Editions Skylight Publishing [email protected] Got any suggestions?We want to hear from you! Send us a message and help improve Slidesgo Top searches Trending searches 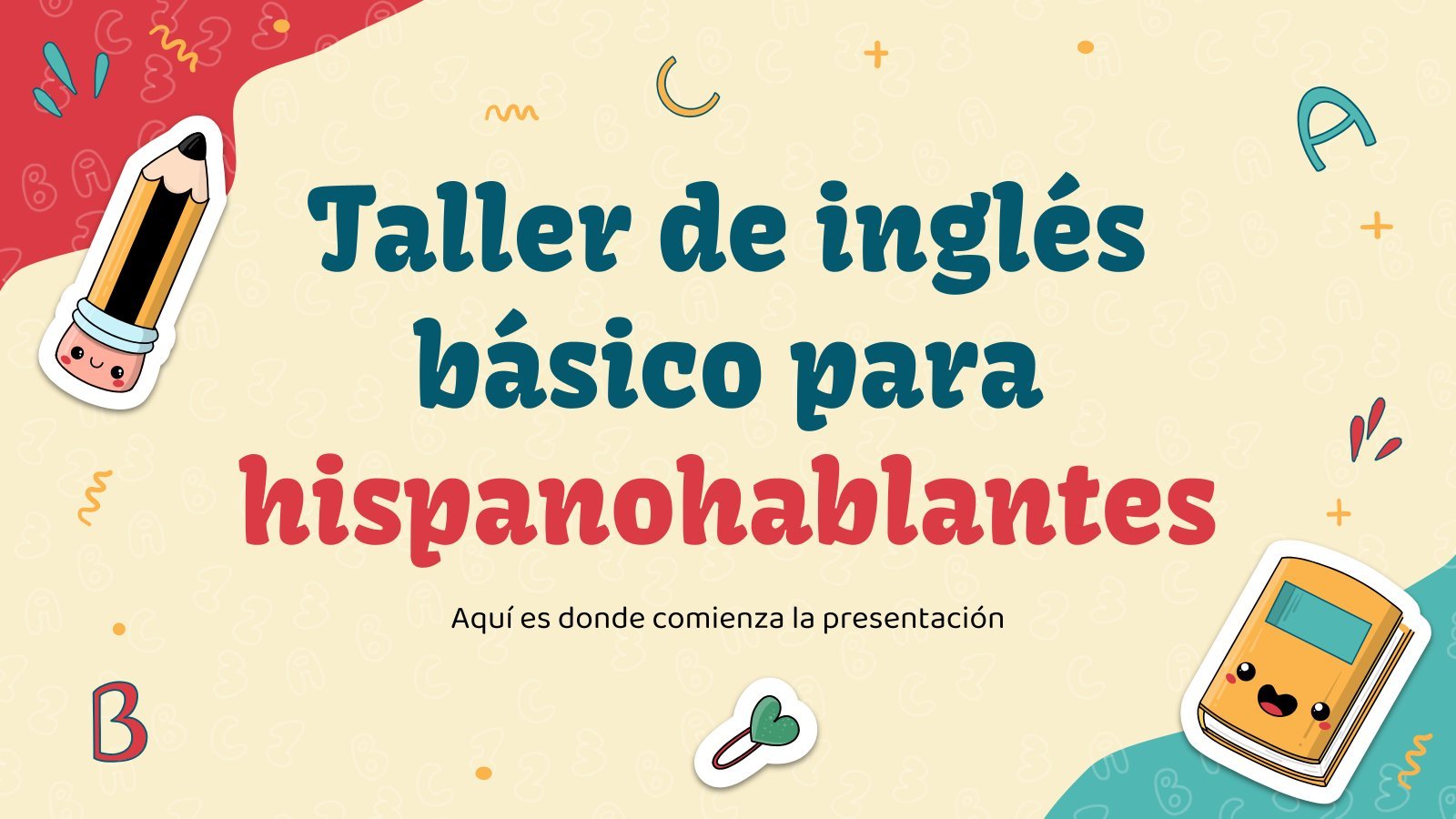 49 templates 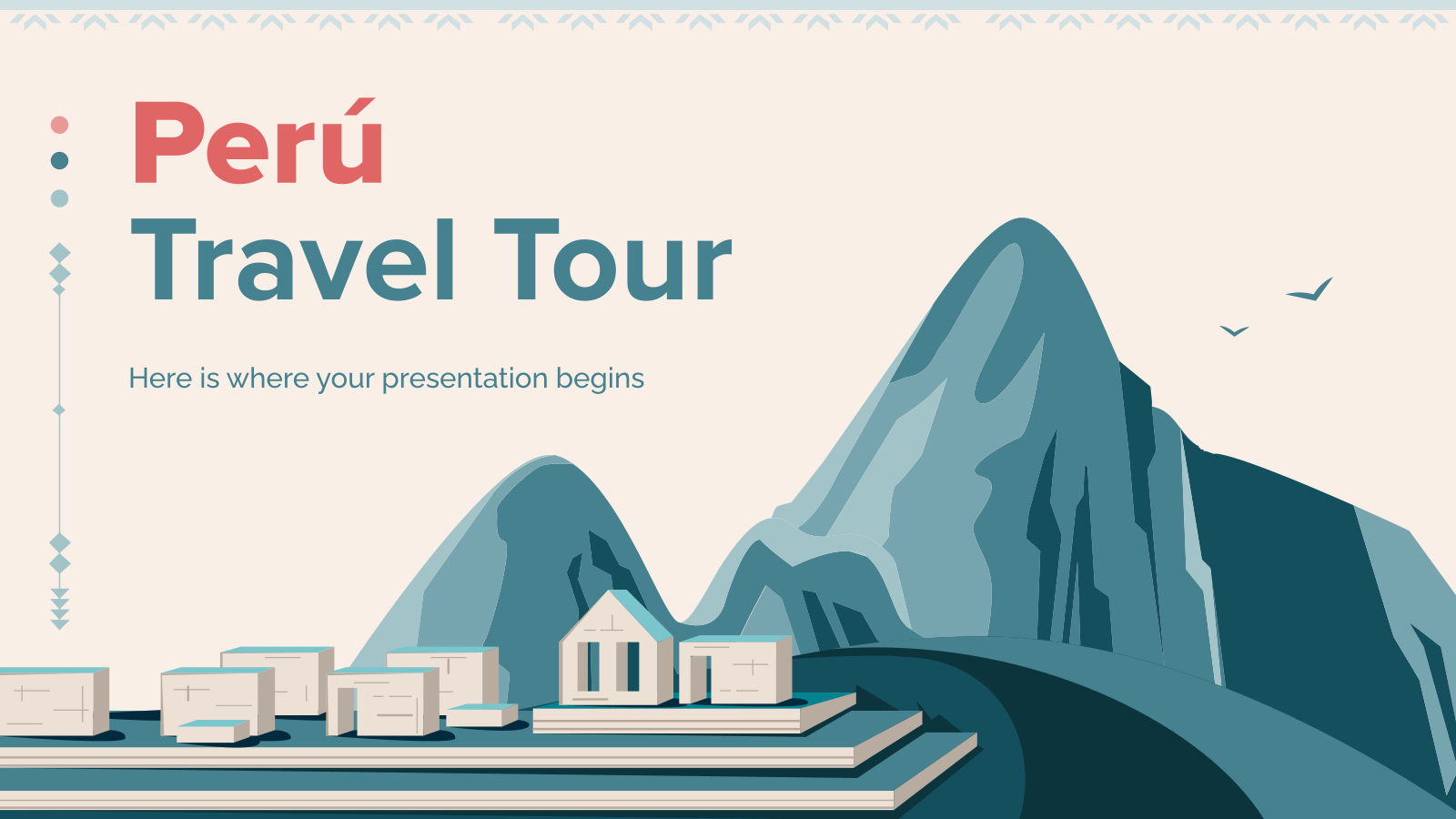 44 templates  61 templates 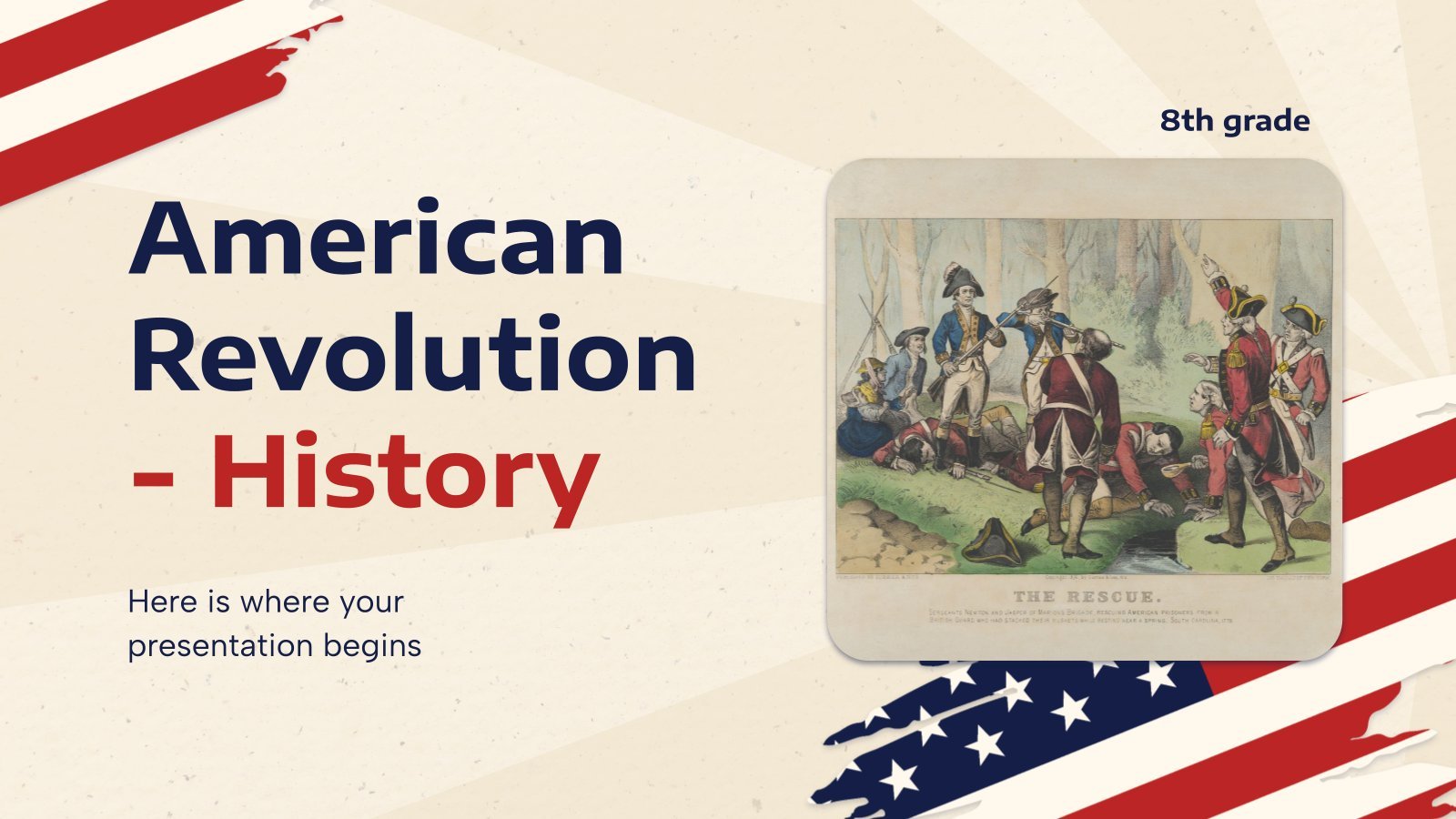 american history85 templates 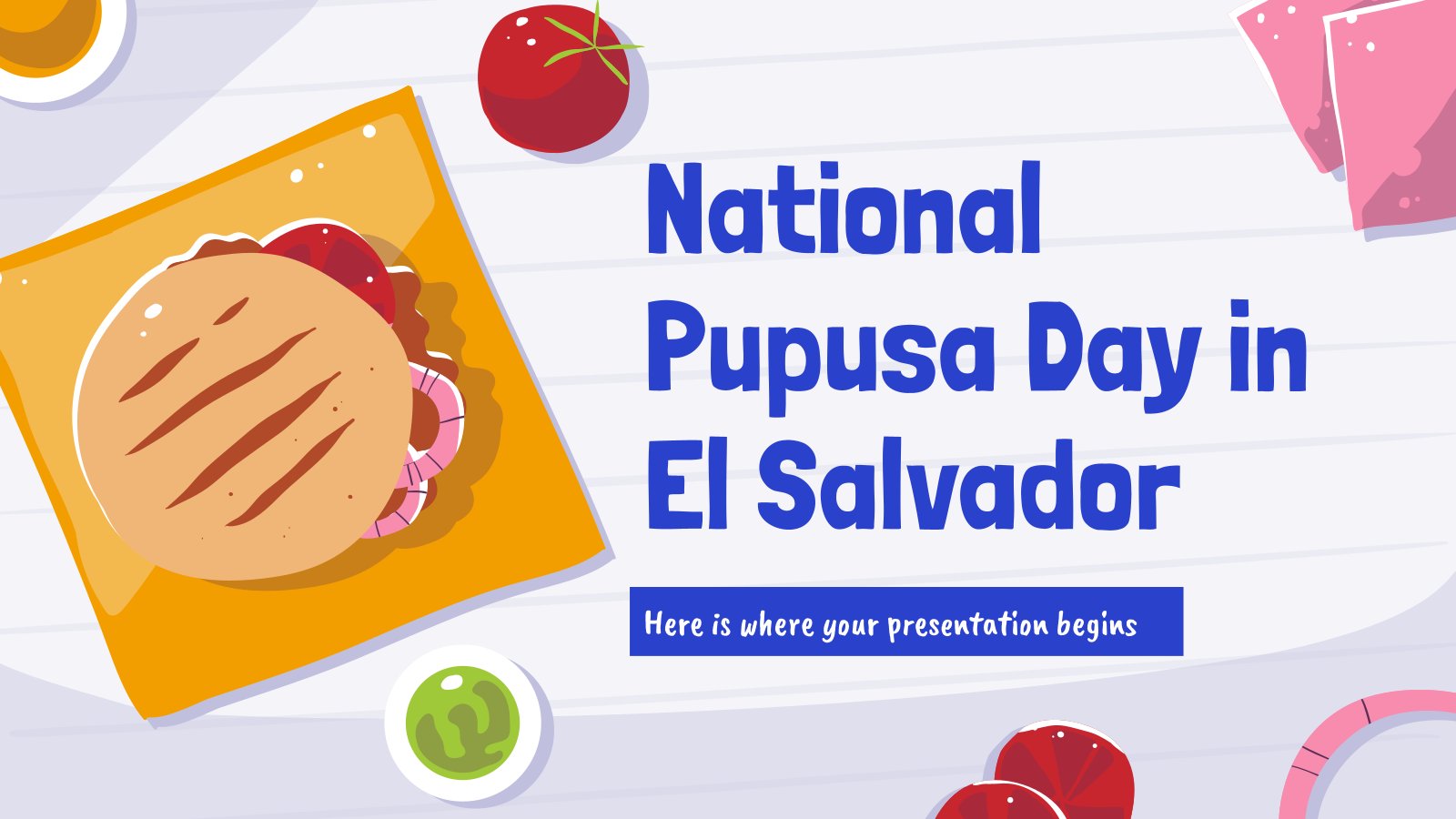 el salvador34 templates 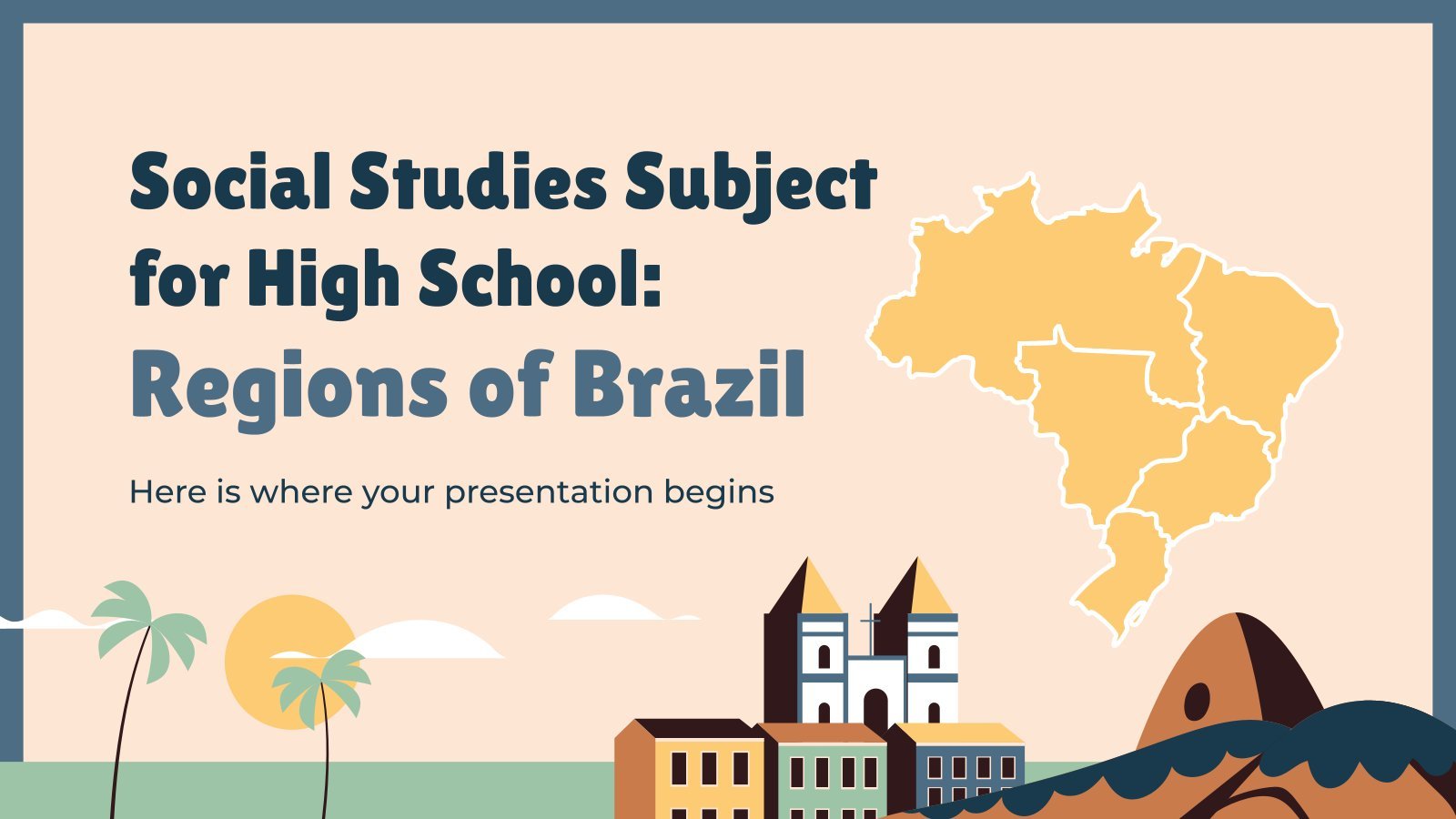 63 templates Java Programming WorkshopIt seems that you like this template, java programming workshop presentation, free google slides theme, powerpoint template, and canva presentation template. Programming... it's hard, it must be said! It won't be after you use this presentation! If you are an expert in Java and programming, share your knowledge in the form of a workshop. This template is designed for you to include everything you know about Java and show it to other interested people. The slides feature black backgrounds decorated with gradient lines of pink, blue, and purple — which by words can mean nothing, but if you look at it, it looks like any programming language! Don't wait any longer to download this template and prepare your workshop! People need your knowledge! Features of this template- 100% editable and easy to modify
- 32 different slides to impress your audience
- Contains easy-to-edit graphics such as graphs, maps, tables, timelines and mockups
- Includes 500+ icons and Flaticon’s extension for customizing your slides
- Designed to be used in Google Slides, Canva, and Microsoft PowerPoint
- 16:9 widescreen format suitable for all types of screens
- Includes information about fonts, colors, and credits of the resources used
How can I use the template? Am I free to use the templates? How to attribute? Attribution required If you are a free user, you must attribute Slidesgo by keeping the slide where the credits appear. How to attribute?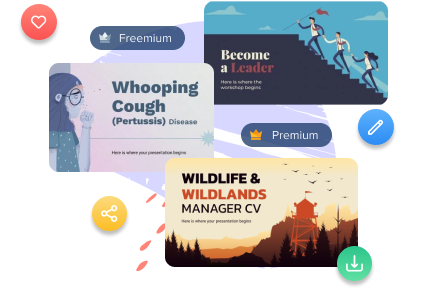 Register for free and start downloading nowRelated posts on our blog. 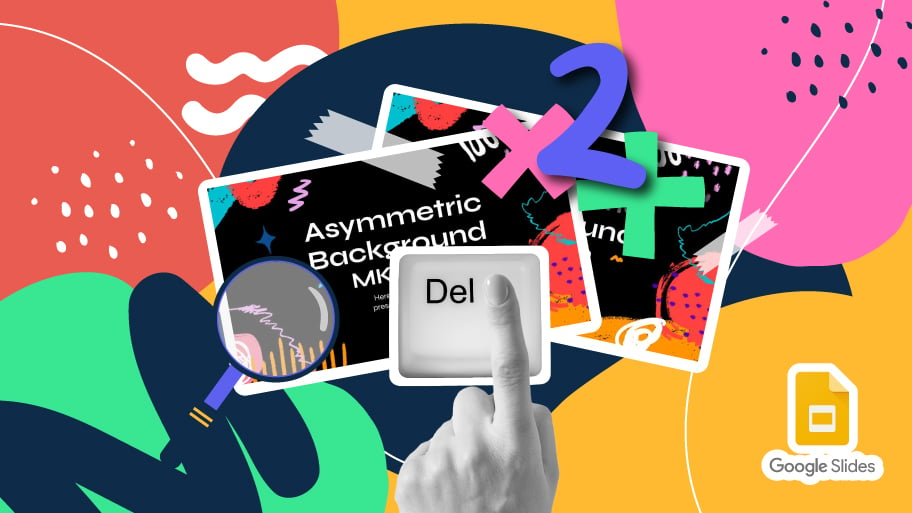 How to Add, Duplicate, Move, Delete or Hide Slides in Google Slides How to Change Layouts in PowerPoint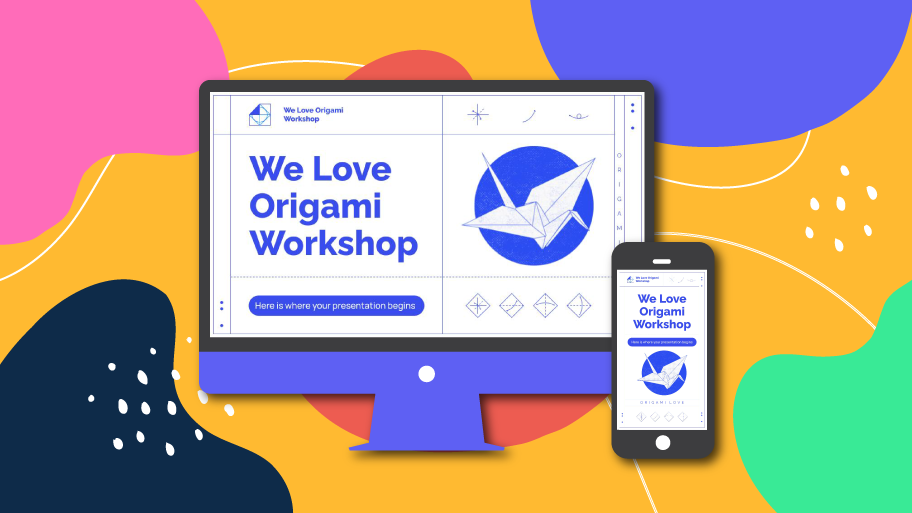 How to Change the Slide Size in Google SlidesRelated presentations. 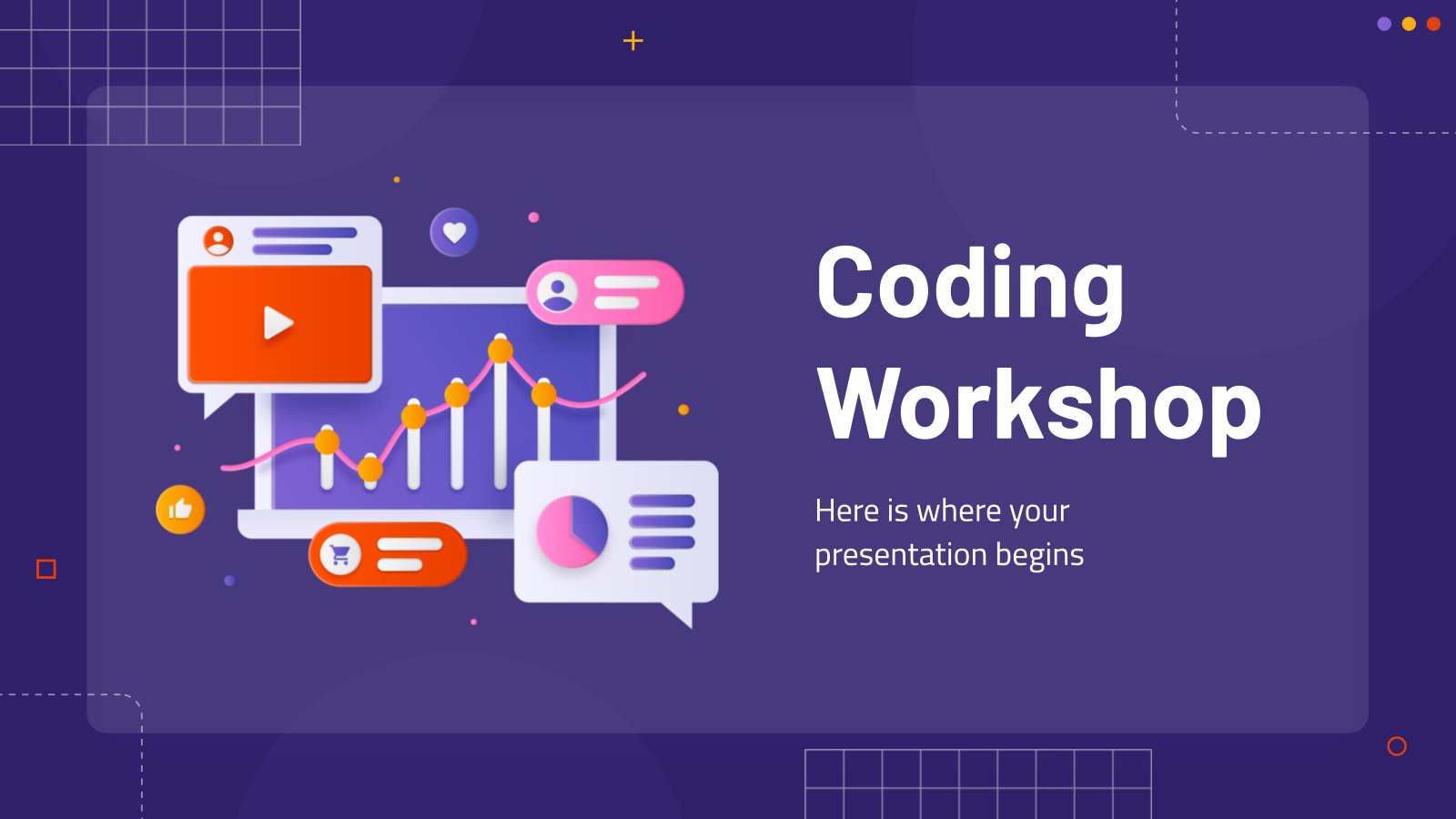 Premium templateUnlock this template and gain unlimited access 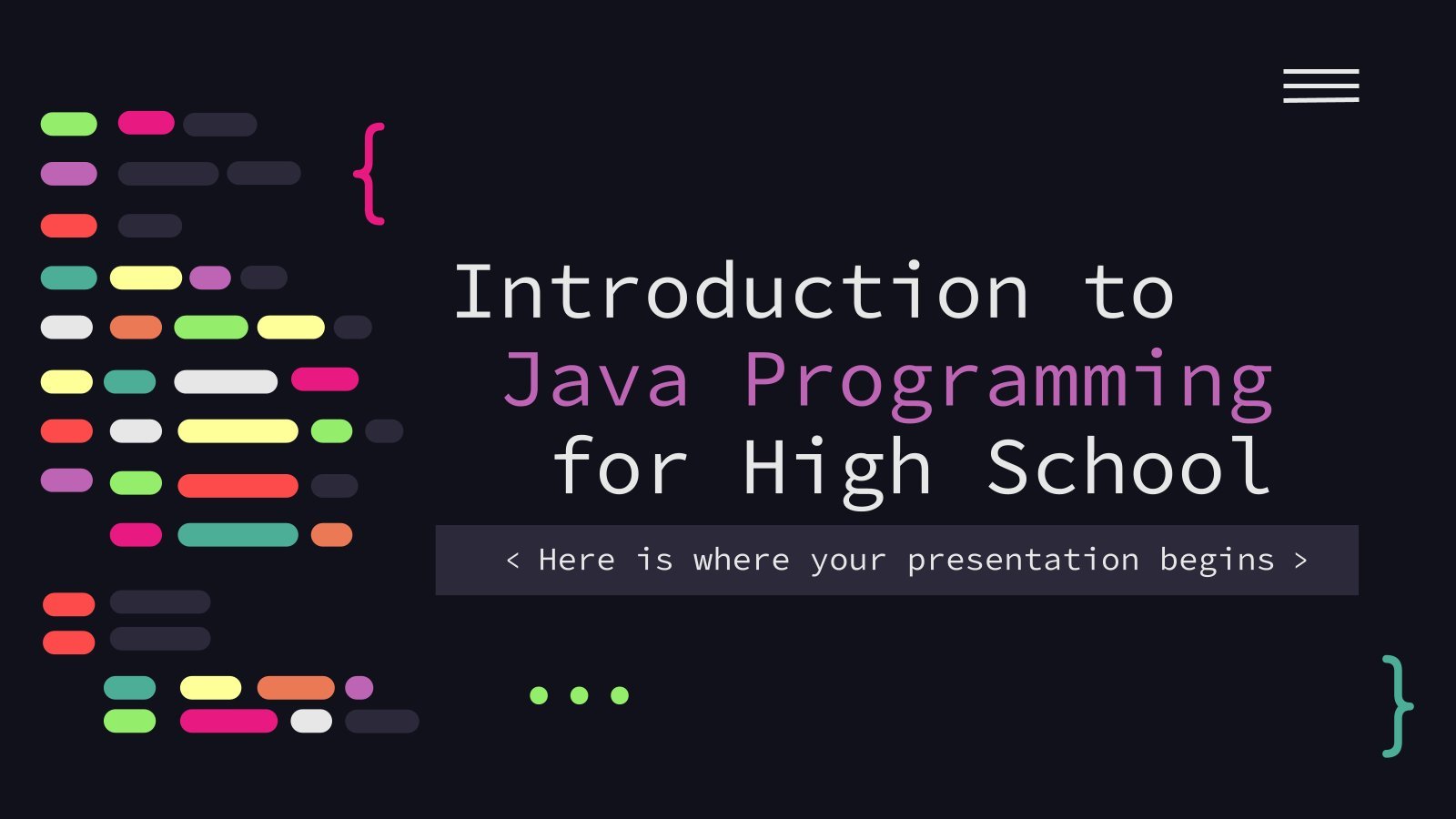 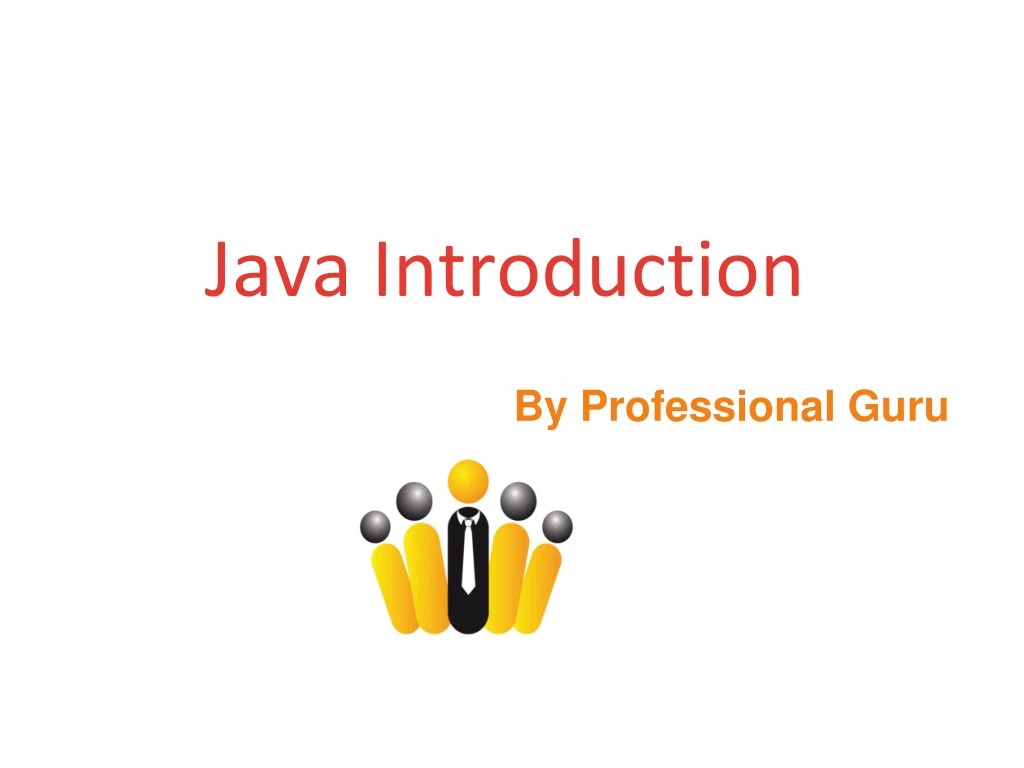 Introduction to JAVAAug 22, 2018 580 likes | 962 Views JAVA was developed by Sun Microsystems Inc in 1991, later acquired by Oracle Corporation. It was developed by James Gosling and Patrick Naughton. It is a simple programming language. Writing, compiling and debugging a program is easy in java. It helps to create modular programs and reusable code. Share Presentation- object oriented
- exception handling
- object oriented language
- java adds labeled break
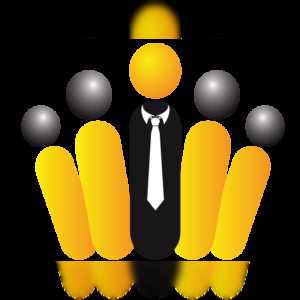 Presentation TranscriptJava Introduction By Professional Guru Introduction • Welcome to the course Object Oriented Programming in JAVA. This course will cover a core set of computer science concepts needed to create a modern software application using Java. http://professional-guru.com Course Objectives On completion of this course we will be able to: 1. 2. 3. 4. 5. Identify the importance of Java . Identify the additional features of Java compared to C++ . Identify the difference between Compiler and Interpreter . Identify the difference between applet and application . Apply Object Oriented Principles of Encapsulations, Data abstraction, Inheritance, Polymorphism. Program using java API (Application Programming Interface). Program using Exception Handling, Files and Threads . Program Using applets and swings . 6. 7. 8. http://professional-guru.com Course Syllabus UNIT CONCEPTS to be covered UNIT-I JAVA Basics UNIT-II Inheritance UNIT-III Data structures creation and manipulation in java UNIT-IV Exception Handling UNIT-V GUI Programming With JAVA http://professional-guru.com JAVA Basics http://professional-guru.com Why Java is Important • Two reasons : – Trouble with C/C++ language is that they are not portable and are not platform independent languages. – Emergence of World Wide Web, which demanded portable programs • Portability and security necessitated the invention of Java http://professional-guru.com History • James Gosling - Sun Microsystems • Co founder – Vinod Khosla • Oak - Java, May 20, 1995, Sun World • JDK Evolutions – JDK 1.0 (January 23, 1996) – JDK 1.1 (February 19, 1997) – J2SE 1.2 (December 8, 1998) – J2SE 1.3 (May 8, 2000) – J2SE 1.4 (February 6, 2002) – J2SE 5.0 (September 30, 2004) – Java SE 6 (December 11, 2006) – Java SE 7 (July 28, 2011) http://professional-guru.com Cont.. • Java Editions. J2SE(Java 2 Standard Edition) - to develop client-side standalone applications or applets. J2ME(Java 2 