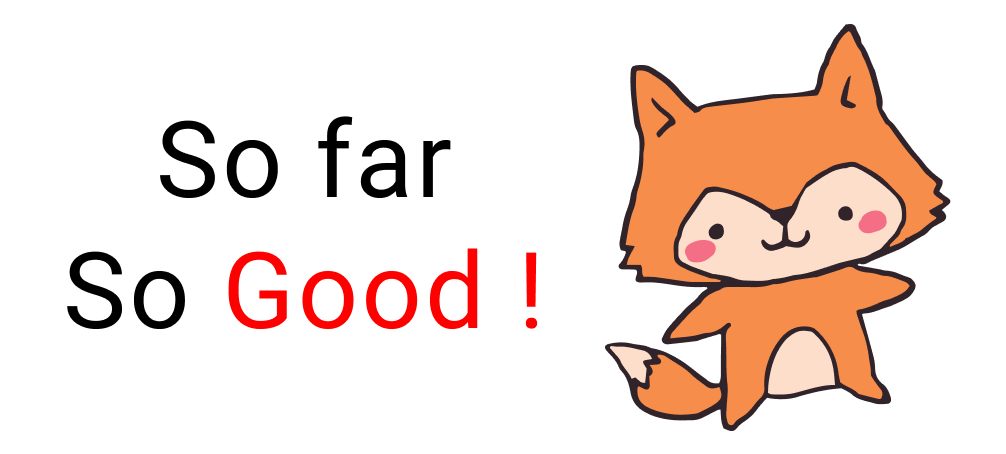
Let's complete our project.
Here's the list of our tasks again: 👇
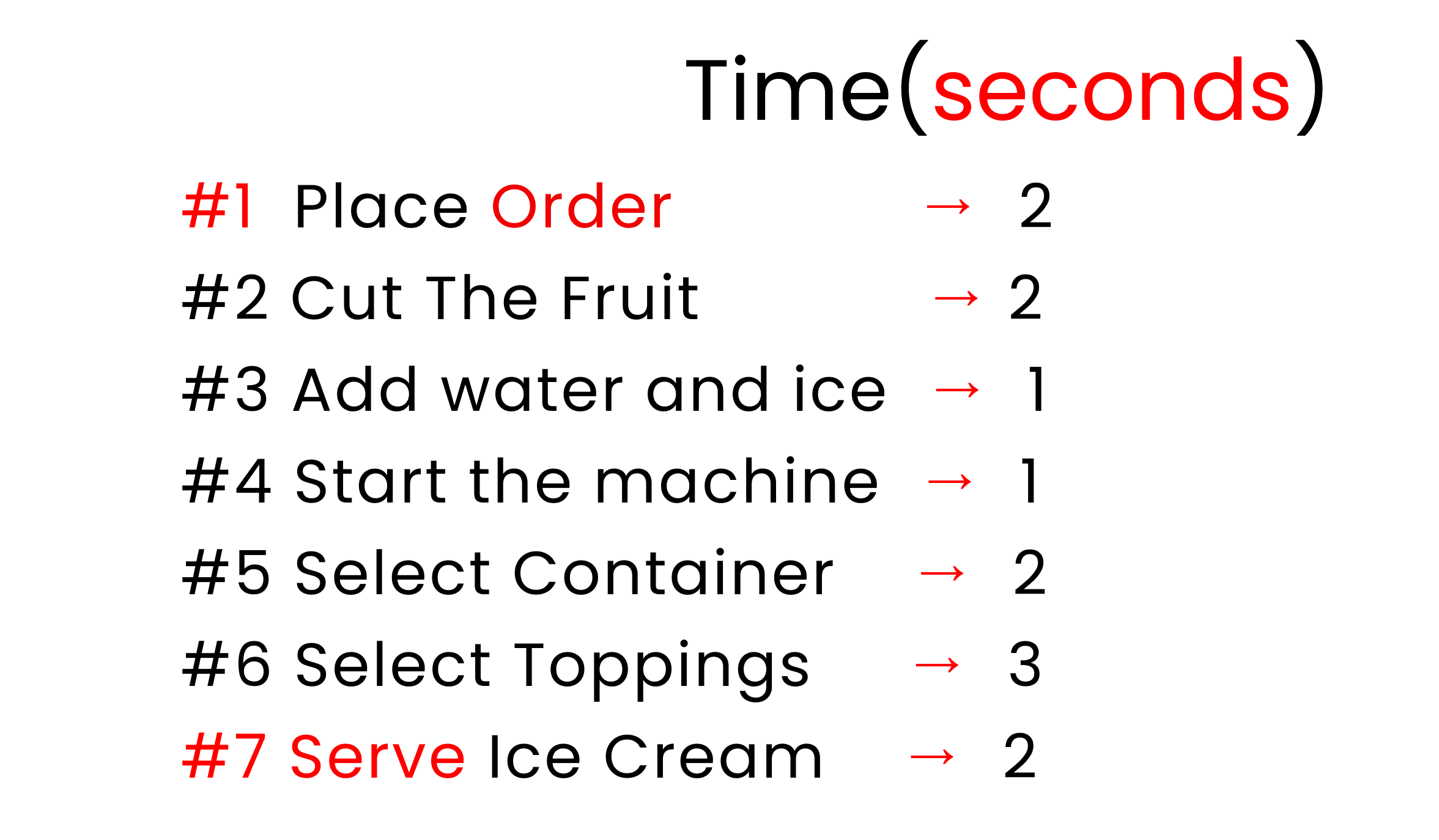
First, open our shop
Now write the steps inside our kitchen() function: 👇
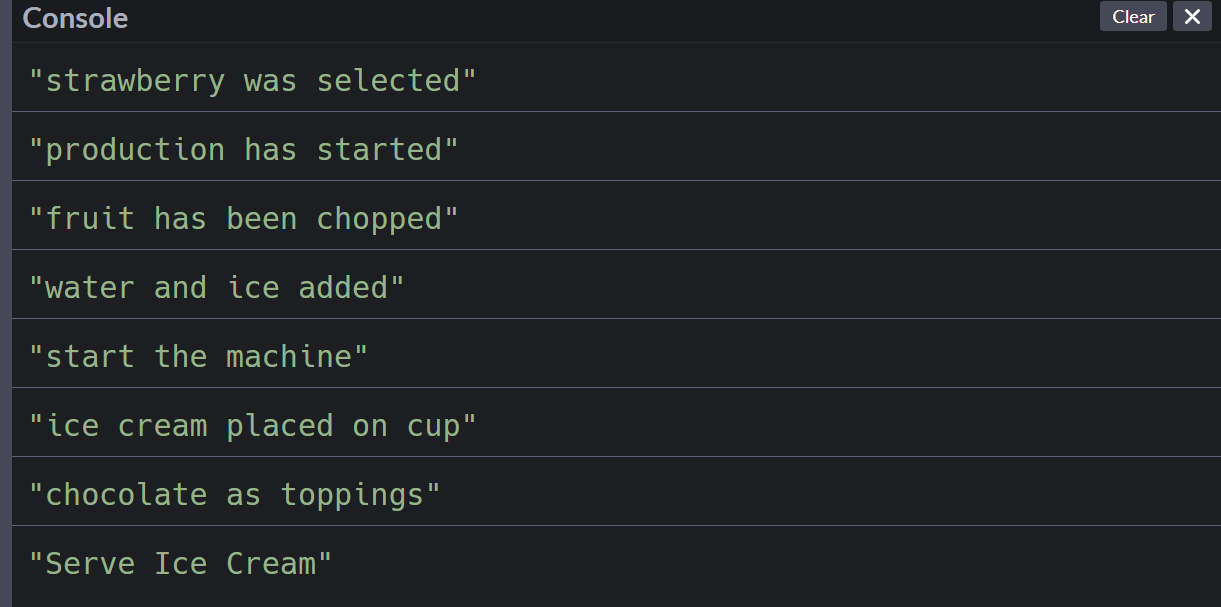
Congratulations for reading until the end! In this article you've learned:
- The difference between synchronous and asynchronous systems
- Mechanisms of asynchronous JavaScript using 3 techniques (callbacks, promises, and Async/ Await)
Here's your medal for reading until the end. ❤️
Suggestions and criticisms are highly appreciated ❤️
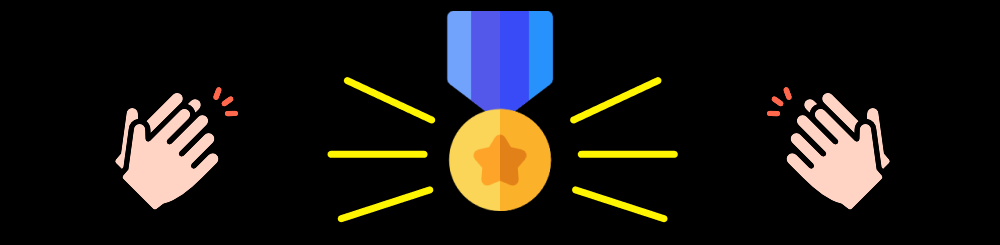
YouTube / Joy Shaheb
LinkedIn / JoyShaheb
Twitter / JoyShaheb
Instagram / JoyShaheb
- Collection of all the images used
- Unicorns , kitty avatar
- tabby cat , Astrologist Woman , girl-holding-flower
- Character emotions
Front end Developer 🔥 || Youtuber 🎬|| Blogger ✍|| K-pop fan ❤️️based in Dhaka, Bangladesh.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Data Science
- Trending Now
- Data Structures
- System Design
- Foundational Courses
- Practice Problem
- Machine Learning
- Data Science Using Python
- Web Development
- Web Browser
- Design Patterns
- Software Development
- Product Management
- Programming
Async/Await Function in JavaScript
In this video, we will explore the concept of async/await in JavaScript. async/await provides a more readable and convenient way to work with asynchronous code compared to traditional methods like callbacks and promises. This tutorial is perfect for students, professionals, or anyone interested in enhancing their JavaScript programming skills.
Why Learn About async/await?
Understanding how to use async/await helps to:
- Simplify asynchronous code and make it more readable.