Micro Edition ) - to develop applications for mobile devices such as cell phones. J2EE(Java 2 Enterprise Edition ) - to develop server-side applications such as Java servlets and Java ServerPages. http://professional-guru.com What is java? • A general-purpose object-oriented language. • Write Once Run Anywhere (WORA). • Designed for easy Web/Internet applications. • Widespread acceptance. http://professional-guru.com How is Java different from C… • C Language: – Major difference is that C is a structure oriented language and Java is an object oriented language and has mechanism to define classes and objects. – Java does not support an explicit pointer type – Java does not have preprocessor, so we cant use #define, #include and #ifdef statements. – Java does not include structures, unions and enum data types. – Java does not include keywords like goto, sizeof and typedef. – Java adds labeled break and continue statements. – Java adds many features programming. required for object oriented http://professional-guru.com How is Java different from C++… • C++ language Features removed in java: Java doesn’t support pointers to avoid unauthorized access of memory locations. Java does not include structures, unions and enum data types. Java does not support operator over loading. Preprocessor plays less important role in C++ and so eliminated entirely in java. Java does not perform automatic type conversions that result in loss of precision. http://professional-guru.com Cont… Java does not support global variables. Every method and variable is declared within a class and forms part of that class. Java does not allow default arguments. Java does not support inheritance of multiple super classes by a sub class (i.e., multiple inheritance). This is accomplished by using ‘interface’ concept. It is not possible to declare unsigned integers in java. In java objects are passed by reference only. In C++ objects may be passed by value or reference. http://professional-guru.com Cont … New features added in Java: Multithreading, that allows two or more pieces of the same program to execute concurrently. C++ has a set of library functions that use a common header file. But java replaces it with its own set of API classes. It adds packages and interfaces. Java supports automatic garbage collection. break and continue statements have been enhanced in java to accept labels as targets. The use of unicode characters ensures portability. http://professional-guru.com Cont … Features that differ: Though C++ and java supports Boolean data type, C++ takes any nonzero value as true and zero as false. True and false in java are predefined literals that are values for a boolean expression. Java has replaced the destructor function with a finalize() function. C++ supports exception handling that is similar to java's. However, in C++ there is no requirement that a thrown exception be caught. http://professional-guru.com Characteristics of Java • Java is simple • Java is architecture-neutral • Java is object-oriented • Java is portable • Java is distributed • Java’s performance • Java is interpreted • Java is multithreaded • Java is robust • Java is dynamic • Java is secure http://professional-guru.com Java Environment • Java includes many development tools, classes and methods – Development tools are part of Java Development Kit (JDK) and – The classes and methods are part of Java Standard Library (JSL), also known as Application Programming Interface (API). • JDK constitutes of tools like java compiler, java interpreter and many. • API includes hundreds of classes and methods grouped into several packages according to their functionality. http://professional-guru.com Java is architecture-neutral JAVA Program Execution http://professional-guru.com WORA(Write Once Run Anywhere) http://professional-guru.com Editplus for Java Programming • Edit Plus Software: • EditPlus is a 32-bit text editor for the Microsoft Windows operating system. • The editor contains tools for programmers, including syntax highlighting (and support for custom syntax files), file type conversions, line ending conversion (between Linux, Windows and Mac styles), regular expressions for search-and-replace, spell check etc). http://professional-guru.com http://professional-guru.com 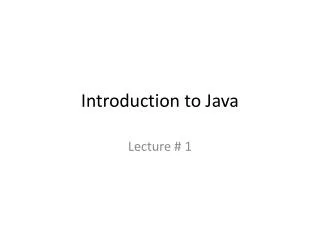 Introduction to JavaIntroduction to Java. Lecture # 1. Java History. 1991 - Green Team started by Sun Microsystems. *7 Handheld controller for multiple entertainment systems. There was a need for a programming language that would run on various devices. Java (first named Oak) was developed for this purpose. 712 views • 58 slides 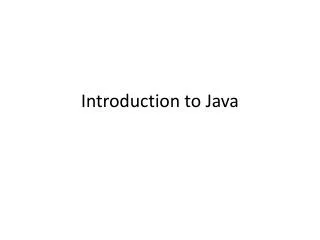 Introduction to Java. Outline. Classes Access Levels Member Initialization Inheritance and Polymorphism Interfaces Inner Classes Generics Exceptions Reflection. Access Levels. Private Protected Default Accessed by other classes in the same package 363 views • 26 slides 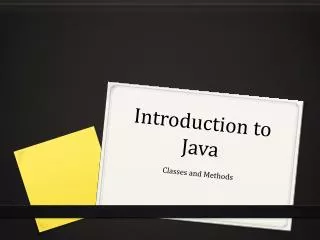 Introduction to Java. Classes and Methods. What is a Class?. A way to define new types of objects. Can be considered as a blueprint, which is a model of the object that you are describing. 