- Avoid the pitfalls of callback hell.
- Handle asynchronous operations more efficiently.
Key Concepts
1. Asynchronous Programming:
- A programming paradigm that allows for non-blocking operations, enabling code execution to continue while waiting for operations like network requests, file reading, or timers to complete.
2. Promises:
- Objects representing the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises provide methods to handle asynchronous results.
3. async Function:
- A function declared with the async keyword. It automatically returns a promise and allows the use of the await keyword inside it.
4. await Keyword:
- Used inside an async function to pause the execution of the function until the promise is resolved or rejected. It simplifies the syntax for working with promises.
Steps to Use async/await
1. Declare an async Function:
- Use the async keyword before a function declaration or expression to create an async function.
2. Use await Inside the async Function:
- Use the await keyword to pause the execution of the async function until a promise is resolved.
3. Handle Errors with try...catch:
- Use try...catch blocks inside async functions to handle errors that occur during asynchronous operations.
Practical Examples
Example 1: Basic async/await Usage
Declare an async Function:
- Create an async function to fetch data from an API.
Use await to Wait for the Promise:
- Use await to wait for the API response and process the data.
Example 2: Handling Multiple Promises
- Create an async function to handle multiple asynchronous operations.
Use await for Multiple Promises:
- Use await to wait for multiple promises to resolve and process the results sequentially or concurrently.
Example 3: Error Handling with try...catch