462 views • 35 slides 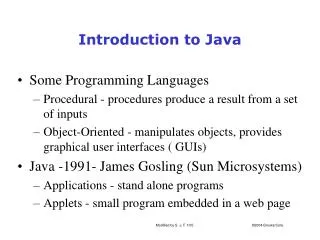 Some Programming Languages Procedural - procedures produce a result from a set of inputs Object-Oriented - manipulates objects, provides graphical user interfaces ( GUIs) Java -1991- James Gosling (Sun Microsystems) Applications - stand alone programs 909 views • 23 slides 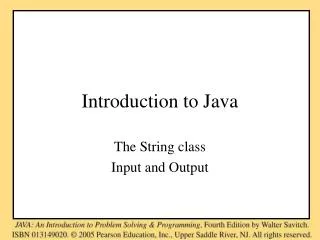 Introduction to Java. The String class Input and Output. Classes. A class is a type used to produce objects. An object is an entity that stores data and can take actions defined by methods . For example: An object of the String class stores data consisting of a sequence of characters. 273 views • 16 slides 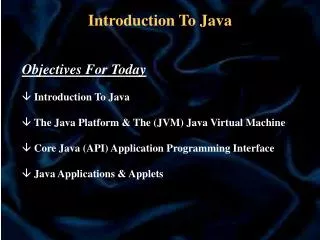 Introduction To JavaIntroduction To Java. Objectives For Today Introduction To Java The Java Platform & The (JVM) Java Virtual Machine Core Java (API) Application Programming Interface Java Applications & Applets. What Is Java ?. 598 views • 20 slides 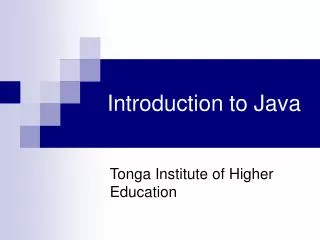 Introduction to Java. Tonga Institute of Higher Education. Programming Basics. Program – A set of instructions that a computer uses to do something. Programming / Develop – The act of creating or changing a program Programmer / Developer – A person who makes a program 348 views • 23 slides 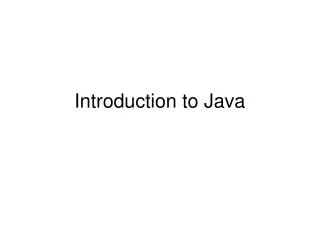 Introduction to Java. A Brief History of OOP and Java. Early high level languages _________________________ More recent languages BASIC, Pascal, C, Ada, Smalltalk, C++, Java ______________ operating system upgrades prompted the development of the C language 711 views • 53 slides 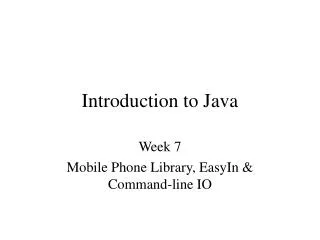 Introduction to Java. Week 7 Mobile Phone Library, EasyIn & Command-line IO. Case Study on Mobiles. Mobile phone library New directory/workspace, eg mobile Class definition file MobileNumber.java Demonstration file MobileNumberDemonstration.java 215 views • 11 slides 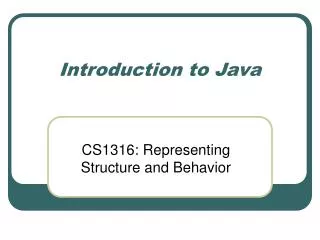 Introduction to Java. CS1316: Representing Structure and Behavior. Story. Getting started with Java Installing it Using DrJava Basics of Java It’s about objects and classes, modeling and simulation, what things know and what they can do. Examples from Pictures, Sounds, and music. 401 views • 28 slides 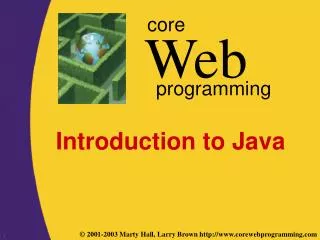 Introduction to Java. Agenda. Unique Features of Java Java versions Installation and running Java programs Basic Hello World application Command line arguments Basic Hello WWW applet. Java is Web-Enabled and Network Savvy. Safety in Java programs can be enforced 499 views • 32 slides 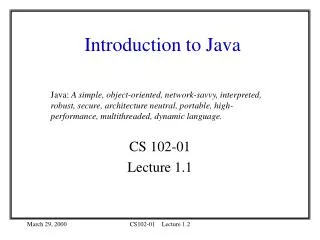 Introduction to Java. Java: A simple, object-oriented, network-savvy, interpreted, robust, secure, architecture neutral, portable, high-performance, multithreaded, dynamic language. CS 102-01 Lecture 1.1. What is Java?. 481 views • 29 slides 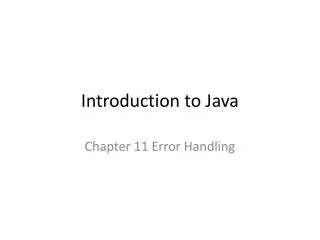 Introduction to Java. Chapter 11 Error Handling. Motivations. When a program runs into a runtime error, the program terminates abnormally. How can you handle the runtime error so that the program can continue to run or terminate gracefully?. Errors to Consider. User input errors 520 views • 40 slides 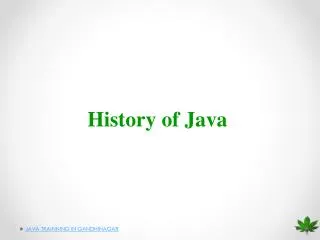 introduction to javawe are providing professional training in gandhinagar. .net training in gandhinagar php training in gandhinagar java training in gandhinagar ios training in gandhinagar android training in gandhinagar 254 views • 12 slides 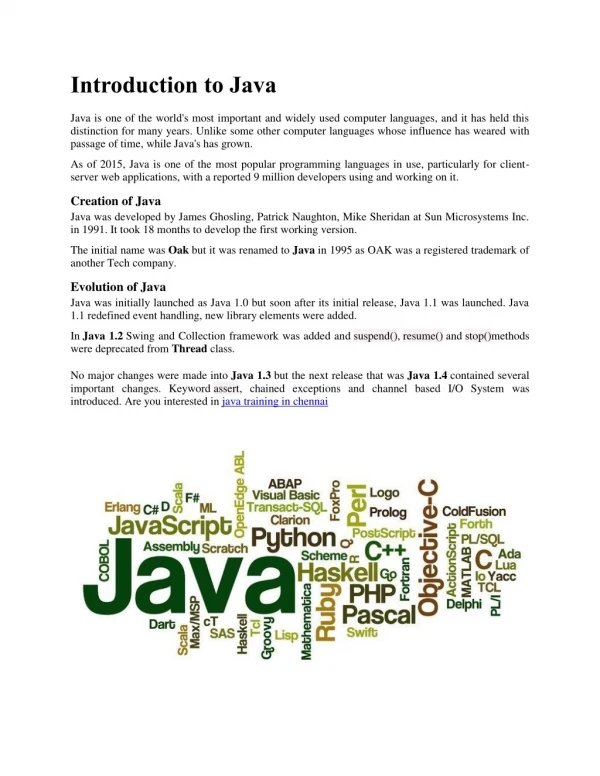 Introduction to javaJava is one of the world's most important and widely used computer languages, and it has held this distinction for many years. Unlike some other computer languages whose influence has weared with passage of time, while Java's has grown. As of 2015, Java is one of the most popular programming languages in use, particularly for client-server web applications, with a reported 9 million developers using and working on it. 268 views • 3 slides 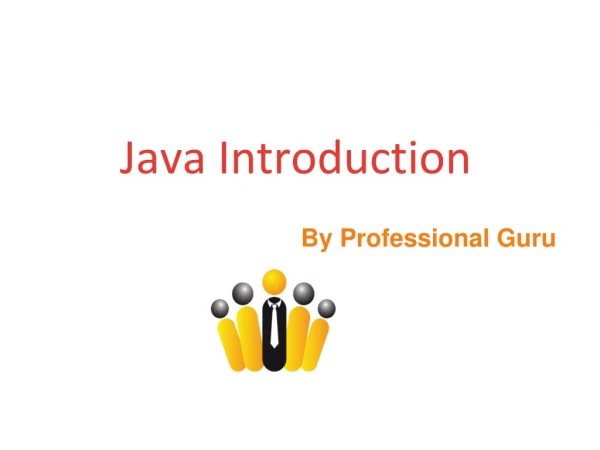 249 views • 20 slides 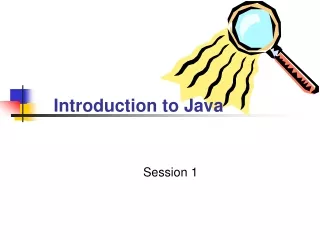 Introduction to Java. Session 1. Objectives. Discuss the brief history of Java. Discuss Java in brief Explore the types of Java programs Discuss what we can do with Java? Examine the differences between Applets and Applications Describe the Java Virtual Machine (JVM) 429 views • 28 slides 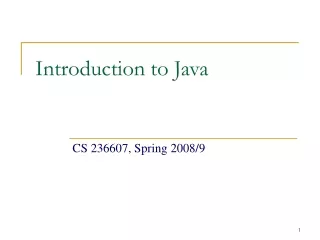 Introduction to Java. CS 236607, Spring 2008/9. הבהרה. בשני השיעורים הקרובים נעבור על יסודות שפת Java . מטרתם היא הקניית כלים שישמשו אתכם ברכישת השפה. מילים המסומנות כך מהוות קישור שיפתח בפניכם עולם ומלואו... כמעט על כל פרק ניתן להרחיב הרבה מעבר למופיע במצגת... 647 views • 61 slides 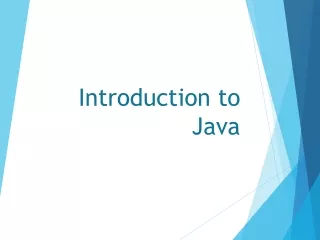 Introduction to Java. Java. Examples in textbook include line numbers. Feature which may be turned on or off in Eclipse. Source code Right click to bring up list Select Preferences Click on link to Text Editors Check mark on Line Numbers Click OK. Objects. An object has structure 325 views • 25 slides 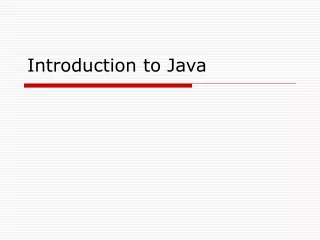 Introduction to Java. Introduction. POP Vs. OOP Programming Why Java? Java Applications; stand-alone Java programs Java applets, which run within browsers e.g. Netscape. Procedural vs. Object-Oriented Programming. 343 views • 21 slides Creating a MS PowerPoint Presentation in JavaLast updated: January 8, 2024  It's finally here: >> The Road to Membership and Baeldung Pro . Going into ads, no-ads reading , and bit about how Baeldung works if you're curious :) Azure Container Apps is a fully managed serverless container service that enables you to build and deploy modern, cloud-native Java applications and microservices at scale. It offers a simplified developer experience while providing the flexibility and portability of containers. Of course, Azure Container Apps has really solid support for our ecosystem, from a number of build options, managed Java components, native metrics, dynamic logger, and quite a bit more. To learn more about Java features on Azure Container Apps, visit the documentation page . You can also ask questions and leave feedback on the Azure Container Apps GitHub page . Java applications have a notoriously slow startup and a long warmup time. The CRaC (Coordinated Restore at Checkpoint) project from OpenJDK can help improve these issues by creating a checkpoint with an application's peak performance and restoring an instance of the JVM to that point. To take full advantage of this feature, BellSoft provides containers that are highly optimized for Java applications. These package Alpaquita Linux (a full-featured OS optimized for Java and cloud environment) and Liberica JDK (an open-source Java runtime based on OpenJDK). These ready-to-use images allow us to easily integrate CRaC in a Spring Boot application: Improve Java application performance with CRaC support Modern software architecture is often broken. Slow delivery leads to missed opportunities, innovation is stalled due to architectural complexities, and engineering resources are exceedingly expensive. Orkes is the leading workflow orchestration platform built to enable teams to transform the way they develop, connect, and deploy applications, microservices, AI agents, and more. With Orkes Conductor managed through Orkes Cloud, developers can focus on building mission critical applications without worrying about infrastructure maintenance to meet goals and, simply put, taking new products live faster and reducing total cost of ownership. Try a 14-Day Free Trial of Orkes Conductor today. To learn more about Java features on Azure Container Apps, you can get started over on the documentation page . And, you can also ask questions and leave feedback on the Azure Container Apps GitHub page . Whether you're just starting out or have years of experience, Spring Boot is obviously a great choice for building a web application. Jmix builds on this highly powerful and mature Boot stack, allowing devs to build and deliver full-stack web applications without having to code the frontend. Quite flexibly as well, from simple web GUI CRUD applications to complex enterprise solutions. Concretely, The Jmix Platform includes a framework built on top of Spring Boot, JPA, and Vaadin , and comes with Jmix Studio, an IntelliJ IDEA plugin equipped with a suite of developer productivity tools. The platform comes with interconnected out-of-the-box add-ons for report generation, BPM, maps, instant web app generation from a DB, and quite a bit more: >> Become an efficient full-stack developer with Jmix DbSchema is a super-flexible database designer, which can take you from designing the DB with your team all the way to safely deploying the schema . The way it does all of that is by using a design model , a database-independent image of the schema, which can be shared in a team using GIT and compared or deployed on to any database. And, of course, it can be heavily visual, allowing you to interact with the database using diagrams, visually compose queries, explore the data, generate random data, import data or build HTML5 database reports. >> Take a look at DBSchema Get non-trivial analysis (and trivial, too!) suggested right inside your IDE or Git platform so you can code smart, create more value, and stay confident when you push. Get CodiumAI for free and become part of a community of over 280,000 developers who are already experiencing improved and quicker coding. Write code that works the way you meant it to: >> CodiumAI. Meaningful Code Tests for Busy Devs The AI Assistant