- Create an async function with error-prone asynchronous operations.
Use try...catch for Error Handling:
- Wrap the await calls in a try...catch block to handle potential errors gracefully.
Practical Applications
Web Development:
- Use async/await to handle asynchronous operations such as fetching data from APIs, reading files, or performing background tasks.
Data Processing:
- Simplify asynchronous data processing tasks, such as reading and writing files, querying databases, or processing streams.
User Interface Interactions:
- Manage user interface interactions that involve asynchronous operations, such as loading content, handling form submissions, or updating the UI.
Additional Resources
For more detailed information and a comprehensive guide on async/await in JavaScript, check out the full article on GeeksforGeeks: https://www.geeksforgeeks.org/async-await-function-in-javascript/ . This article provides in-depth explanations, examples, and further readings to help you master the use of async/await.
By the end of this video, you’ll have a solid understanding of how to use async/await in JavaScript, enhancing your ability to write more readable and efficient asynchronous code.
Read the full article for more details: https://www.geeksforgeeks.org/async-await-function-in-javascript/ .
Thank you for watching!

- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Async / await assignment to object keys: is it concurrent?
I know that doing this:
Is effectively
Basically, a() runs then b() runs. I nested them to show that both resultA and resultB are in our scope; yet both function didn't run at once.
But what about this:
do a() and b() run concurrently?
For reference:
I know here funcA and funcB do run concurrently.