to boost Boost your productivity writing unit tests - Machinet AI . AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. And, the AI Chat crafts code and fixes errors with ease, like a helpful sidekick. Simplify Your Coding Journey with Machinet AI : >> Install Machinet AI in your IntelliJ Since its introduction in Java 8, the Stream API has become a staple of Java development. The basic operations like iterating, filtering, mapping sequences of elements are deceptively simple to use. But these can also be overused and fall into some common pitfalls. To get a better understanding on how Streams work and how to combine them with other language features, check out our guide to Java Streams: Download the E-book Do JSON right with Jackson Get the most out of the Apache HTTP Client Get Started with Apache Maven: Working on getting your persistence layer right with Spring? Explore the eBook Building a REST API with Spring? Get started with Spring and Spring Boot, through the Learn Spring course: Explore Spring Boot 3 and Spring 6 in-depth through building a full REST API with the framework: >> The New “REST With Spring Boot” Get started with Spring and Spring Boot, through the reference Learn Spring course: >> LEARN SPRING Yes, Spring Security can be complex, from the more advanced functionality within the Core to the deep OAuth support in the framework. I built the security material as two full courses - Core and OAuth , to get practical with these more complex scenarios. We explore when and how to use each feature and code through it on the backing project . You can explore the course here: >> Learn Spring Security Spring Data JPA is a great way to handle the complexity of JPA with the powerful simplicity of Spring Boot . Get started with Spring Data JPA through the guided reference course: >> CHECK OUT THE COURSE 1. IntroductionIn this article, we’ll see how we can create a presentation using Apache POI . This library gives us a possibility to create PowerPoint presentations, read existing ones, and to alter their content. 2. Maven DependenciesTo begin, we’ll need to add the following dependencies into our pom.xml : The latest version of both libraries can be downloaded from Maven Central. 3. Apache POIThe Apache POI library supports both .ppt and .pptx files , and it provides the HSLF implementation for the Powerpoint ’97(-2007) file format and the XSLF for the PowerPoint 2007 OOXML file format. Since a common interface doesn’t exist for both implementations, we have to remember to use the XMLSlideShow , XSLFSlide and XSLFTextShape classes when working with the newer .pptx file format . And, when it’s required to work with the older .ppt format, use the HSLFSlideShow , HSLFSlide and HSLFTextParagraph classes. We’ll use the new .pptx file format in our examples, and the first thing we have to do is create a new presentation, add a slide to it (maybe using a predefined layout) and save it. Once these operations are clear, we can then start working with images, text, and tables. 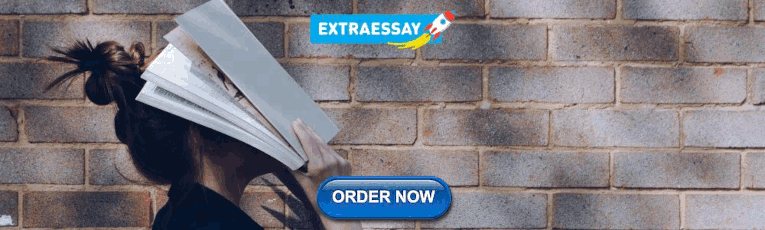 3.1. Create a New PresentationLet’s first create the new presentation: 3.2. Add a New SlideWhen adding a new slide to a presentation, we can also choose to create it from a predefined layout. To achieve this, we first have to retrieve the XSLFSlideMaster that holds layouts (the first one is the default master): Now, we can retrieve the XSLFSlideLayout and use it when creating the new slide: Let’s see how to fill placeholders inside a template: Remember that each template has its placeholders, instances of the XSLFAutoShape subclass, which could differ in number from one template to another. Let’s see how we can quickly retrieve all placeholders from a slide: 3.3. Saving a PresentationOnce we’ve created the slideshow, the next step is to save it: 4. Working With ObjectsNow that we saw how to create a new presentation, add a slide to it (using or not a predefined template) and save it, we can start adding text, images, links, and tables. Let’s start with the text. When working with text inside a presentation, as in MS PowerPoint, we have to create the text box inside a slide, add a paragraph and then add the text to the paragraph: When configuring the XSLFTextRun , it’s possible to customize its style by picking the font family and if the text should be in bold, italic or underlined. 4.2. HyperlinksWhen adding text to a presentation, sometimes it can be useful to add hyperlinks. Once we have created the XSLFTextRun object, we can now add a link: 4.3. ImagesWe can add images, as well: However, without a proper configuration, the image will be placed in the top left corner of the slide . To place it properly, we have to configure its anchor point: The XSLFPictureShape accepts a Rectangle as an anchor point, which allows us to configure the x/y coordinates with the first two parameters, and the width/height of the image with the last two. Text, inside of a presentation, is often represented in the form of a list, numbered or not. Let’s now define a list of bullet points: Similarly, we can define a numbered list: In case we’re working with multiple lists, it’s always important to define the indentLevel to achieve a proper indentation of items. 4.5. TablesTables are another key object in a presentation and are helpful when we want to display data. Let’s start by creating a table: Now, we can add a header: Once the header is completed, we can add rows and cells to our table to display data: When working with tables, it’s important to remind that it’s possible to customize the border and the background of every single cell. 5. Altering a PresentationNot always when working on a slideshow, we have to create a new one, but we have to alter an already existing one. Let’s give a look to the one that we created in the previous section and then we can start altering it: 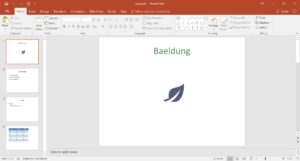 5.1. Reading a PresentationReading a presentation is pretty simple and can be done using the XMLSlideShow overloaded constructor that accepts a FileInputStream : 5.2. Changing Slide OrderWhen adding slides to our presentation, it’s a good idea to put them in the correct order to have a proper flow of slides. When this doesn’t happen, it’s possible to re-arrange the order of the slides. Let’s see how we can move the fourth slide to be the second one: 5.3. Deleting a SlideIt’s also possible to delete a slide from a presentation. Let’s see how we can delete the 4th slide: 6. ConclusionThis quick tutorial has illustrated how to use the Apache POI API to read and write PowerPoint file from a Java perspective. The complete source code for this article can be found, as always, over on GitHub . Looking for the ideal Linux distro for running modern Spring apps in the cloud? Meet Alpaquita Linux : lightweight, secure, and powerful enough to handle heavy workloads. This distro is specifically designed for running Java apps . It builds upon Alpine and features significant enhancements to excel in high-density container environments while meeting enterprise-grade security standards. Specifically, the container image size is ~30% smaller than standard options, and it consumes up to 30% less RAM: >> Try Alpaquita Containers now. Explore the secure, reliable, and high-performance Test Execution Cloud built for scale. Right in your IDE: Basically, write code that works the way you meant it to. AI is all the rage these days, but for very good reason. The highly practical coding companion, you'll get the power of AI-assisted coding and automated unit test generation . Machinet's Unit Test AI Agent utilizes your own project context to create meaningful unit tests that intelligently aligns with the behavior of the code. Get started with Spring Boot and with core Spring, through the Learn Spring course: 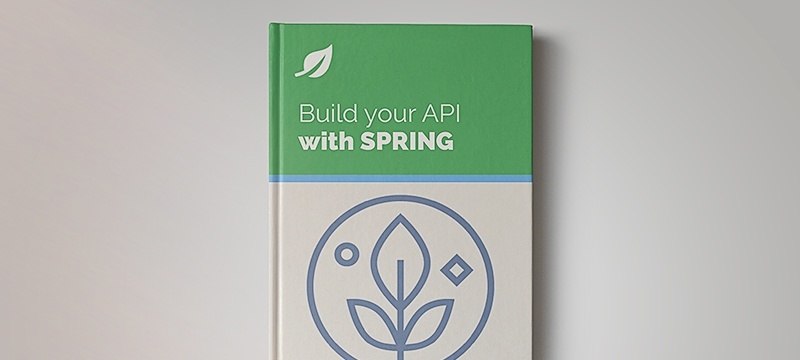 Follow the Java Category 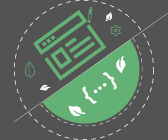  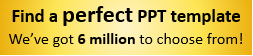 Full Stack Java Development - PowerPoint PPT Presentation Full Stack Java DevelopmentFull stack java development means that you have to develop product or application from end to end from scratch to live deployment. 100% job placement assistance is offered after the completion of the course. – powerpoint ppt presentation. - To Learn the core concepts of both the frontend and backend programming course
- To Get familiar with the latest web development technologies
- To Learn all about sql and nosql databases To Learn complete web development process
PowerShow.com is a leading presentation sharing website. It has millions of presentations already uploaded and available with 1,000s more being uploaded by its users every day. Whatever your area of interest, here you’ll be able to find and view presentations you’ll love and possibly download. And, best of all, it is completely free and easy to use. You might even have a presentation you’d like to share with others. If so, just upload it to PowerShow.com. We’ll convert it to an HTML5 slideshow that includes all the media types you’ve already added: audio, video, music, pictures, animations and transition effects. Then you can share it with your target audience as well as PowerShow.com’s millions of monthly visitors. And, again, it’s all free. About the Developers PowerShow.com is brought to you by CrystalGraphics , the award-winning developer and market-leading publisher of rich-media enhancement products for presentations. Our product offerings include millions of PowerPoint templates, diagrams, animated 3D characters and more.   DEV Community Posted on Dec 14, 2018 • Updated on Dec 17, 2018 Create PowerPoint Presentations in JavaIn this article, we will show you how to create PowerPoint presentations from scratch using a free Java PowerPoint API – Free Spire.Presentation for Java. Table of ContentsOverview of free spire.presentation for java, create a “hello world” presentation. - Format Content in Presentation