How would you represent the object assignment
using then / callbacks?
@Bergi is correct in this answer, this occurs sequentially. To share a nice solution for having this work concurrently for an object without having to piece together the object from an array, one can also use Bluebird as follows
http://bluebirdjs.com/docs/api/promise.props.html
- async-await
- es6-promise
- ecmascript-2017
- Your "for reference" example has a syntax error (and is misleading because of that). If you use await , you have to make the arrow function async , and then it becomes obvious that they might run concurrently because they're separate function evaluation. – Bergi Commented May 18, 2017 at 2:49
- Thanks for catching that, corrected. – Babakness Commented May 18, 2017 at 2:59
- 1 Note that the JS functions may trigger asynchronous processes that run concurrently, but the JS functions themselves aren't running concurrently in the sense of multi-threaded simultaneous execution. – nnnnnn Commented May 18, 2017 at 3:38
2 Answers 2
No, every await will stop the execution until the promise has fulfilled, even mid-expression. It doesn't matter whether they happen to be part of the same statement or not.
If you want to run them in parallel, and wait only once for their result, you have to use await Promise.all(…) . In your case you'd write
How would you represent the object assignment using then / callbacks?
With temporary variables for each awaited value. Every await translates into one then call:
If we wanted to be pedantic, we'd have to pick apart the object creation:
- You're right! I just finished writing test code to try the above code using setTimeout and it does indeed run sequentially. – Babakness Commented May 18, 2017 at 3:07
No, they run sequentially.
The equivalent would be something like

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged javascript async-await es6-promise ecmascript-2017 or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- From PHP to JavaScript to Kubernetes: how one backend engineer evolved over time
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- Can a "sharp turn" on a trace with an SMD resistor also present a risk of reflection?
- Should I Inform My PhD Program About Not Completing My Master's Degree Due to Personal Challenges?
- Can the speed of light inhibit the synchronisation of a power grid?
- How to remove a file which name seems to be "." on an SMB share?
- Why don't we observe protons deflecting in J.J. Thomson's experiment?
- Four out puzzle: Get rid of a six digit number in 4 moves
- Highlight shortest path between two clickable graph vertices
- How should I respond to a former student from my old institution asking for a reference?
- Why is global state hard to test? Doesn't setting the global state at the beginning of each test solve the problem?
- Calculate the sum of numbers in a rectangle
- If you get pulled for secondary inspection at immigration, missing flight, will the airline rebook you?
- Is it OK to use the same field in the database to store both a percentage rate and a fixed money fee?
- Looking for source of story about returning a lost object
- Makeindex lost some pages when using custom schem
- In the US, can I buy iPhone and Android phones and claim them as expense?
- Rate of change of surface area is acting weird
- What is the default font of report documentclass?
- Is there anything that stops the majority shareholder(s) from destroying company value?
- Meaning of capacitor "x2" symbol on data sheet schematic
- How can I push back on my co-worker's changes that I disagree with?
- bash script quoting frustration
- Everyone hates this Key Account Manager, but company won’t act
- SF novel where the story, or part of it, is narrated by two linked brains taking turns
- Who said "If you don't do politics, politics will do you"?
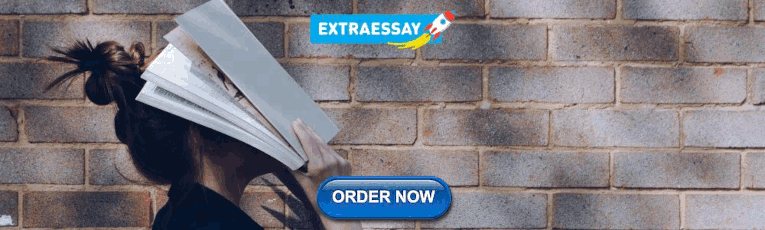
IMAGES
COMMENTS
Description. await is usually used to unwrap promises by passing a Promise as the expression. Using await pauses the execution of its surrounding async function until the promise is settled (that is, fulfilled or rejected). When execution resumes, the value of the await expression becomes that of the fulfilled promise.