Add Images to PresentationAdd bullet list to presentation, create table in presentation, create chart in presentation, set document properties to presentation, protect presentation with password. Free Spire.Presentation for Java is a free Java PowerPoint API, by which developers can create, read, modify, write, convert and save PowerPoint document in Java applications without installing Microsoft Office. For more information of Free Spire.Presentation for Java, check here . Download Free Spire.Presentation jars: https://www.e-iceblue.com/Download/presentation-for-java-free.html The following example shows how to create a simple presentation with “Hello World” text. 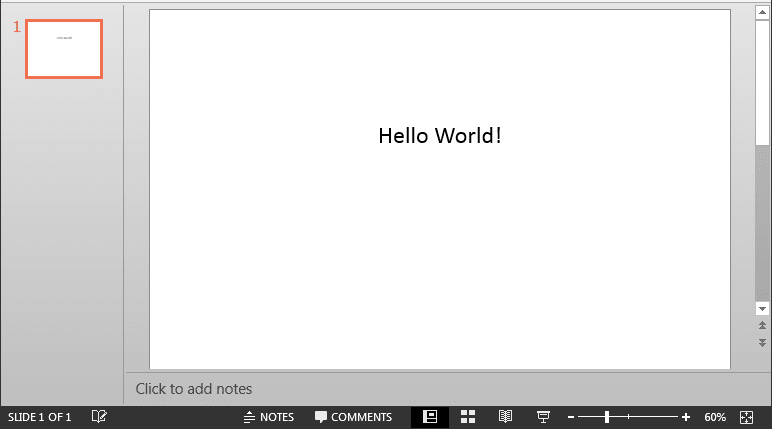 Format Text Content in PresentationThe following example shows how to format text content in a presentation. 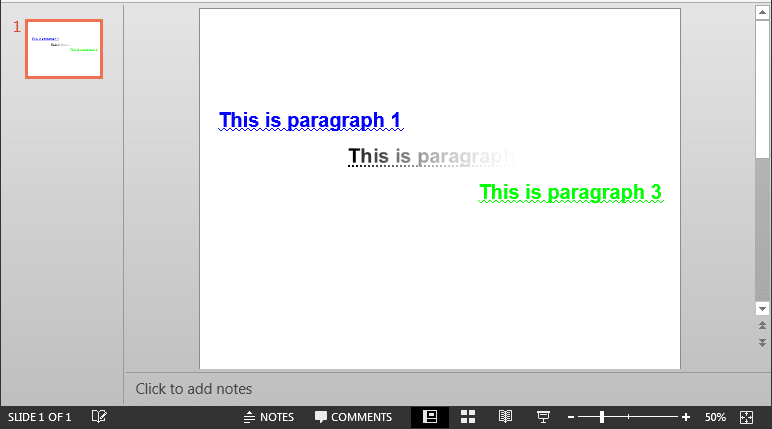 The following example shows how to add images to a presentation. 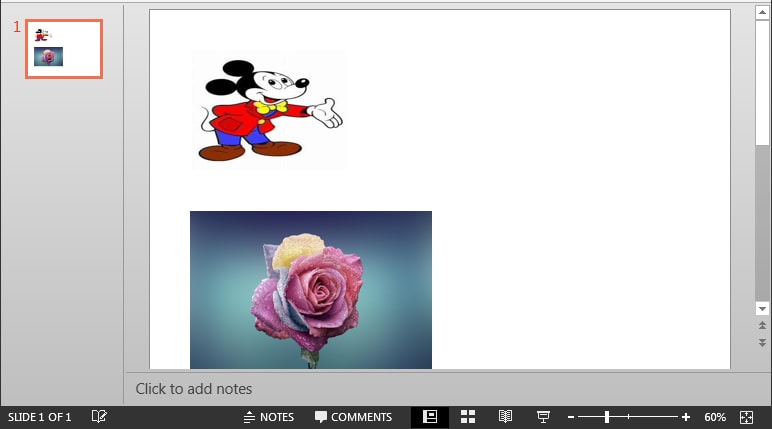 The following example shows how to add bullet list to presentation. 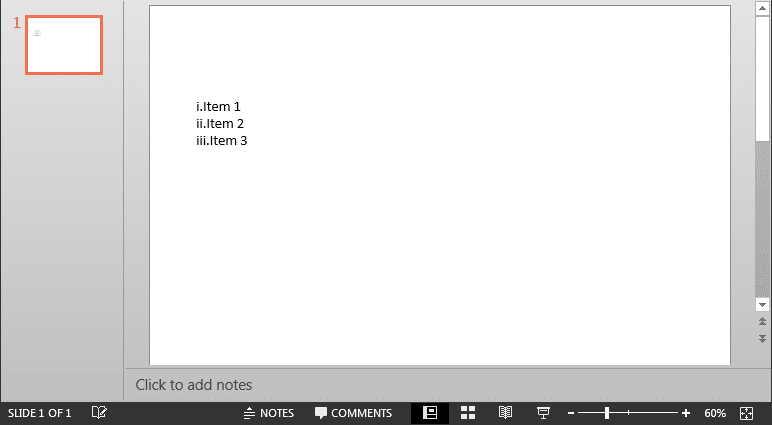 The following example shows how to create table in presentation. 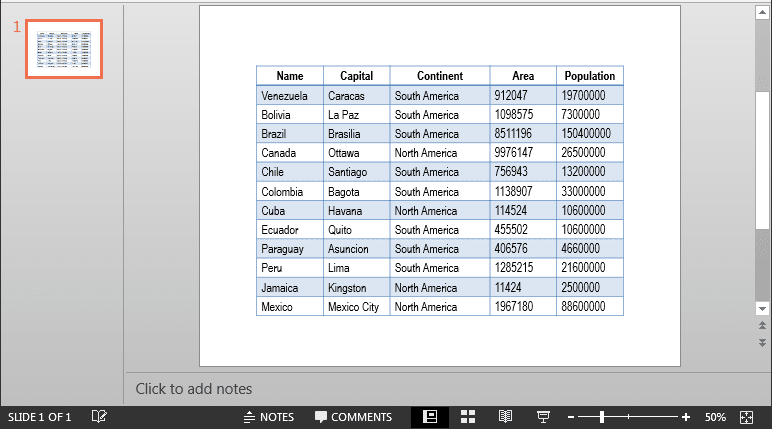 Free Spire.Presentation for Java supports a variety types of charts. Here we choose bubble chart as an example.  The following example shows how to set document properties, such as author, company, key words, comments, category, title and subject, to a presentation. 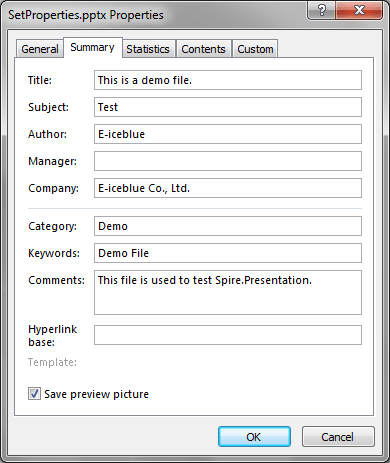 The following example shows how to protect a presentation with password. 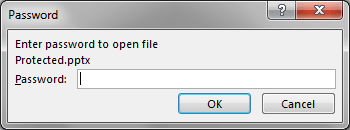 Top comments (0)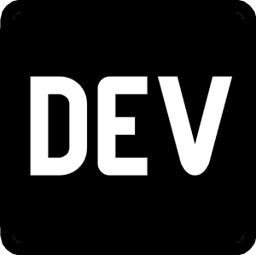 Templates let you quickly answer FAQs or store snippets for re-use. Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink . Hide child comments as well For further actions, you may consider blocking this person and/or reporting abuse  𝗠𝗶𝗰𝗿𝗼𝘀𝗲𝗿𝘃𝗶𝗰𝗲𝘀 𝗶𝗻 𝗝𝗮𝘃𝗮: 𝗔𝗿𝗰𝗵𝗶𝘁𝗲𝗰𝘁𝘂𝗿𝗲, 𝗕𝗲𝗻𝗲𝗳𝗶𝘁𝘀, 𝗮𝗻𝗱 𝗜𝗺𝗽𝗹𝗲𝗺𝗲𝗻𝘁𝗮𝘁𝗶𝗼𝗻Ricardo Maia - Aug 19  Aplicando o Single Responsability Principle com Typescript e JavaVictor Lima Reboredo - Aug 18  Java - BeginnerBellamer - Aug 16  How to Build a PWA with Java and Spring Boot: Best Practices for Offline Functionality?Nathan Lively - Aug 13 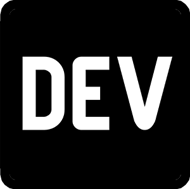 We're a place where coders share, stay up-to-date and grow their careers. - Java Course
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
How to Create a MS PowerPoint Presentation in Java with a Maven Project?In the software industry, presentations play a major role as information can be conveyed easily in a presentable way via presentations. Using Java, with the help of Apache POI, we can create elegant presentations. Let us see in this article how to do that. Necessary dependencies for using Apache POI: It has support for both .ppt and .pptx files. i.e. via - HSLF implementation is used for the Powerpoint 97(-2007) file format
- XSLF implementation for the PowerPoint 2007 OOXML file format.
There is no common interface available for both implementations. Hence for - .pptx formats, XMLSlideShow, XSLFSlide, and XSLFTextShape classes need to be used.
- .ppt formats, HSLFSlideShow, HSLFSlide, and HSLFTextParagraph classes need to be used.
Let us see the example of creating with .pptx format Creation of a new presentation: Next is adding a slide Now, we can retrieve the XSLFSlideLayout and it has to be used while creating the new slide Let us cover the whole concept by going through a sample maven project. Example Maven ProjectProject Structure: As this is the maven project, let us see the necessary dependencies via pom.xml PowerPointHelper.java In this file below operations are seen - A new presentation is created
- New slides are added
- save the presentation as
We can write Text, create hyperlinks, and add images. And also the creation of a list, and table are all possible. In general, we can create a full-fledged presentation easily as well can alter the presentation by adjusting the slides, deleting the slides, etc. Below code is self-explanatory and also added comments to get an understanding of it also. We can able to get the presentation got created according to the code written and its contents are shown in the image below We can test the same by means of the below test file as well PowerPointIntegrationTest.java Output of JUnit: We have seen the ways of creating of presentation, adding text, images, lists, etc to the presentation as well as altering the presentation as well. Apache POI API is a very useful and essential API that has to be used in software industries for working with the presentation. 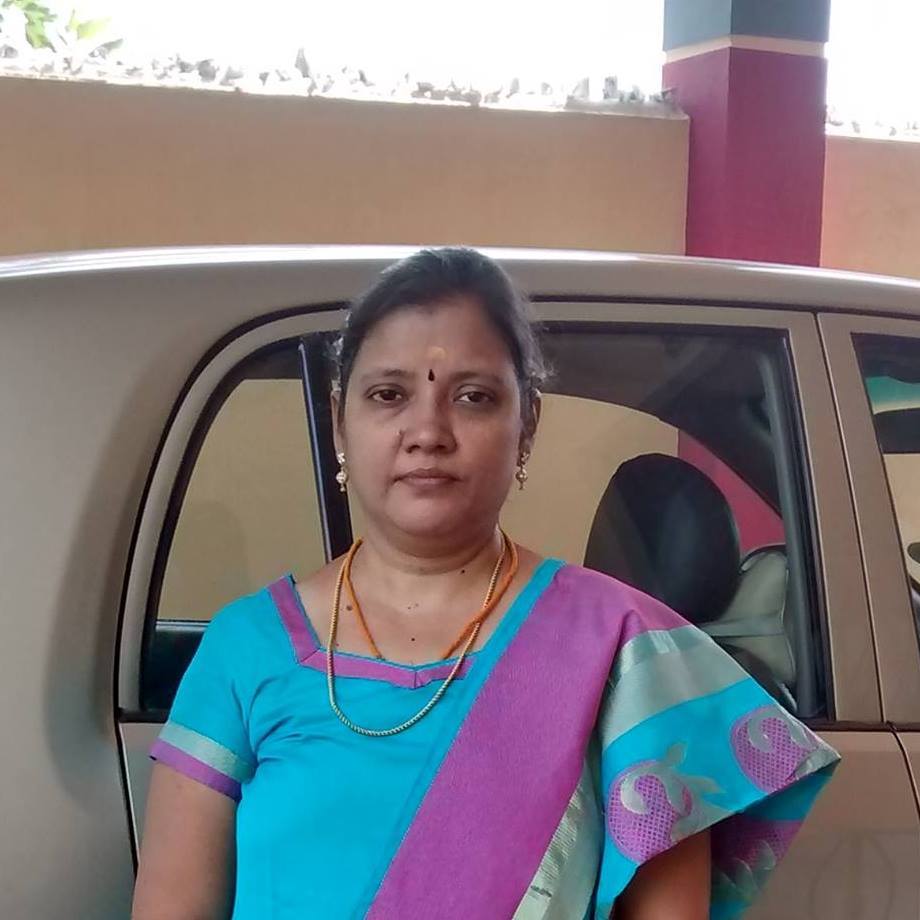 Please Login to comment...Similar reads. - Technical Scripter
- Technical Scripter 2022
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- What is OpenAI SearchGPT? How it works and How to Get it?