In order to make a call to a function like await getContacts(){} you need to have that code wrapped in an asynchronous function that is defined like: async function wrapperFunction() {. const contacts = await getContacts() console.log('body: ') console.log(contacts)
Like promise.then, await allows us to use thenable objects (those with a callable then method). The idea is that a third-party object may not be a promise, but promise-compatible: if it supports .then, that's enough to use it with await. Here's a demo Thenable class; the await below accepts its instances:
The Async Keyword. To create an asynchronous function, you need to add the async keyword before your function name. Take a look at line 1 in the example below: console.log(json) } runProcess(); Here, we created an asynchronous function called runProcess() and put the code that uses the await keyword inside it.
Description. An async function declaration creates an AsyncFunction object. Each time when an async function is called, it returns a new Promise which will be resolved with the value returned by the async function, or rejected with an exception uncaught within the async function. Async functions can contain zero or more await expressions.
In the above example, getMovies() at the (*) line is waiting for createMovies() to be executed in the async function. In other words, createMovies() is async, so getMovies() will only run after createMovies() is done. Now you know all the basics of Event Loops, Callbacks, Promises and Async/Await.
Asynchronous Awaits in Synchronous Loops. At some point, we'll try calling an asynchronous function inside a synchronous loop. For example: // Return promise which resolves after specified no ...
Summary: in this tutorial, you will learn how to write asynchronous code using JavaScript async/ await keywords.. Note that to understand how the async / await works, you need to know how promises work.. Introduction to JavaScript async / await keywords. In the past, to handle asynchronous operations, you used the callback functions.However, nesting many callback functions can make your code ...
freeCodeCamp is a donor-supported tax-exempt 501(c)(3) charity organization (United States Federal Tax Identification Number: 82-0779546) Our mission: to help people learn to code for free.
To use async/await in JavaScript, we need to declare an async function. The async keyword is used to declare an async function. Inside this function, we can use the await keyword. The await keyword is used to wait for a promise to resolve or reject. Note: The await keyword can only be used inside an async function. Let's see an example of an ...
variable. Receives a value from the sequence on each iteration. May be either a declaration with const, let, or var, or an assignment target (e.g. a previously declared variable, an object property, or a destructuring assignment pattern).Variables declared with var are not local to the loop, i.e. they are in the same scope the for await...of loop is in.
JavaScript await Keyword. The await keyword is used inside the async function to wait for the asynchronous operation. The syntax to use await is: let result = await promise; The use of await pauses the async function until the promise returns a result (resolve or reject) value.
Let's use await. As in, "Hey engine. This function is asynchronous and returns a promise. Instead of continuing on like you typically do, go ahead and 'await' the eventual value of the promise and return it before continuing". With both of our new async and await keywords in play, our new code will look like this. $
The async keyword is what lets the JavaScript engine know that you are declaring an asynchronous function. This is required to use await inside any function. ... Do the assignments below, and dive deeper into the understanding of async/await. Assignment. Read this Async and Await article for a solid introduction to async/await.
Browser Support. ECMAScript 2017 introduced the JavaScript keywords async and await. The following table defines the first browser version with full support for both: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The keyword await makes JavaScript wait until a promise settles and returns its result. How to use the await keyword in JavaScript. Let's go back to our ice cream shop. We don't know which topping a customer might prefer, chocolate or peanuts. ... time: to assign the amount of time each small task will take. Let's start! First, create the time ...
Async and Await in JavaScript is used to simplify handling asynchronous operations using promises. By enabling asynchronous code to appear synchronous, they enhance code readability and make it easier to manage complex asynchronous flows. Async Function. The async function allows us to write promise-based code as if it were synchronous. This ...
JavaScript interpreters are single threaded, so a variable can never change, when the code is waiting in some other code that does not change the variable. ... The solution can be run on modern browsers that support Promise and async/await. If you are using Node.js, consider EventEmitter as a better solution. <!-- This div is the trick ...
Steps to Use async/await. 1. Declare an async Function: Use the async keyword before a function declaration or expression to create an async function. 2. Use await Inside the async Function: Use the await keyword to pause the execution of the async function until a promise is resolved. 3.
Using async/await allows us to pause the operations within the async function until the Promise being await'd has moved from the Pending state to the either the Fulfilled or Rejected state. So in your first example, an assignment statement with an await pauses until the state changes. If the Promise is fulfilled, the left hand side of the ...
For reference: const asyncFunc = async (func) => await func.call() const results = [funcA,funcB].map( asyncFunc ) I know here funcA and funcB do run concurrently. Bonus: How would you represent the object assignment. const obj = {.