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice What kind of Experience do you want to share?- Create a presentation Article
- Save Article
- Design Article
- Share and collaborate Article
- Give a presentation Article
- Set up your mobile apps Article
- Learn more Article
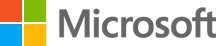 Create a presentation Create a presentation in PowerPoint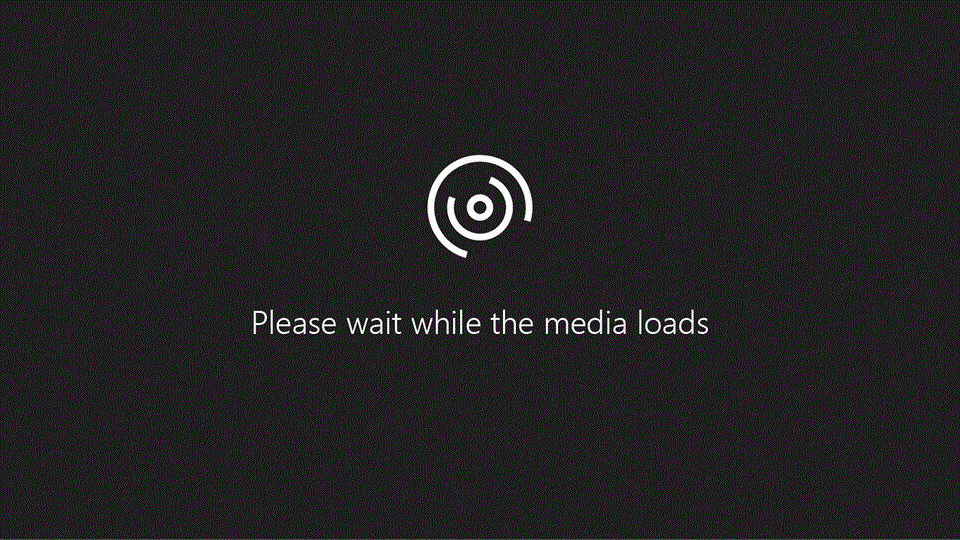 Create presentations from scratch or start with a professionally designed, fully customizable template from Microsoft Create . Tip: If you have Microsoft Copilot it can help you create a presentation, add slides or images, and more. To learn more see Create a new presentation with Copilot in PowerPoint. Open PowerPoint. In the left pane, select New . Select an option: To create a presentation from scratch, select Blank Presentation . To use a prepared design, select one of the templates. To see tips for using PowerPoint, select Take a Tour , and then select Create , .  Add a slide In the thumbnails on the left pane, select the slide you want your new slide to follow. In the Home tab, in the Slides section, select New Slide . In the Slides section, select Layout , and then select the layout you want from the menu. 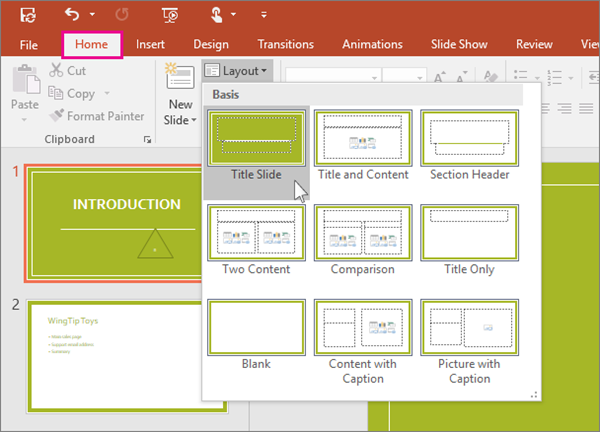 Add and format text Place the cursor inside a text box, and then type something. Select the text, and then select one or more options from the Font section of the Home tab, such as Font , Increase Font Size , Decrease Font Size , Bold , Italic , Underline , etc. To create bulleted or numbered lists, select the text, and then select Bullets or Numbering . 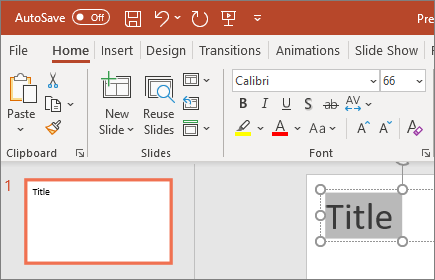 Add a picture, shape, and more Go to the Insert tab. To add a picture: In the Images section, select Pictures . In the Insert Picture From menu, select the source you want. Browse for the picture you want, select it, and then select Insert . To add illustrations: In the Illustrations section, select Shapes , Icons , 3D Models , SmartArt , or Chart . In the dialog box that opens when you click one of the illustration types, select the item you want and follow the prompts to insert it. 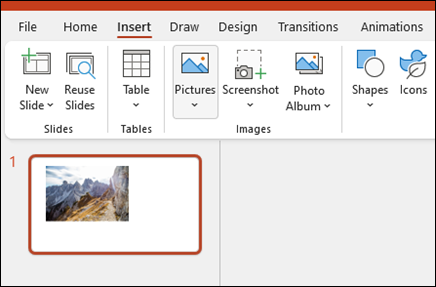 Need more help?Want more options. Explore subscription benefits, browse training courses, learn how to secure your device, and more. 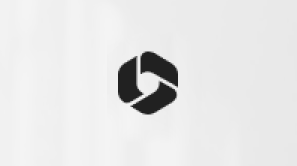 Microsoft 365 subscription benefits 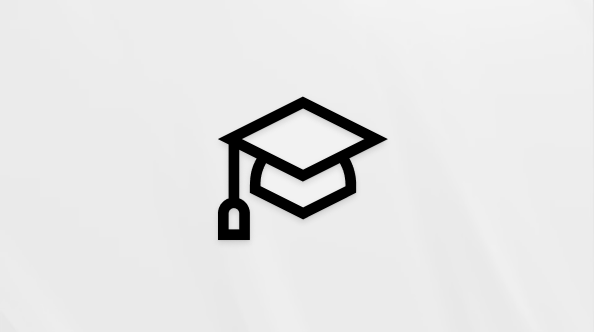 Microsoft 365 training 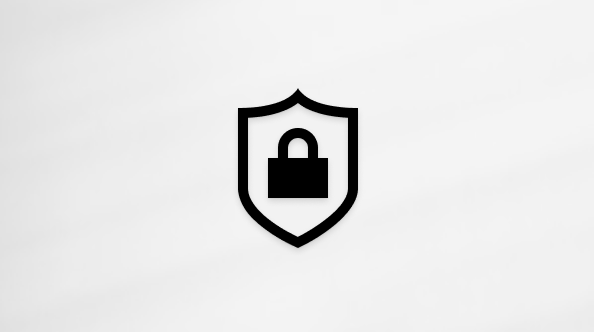 Microsoft security 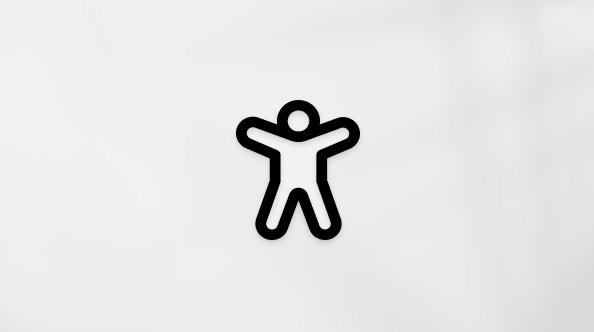 Accessibility center Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.  Ask the Microsoft Community 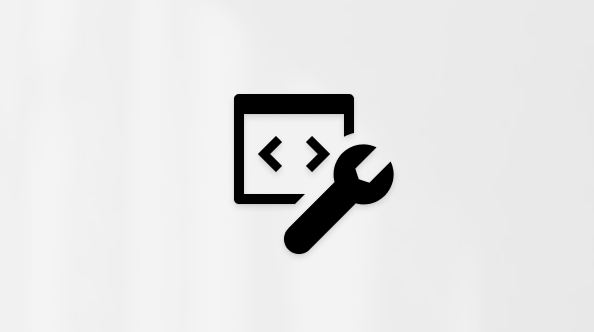 Microsoft Tech Community 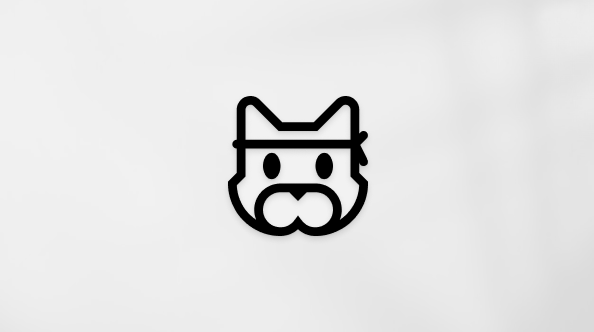 Windows Insiders Microsoft 365 Insiders Find solutions to common problems or get help from a support agent.  Online support Was this information helpful?Thank you for your feedback. 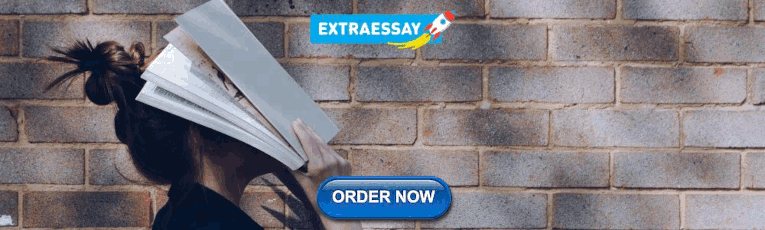 |
IMAGES
COMMENTS
Chapter 01: Introduction to Java. To understand the relationship between Java and World Wide Web. To know Java's advantages. To distinguish the terms API, IDE and JDK. To write a simple Java program. To create, compile and run Java programs. To know the basic syntax of a Java program.
Lecture presentation on programming in Java. Topics include: object oriented programming, defining classes, using classes, constructors, methods, accessing fields, primitives versus references, references versus values, and static types and methods.
Activity : Create and Execute a Java project Writing a simple HelloWorld program in Java using Eclipse. Step l: Start Eclipse. Step 2: Create a new workspace called sample and 01. Project Eun Step 5: Select "Java" in the categ Java EE - Eclipse Eile Edit Navigate Search ti ct • Select "Java Project" in the project list.
Presentation transcript: 1 Chapt. 1 Introduction to Core Java. 2 Lecture 1: Overview of Java. 3 Introduction Java is a programming language invented by James Gosling and others in 1994. originally named Oak,was developed as a part of the Green project at the Sun Company. A general-purpose object-oriented language Based on C/C++.
Download ppt "Introduction to Java Programming". Focus of the Course Object-Oriented Software Development problem solving program design, implementation, and testing object-oriented concepts classes objects encapsulation inheritance polymorphism.
Java is high-level programming language originally developed by Sun Microsystems and released in 1995. Java runs on a variety of platforms, such as Windows, Mac OS/ and the various versions of UNIX. According to SUN, 3 billi n devices run java. There are many devices where java is currently used. Some of them are as follows: Presentation by Abk ...
Ch03.ppt ~ Chapter 3. Java Syntax and Style Ch04.ppt ~ Chapter 4. Objects and Classes Ch05.ppt ~ Chapter 5. Data Types, Variables, and Arithmetic Ch06.ppt ~ Chapter 6. Boolean Expressions and if-else Statements Ch07.ppt ~ Chapter 7. Algorithms and Iterations Ch08.ppt ~ Chapter 8. Strings
Contains easy-to-edit graphics such as graphs, maps, tables, timelines and mockups. Includes 500+ icons and Flaticon's extension for customizing your slides. Designed to be used in Google Slides, Canva, and Microsoft PowerPoint. 16:9 widescreen format suitable for all types of screens. Includes information about fonts, colors, and credits of ...
Free Google Slides theme, PowerPoint template, and Canva presentation template. Hey teachers, get your students coding in no time with our sleek, minimal black PowerPoint and Google Slides template tailored for high school Java programming lessons. ... This easy-to-use, engaging slideshow template is perfect for breaking down the basics of Java ...
CORE JAVA Fundamentals of OOP What is OOP Difference between Procedural and Object oriented programming Basic OOP concept - Object, classes, abstraction,encapsulation, inheritance, polymorphism 1 Fundamentals of OOP Introduction to JAVA Introductin to JAVA 2 History of Java Java ... Sales pitch presentation: creating impact with Prezi; July 22 ...
Introduction to JAVA. JAVA was developed by Sun Microsystems Inc in 1991, later acquired by Oracle Corporation. It was developed by James Gosling and Patrick Naughton. It is a simple programming language. Writing, compiling and debugging a program is easy in java. It helps to create modular programs and reusable code.
What is Java? Presentation On Introduction To Core Java By PSK Technologies (1) - Learn JAVA tutorial -This Java tutorial is specially prepared for the Beginners who wants to learn Java programming language from the basics. This tutorial is prepared by Easy Web Solutions by PSK Technologies located in Nagpur that provides best training in Java,PHP,Web Development Hardware and Networking and ...
Unlock a Vast Repository of Java And J2EE PPT Slides, Meticulously Curated by Our Expert Tutors and Institutes. Download Free and Enhance Your Learning! ... PowerPoint Presentation Slides. Search our vast collection of PowerPoint presentations. Choose Subject .Net; 2D Animation; 3D Printing; Abacus / Brain Gym; Accountancy;
When working with text inside a presentation, as in MS PowerPoint, we have to create the text box inside a slide, add a paragraph and then add the text to the paragraph: XSLFTextBox shape = slide.createTextBox(); XSLFTextParagraph p = shape.addNewTextParagraph(); XSLFTextRun r = p.addNewTextRun(); r.setText( "Baeldung" );
Description: Full stack java development means that you have to develop product or application from end to end from scratch to live deployment. 100% job placement assistance is offered after the completion of the course. - PowerPoint PPT presentation. Number of Views: 285. Slides: 9. Provided by: Maddy333. Category: How To, Education & Training.
Overview of Free Spire.Presentation for Java Free Spire.Presentation for Java is a free Java PowerPoint API, by which developers can create, read, modify, write, convert and save PowerPoint document in Java applications without installing Microsoft Office. For more information of Free Spire.Presentation for Java, check here.
A new presentation is created. New slides are added. save the presentation as. FileOutputStream outputStream = new FileOutputStream(fileLocation); samplePPT.write(outputStream); outputStream.close(); We can write Text, create hyperlinks, and add images. And also the creation of a list, and table are all possible.
create and deliver PowerPoint presentations , (SOPs): 5 years (Required) tracking APD project actual expenditures against CMS: 7 years (Required) Work Location: Remote. Show more If you require alternative methods of application or screening, you must approach the employer directly to request this as Indeed is not responsible for the ...
Create a presentation. Open PowerPoint. In the left pane, select New. Select an option: To create a presentation from scratch, select Blank Presentation. To use a prepared design, select one of the templates. To see tips for using PowerPoint, select Take a Tour, and then select Create, .